Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial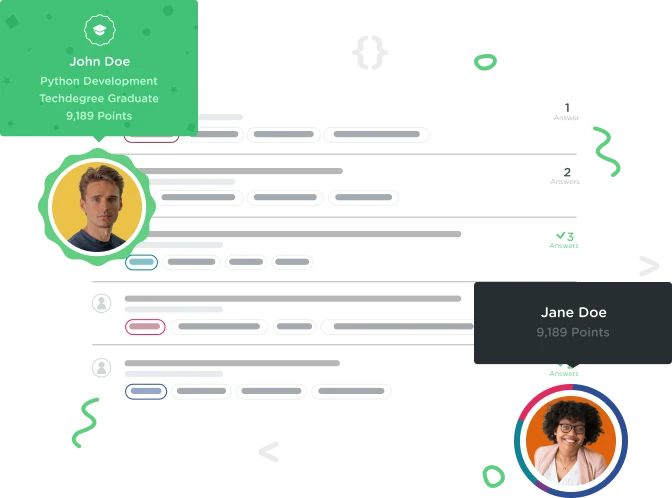
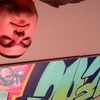
Andrew Neary
Courses Plus Student 939 PointsSimple array problem I am stuck on
this is the challenge
Declare an array of type 'char' called "letters" with a size of 2. Assign the variable "alpha" as the first element of the array "letters".
I am writing this
char letters[2]; letters [0] = alpha;
or
char letters[] = {alpha}
Am I missing code or doing something wrong
10 Answers

novall
3,397 PointsHi Andrew! Here's the correct syntax for this question:
char alpha = 'a';
char letters[2];
letters[0] = alpha;

novall
3,397 Points@Nick -- I like the succinct way in which your array was declared, but both ways work. For the purposes of this problem, I simply broke it down into steps, first initializing the array, and then later adding to the array. See here for further explanations: http://www.thegeekstuff.com/2011/12/c-arrays/
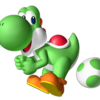
Ninjutsu NoOb to ExpeRt!
Courses Plus Student 129 PointsOMG SO MANY WRONG ANSWERS!

novall
3,397 Pointslol :) What do you propose are the right answers?
The great thing about Treehouse discussions vs. say, Stack Overflow, is that it's "ok" to be wrong -- we're all learning here! :)
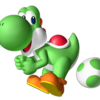
Ninjutsu NoOb to ExpeRt!
Courses Plus Student 129 PointsLOL the answer i'm seeking is the right answer, and usually that answer is the right answer! well i've just spent a great deal of time just researching array... i'm still clueless! =P

novall
3,397 PointsAh, I see. Here's a brief explanation of arrays -- hope this helps!
So arrays are just data structures (i.e. they hold data). They're great for storing data in a particular order, which enables you to pull a given object from the list at any given location. This location, or position in the array, is also known as an index.
To help you picture what's going on, say we have a box called box. This box contains 5 objects. Here's what our box array looks like upon defining it:
char box[5];
The char indicates that the type of items inside the box are all one-letter characters. Arrays should always contain elements of the same type.
Now say we want to initialize the array, to actually stuff the box with our lettered objects. Here's what we'd do:
char box[] = {'a','b','c','d','e'};
Now we have a box with 5 letters. Just because the box has 5 elements, though, that does not mean that the highest index is 5. When we want to pull an item from the box, or reference an item, we have to start counting from 0. So, our letter a is at index 0, our letter b is at index 1, and our letter e is at index 4.
So, the 5th letter in our box can also be placed in our box like so:
box[4]={'e'};
Let me know if you have any more questions -- I'm happy to help :) Here's another tutorial I found which I thought had a good explanation of accessing arrays as well, which is a lot more complicated in C than it is in other languages: http://www.tutorialspoint.com/cprogramming/c_arrays.htm
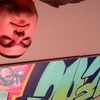
Andrew Neary
Courses Plus Student 939 Pointscorrection char letters[2]; letters[0] = alpha;
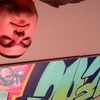
Andrew Neary
Courses Plus Student 939 PointsThanks Novall

Nick Blomquist
3,659 Pointsthat is wrong here is the answer
char letters[2] = {alpha};
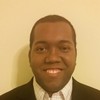
Frankie Lamar
11,120 Points@Nick Blomquist - How can your answer be correct. The array has a zero index so char letters[2] would be pointing to the third item in the array. The instructions say to add the var alpha to the first location in the array. That would be letters[0].

novall
3,397 PointsHey Frankie! Nick's answer is actually ok, because when you're defining an array, the value you insert in the brackets [ ] is the number of elements the array stores (which in this case, starts at 1). Otherwise, you're right -- letters[2] would indicate the third item in the array when accessing that item. See this link for further clarification: http://www.thegeekstuff.com/2011/12/c-arrays/

novall
3,397 PointsAh, I see. Here's a brief explanation of arrays -- hope this helps!
So arrays are just data structures (i.e. they hold data). They're great for storing data in a particular order, which enables you to pull a given object from the list at any given location. This location, or position in the array, is also known as the index.
To help you picture what's going on, say we have a box called box. This box contains 5 objects. Here's what our box array looks like upon defining it:
char box[5];
The char indicates that the type of items inside the box are all one-letter characters. Arrays should always contain elements of the same type.
Now say we want to initialize the array, to actually stuff the box with our lettered objects. Here's what we'd do:
char box[] = {'a','b','c','d','e'};
Now we have a box with 5 letters. Just because the box has 5 elements, though, that does not mean that the highest index is 5. When we want to pull an item from the box, or reference an item, we have to start counting from 0. So, our letter a is at index 0, our letter b is at index 1, and our letter e is at index 4.
So, the 5th letter in our box can also be placed in our box like so:
box[4]={'e'};
Let me know if you have any more questions -- I'm happy to help :) Here's another tutorial I found which I thought had a good explanation of accessing arrays as well, which is a lot more complicated in C than it is in other languages: http://www.tutorialspoint.com/cprogramming/c_arrays.htm
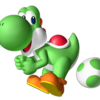
Ninjutsu NoOb to ExpeRt!
Courses Plus Student 129 PointsThanks Navall you've been a great help!!! Soon i'll be an expert like you! =D

novall
3,397 PointsAny time! Glad I could help! :)
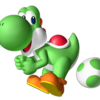
Ninjutsu NoOb to ExpeRt!
Courses Plus Student 129 Points824 points my gawd!!! ur good.... since november 2013 wow

wei kang ng
139 Points- Declare 2 separate variables of type char named "alpha" and "bravo". Assign the letter 'a' to the variable "alpha" and the letter 'b' to "bravo".
char alpha = 'a'; char bravo = 'b';
- Declare an array of type 'char' called "letters" with a size of 2. Assign the variable "alpha" as the first element of the array "letters".
char alpha = 'a'; char bravo = 'b'; char letters[2] = {alpha};
- Assign the variable "bravo" as the second element of the array "letters".
char alpha = 'a'; char bravo = 'b'; char letters [2] = {alpha, bravo};
- Print the results. Your output should look like "letters a b".
char alpha = 'a'; char bravo = 'b'; char letters [2] = {alpha, bravo}; printf("letters %c %c \n", letters [0], letters [1]);

Nick Blomquist
3,659 Pointshere is the full version from step 1 and 2
char alpha = 'a'; char bravo = 'b';
char letters[2] = {alpha};