Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial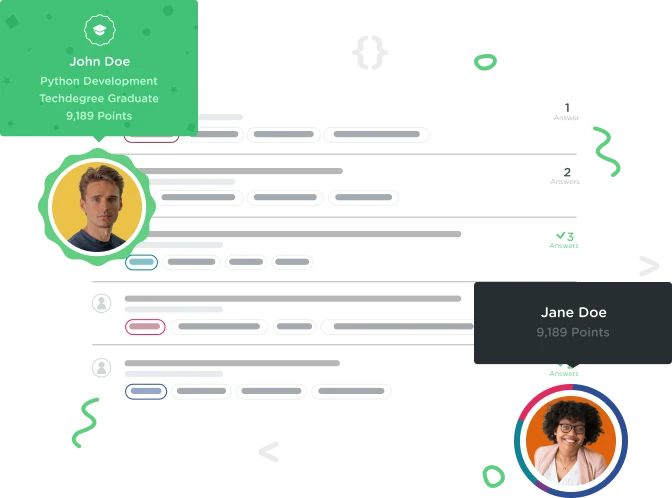
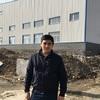
ali mur
UX Design Techdegree Student 23,377 Pointssimple calculator with html, css, js
I have created a simple calculator, and I am trying to add items, and I want to print the results on the console. The problem is when I press the button with id "addbtn" it does not add the items. It seems that something working in the background but, when I check the console I just see nothing.
<html>
<head>
</head>
<link rel="stylesheet" href="style.css">
<body>
<div id="container">
Number 1:<input type="text" id="input1" /><br>
Number 2:<input type="text" id="input2" />
<div id="operations">
<button id="addbtn">+</button>
<button id="subtractbtn">-</button>
<button id="multiplybtn">*</button>
<button id="divide"btn>/</button>
</div>
Result: <input type="text" id="result"/>
</div>
<script src="app.js"></script>
</body>
</html>```
```css
#container {
border:2px solid #3b5998;
background-color: #d3d3d3;
margin:auto;
padding:5px;
height:20%;
width:20%;
}
#operations{
margin:20px;
}```
JAVASCRIPT
```javascript
var num1=document.getElementById('input1').value;
var num2=document.getElementById('input2').value;
var addvar=document.getElementById('addbtn');
var subtractvar=document.getElementById('subtractbtn');
var multiplyvar=document.getElementById('multiplybtn');
var dividevar=document.getElementById('dividebtn');
addvar.addEventListener("click", function add(){console.log(num1 + num2)});```
2 Answers
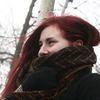
Ella Ruokokoski
20,881 PointsHi! You are getting the values for the input buttons before they are being assigned with any values, That's why your code doesn't work. Instead, you have to get the values from inside your click function
var num1=document.getElementById('input1'); // removed the .value from the variable declarations
var num2=document.getElementById('input2');
var result = document.getElementById("result");//created variable for result input
addvar.addEventListener("click", function(){
var firstNum = parseInt(num1.value); //get the values from num1 and num2 inputs and turn the string into a number with parseInt() so the calculation will work
var secondNum = parseInt(num2.value);
result.value = firstNum + secondNum; //print the result into result input
console.log( firstNum+ secondNum);
});
also I noticed you have a typo in your html where you set an id to your divide button
<button id="divide"btn>/</button>
also, since your inputs should be only numbers you should change your input type to "number" for your inputs. This way writing letters to the inputs isn't possible. If you want to hide the spin-button arrows of the number input you can add this to your css
input::-webkit-outer-spin-button,
input::-webkit-inner-spin-button {
-webkit-appearance: none;
margin: 0;
}
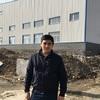
ali mur
UX Design Techdegree Student 23,377 PointsHi, this works. thx