Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial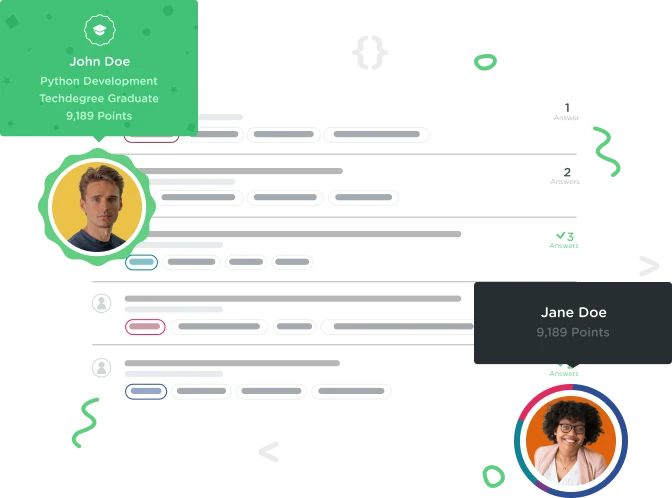

Enzo Cnop
5,157 PointsSimple way to ask python to check if a number is/is not/ a float.
For the code challenge after creating a number game, I was asked to create a function that would return True if the number entered is even, and False, if the number entered is odd. The code that let me pass the challenge is below.
def even_odd(number):
if number % 2 == 0 :
return True
else:
return False
My question: Why can't I write this code? (please ignore indentation, i'm not sure how to format code on this website yet.)
def even_odd(number):
if number % 2 == float :
return False
else:
return True
Surely, python recognizes what a 'float' is, so why can't it check whether or not a number is a float this way? I don't even understand how the code that let me pass the challenge works. Say 5 is the number entered. 6 % 2 = 3, by my understanding of this logic, the code should return false, because the resulting integer is not equal to 0. How does this work. Please help me understand.
2 Answers
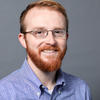
Kurt Maurer
16,725 PointsHey Enzo,
Many languages work solely with integer values unless specified, so 5 / 3 = 1 (1.67, but it returns the integer value). In this case, you could probably get away with it, since Python will actually return a float when performing functions with integers. You could use:
isinstance(number, float)
But in the case of even/odd, the modulus operator (%) returns the remainder, so it is not returning the value like a division operator. The example you cited above, 6 / 3 = 2, but 6 % 3 = 0, since three divides completely into 6. If you did 6 % 5, you would get 1, as there is a remainder of 1 when six is divided by 5. In this challenge, any even number divided by 2 will equal 0; thus, it is even. If it isn't 0, then the number is odd. I hope this helps!

Selemawit Gebremedhin
1,331 Pointsnum = int (input ("Enter number: "))
def is_odd (num):
if num % 2 != 0:
return True
else:
return False
I don't know why it is giving me error