Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial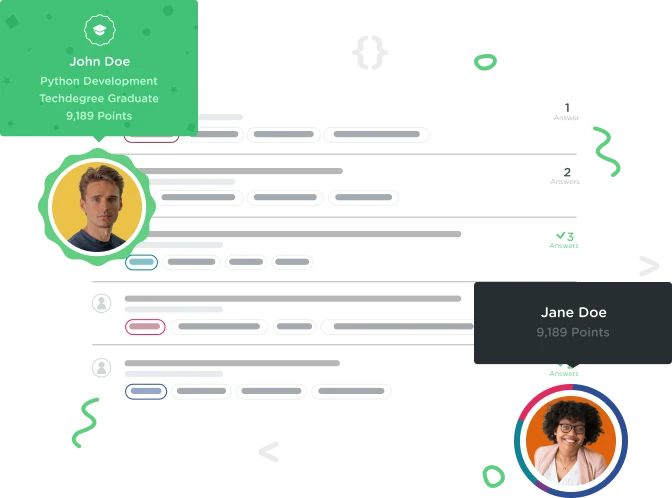
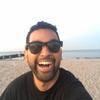
Anthony Albertorio
22,624 PointsSimple example to see functions, methods, methods with variable arguments
package main
import "fmt"
type Hours int
// function
func Increment(h Hours) Hours {
return (h + 1) % 24
}
// method with no arguments (pass by value)
func (h Hours) IncrementMethod() {
return (h + 1) % 24 // return value aka return copy of original
}
// method with pointer no arguments (pass by reference)
func (h *Hours) IncrementMethod() {
*h = (*h + 1) % 24 // update value aka mutate orignal in memory
}
// method with single argument
func (h *Hours) IncrementByX(x Hours) {
*h = (*h + x) % 24
}
// method with variable arguments
func (h *Hours) IncrementBySeveral(x ...Hours) {
sum := Hours(0)
for i := range x { // come in array, so iterate
sum += x[i]
}
*h = (*h + sum) % 24
}
func main() {
// as function
hours1 := Hours(23)
hours1 = Increment(hours1)
fmt.Println(hours1) // Prints "0".
// as method
hours2 := Hours(23)
hours2.IncrementMethod()
fmt.Println(hours2) // Prints "0"
// as method with parameter
hours3 := Hours(23)
hours3.IncrementByX(5)
fmt.Println(hours3) // Prints "4"
// as method with variable amount of arguments
hours4 := Hours(23)
hours4.IncrementBySeveral(3, 5)
fmt.Println(hours4) // Prints "7"
}
Hope this helps