Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial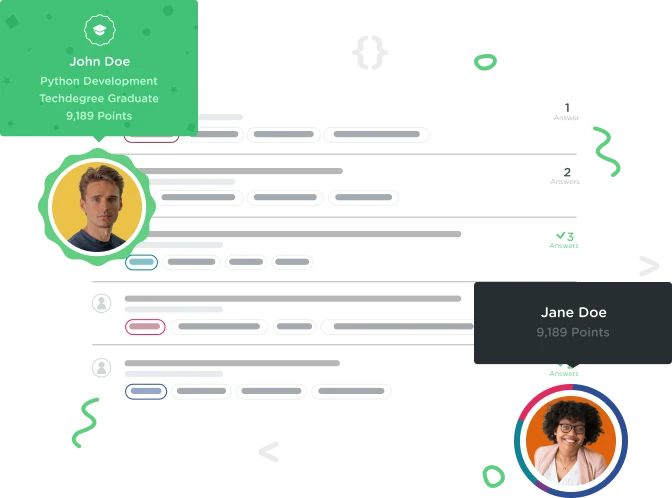
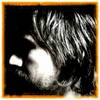
Justin Bigos
3,828 PointsSimple solution
My example
var lowNum = parseInt( prompt('Please enter a number 1 to 10'));
var highNum = parseInt( prompt('Please enter a 100 to 200'));
var randomNum = Math.floor(Math.random() * highNum) + lowNum;
document.write(randomNum);
2 Answers
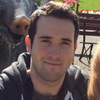
Justin Iezzi
18,199 PointsHi Justin,
There are a few issues with your solution. I assume you're looking for a random number between lowNum and highNum?
First, lets talk about the + 1 correction we can do. This line: Math.floor(Math.random() * highNum)
will generate a number between 0 and highNum, and of course it will be rounded down because of Math.floor. So for example, if I input the number 5 into the highNum prompt, this line will calculate a number between 0 or 4, because Math.random() starts at 0, and 0 registers as a number. If this doesn't make sense, think of it like this: there are five numbers here - 0 1 2 3 4. In order to return a number between 1 - 5, all you need to do is add a + 1 to highNum. This increment shifts the potential numbers into 1 2 3 4 5.
Math.floor(Math.random() * highNum) + 1
Now this will return a number between 1 and 5, instead of 0 and 4. This will give the user a more accurate number.
But that isn't the only thing wrong here, there is still a pretty big logical error. Lets take a look. I'm going to take a step back and remove the + 1 from these examples.
var randomNum = Math.floor(Math.random() * highNum) + lowNum;
There are two numbers being added together here.
- The result of
Math.floor(Math.random() * highNum)
- lowNum
Lets assume that the user inputs 5 for the low number, and 10 for the high number. High number is multiplied with the .random() method. Since this calculation can result in a number between 1 and 10, lets say we got 8. Now 8 is added to lowNum, which is 5. So the user thought they were going to get a number between 5 and 10, but instead received the number 13.
We can now see that the result isn't truly a number between 5 and 10, but a number between 1 and 10 plus 5.
Now, it's a bit confusing, but to properly get a random number between two numbers, we need to write our calculation like this:
var randomNum = Math.floor(Math.random() * (highNum - lowNum) + 1 ) + lowNum;
Just like the + 1 fix (which I've included now), this will bump up the starting value to what the user specified for lowNum, as well as maintain the correct highNum number cap.
Say that lowNum = 20 and highNum = 55. Lets walk through how the calculation works.
Math.floor(Math.random() * (55 - 20) + 1 ) + 20;
55 minus 20 equals 35.
Math.floor(Math.random() * (35) + 1 ) + 20;
Now lets assume that Math.random() * (35) returned the number 13.
var randomNum = (13 + 1) + 20;
Now the +1 fix is added, so that we don't potentially get a number lower than what we specified, or never get the highest number we specified.
var randomNum = 14 + 20;
var randomNum = 34;
The result will always be a number between 20 and 55. By subtracting (highNum - lowNum), we get the amount of numbers between lowNum and highNum. The +1 is to make sure we don't get a 0 and that reaching 55 is possible. The final + lowNum essentially sets where our bracket started and ended, giving us a number we expected between 20 and 55.
It's funny that I've ran across this because I just finished these few lessons, and I was a little disappointing that the way of doing this was so complicated. So if you still don't understand how Math.floor(Math.random() * (highNum - lowNum) + 1 ) + lowNum;
works, it's okay. You can always refer back to this base calculation and you'll get your desired effect.
I also have to mention that this is a bit less intuitive than other languages default random methods. Many high level languages that I've come across hide this calculation within the method, so you would just have to specify the starting and ending numbers. If desired, you could easily make a function that would make the process a bit cleaner, you'll learn those later.
And speaking of things you'll be learning, the next course will teach you how to force the user to input a number between 1 and 10, 100 and 200. You could revisit this challenge and implement that sort of feature for practice.
Long winded, I know, but I hope that I've helped some here. If you have any questions please feel free to ask.
Justin
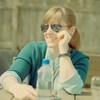
Jessica Evans
7,372 PointsThank you, this was very helpful.
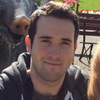
Justin Iezzi
18,199 PointsGlad it was helpful!

Clara Roldan
3,074 PointsHelpful explanation, thanks

Sophia He
2,554 PointsThanks for the answer, it was very informative!
I just not quite sure if I understand why do we need to add Lowernumber. You see, if my number is 1 and 100, and random number is 0.2, my result will still in between 1 and 100, because Math.floor(Math.random() * (highNum - lowNum) + 1 ) has gauranteed that the number will not be bigger than the highNum. Please help me understand it.
Samuel Webb
25,370 PointsSamuel Webb
25,370 PointsI added some formatting for your code to make it easier to read. Go to the Markdown Cheatsheet for more information on how to do what I did.