Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial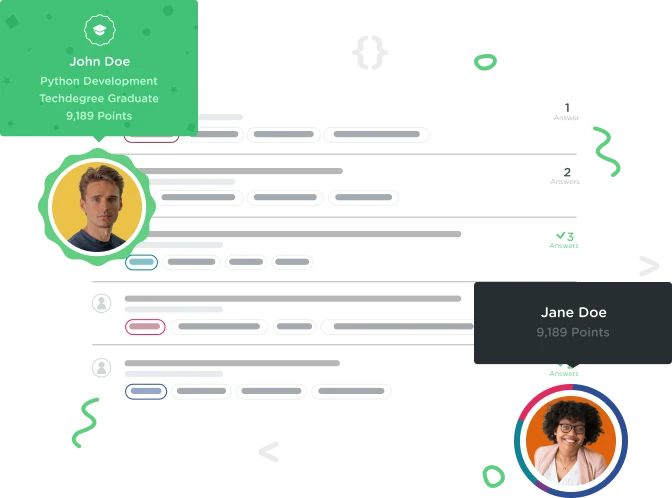
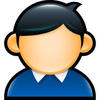
Daniel Hildreth
16,170 PointsSimple Drop Down Menu Help
So I'm having a slight issue with my code in Treehouse. I went back to the Simple Drop Down Menu video in jQuery Basics course, because I wanted to download the workspace for reference material if I ever needed it. But my code seems to match up, but something is obviously wrong with it. The menu and such still appears in the bigger version windows. Here's the css and js coding.
CSS
* {
margin: 0;
padding: 0;
font-family: sans-serif;
}
body {
background: #fff;
}
#menu {
background: #384047;
height: 60px;
padding: 0 5px 0;
text-align: center;
}
ul {
list-style: none;
}
ul li {
display: inline-block;
width: 84px;
text-align: center;
}
ul li a {
color: #fff;
width: 100%;
line-height: 60px;
text-decoration: none;
}
ul li.selected {
background: #fff;
}
ul li.selected a {
color: #384047;
}
select {
width: 94%;
margin: 11px 0 11px 2%;
float: left;
}
h1 {
margin: 40px 0 10px;
text-align: center;
color: #384047;
}
p {
text-align: center;
color: #777;
}
/** Start Coding Here **/
/**
Modify CSS to hide links on small width and show button and select
Also hides select and button on larger width and show's links
**/
@media (min-width: 320px) and (max-width: 568px) {
#menu ul {
display:none;
}
}
@media (min-width: 568px) {
#menu select, #menu button {
display:none;
}
}
JS (jQuery)
//Problem: It looks gross in smaller browser widths and small devices
//Solution: To hide the text links and swap them out with a more appropriate navigation
//Create a select and apend to menu
var $select = $("<select></select>");
$("#menu").append($select);
//Cycle over menu links
$("#menu a").each(function(){
var $anchor = $(this);
//Create an option
var $option = $("<option></option>");
//Deal with selected options depending on current page
if($anchor.parent().hasClass("selected")) {
$option.prop("selected", true);
}
//option's value is the href
$option.val($anchor.attr("href"));
//option's text is the text of link
$option.text($anchor.text());
//append option to select
$select.append($option);
});
//Bind change listener to the select
$select.change(function(){
//Go to select's location
window.location = $select.val();
});
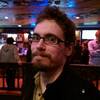
Chris Andruszko
18,392 PointsThe code here is solid. It would help to see your HTML because I'm guessing there might be parts with incorrect elements or IDs that may not match the CSS.
4 Answers
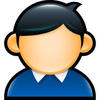
Daniel Hildreth
16,170 PointsYes sorry meant to clarify that Marcus. Yes I still see the select drop down box on larger views, and the text in the middle where it says like "About" in on the right side of the screen and not in the middle.
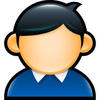
Daniel Hildreth
16,170 PointsChris you asked for the HTML code for this as well, so here it is:
HTML
<!DOCTYPE html>
<html>
<head>
<link rel="stylesheet" href="css/style.css" type="text/css" media="screen" title="no title" charset="utf-8">
</head>
<body>
<div id="menu">
<ul>
<li class="selected"><a href="index.html">Home</a></li>
<li><a href="about.html">About</a></li>
<li><a href="contact.html">Contact</a></li>
<li><a href="support.html">Support</a></li>
<li><a href="faqs.html">FAQs</a></li>
<li><a href="events.html">Events</a></li>
</ul>
</div>
<h1>Home</h1>
<p>This is the home page.</p>
<script src="http://code.jquery.com/jquery-1.11.0.min.js" type="text/javascript" charset="utf-8"></script>
<script src="js/app.js" type="text/javascript" charset="utf-8"></script>
</body>
</html>
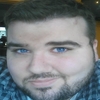
Marcus Parsons
15,719 PointsWhat browser are you running your tests on? I am not seeing any problem with your code on either Chrome or Firefox.
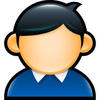
Daniel Hildreth
16,170 PointsIm using chrome
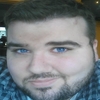
Marcus Parsons
15,719 PointsHmmm, I used the exact code you posted here to make a test page, and everything is working as expected on my end. That's strange. Your browser may be trying to use a cached version of your site. Try clearing your internet history including your cache and then refresh the page and resize.
Marcus Parsons
15,719 PointsMarcus Parsons
15,719 PointsDaniel,
What do you mean by "the menu and such still appears in the bigger version windows"? Do you mean that the select box is showing at a larger resolution?