Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial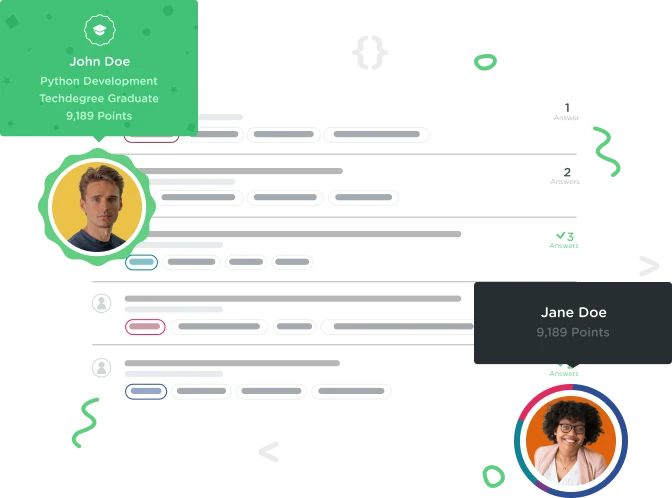
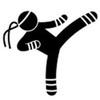
fabianfroding
18,491 PointsSimplified If-statement same as == or ===?
When using simplified if-statement ex: if (isEmailEmpty()) {}, is it the same as isEmailEmpty() == true, or isEmailEmpty() === true?
Thanks
/Fabian
2 Answers

andren
28,558 PointsThe answer is neither. As can be demonstrated by the following code:
console.log("Non-empty string test")
function test() {
return "Non empty strings are loosely considered to be true"
}
if (test()) {
console.log("TRUE")
} else {
console.log("FALSE")
}
if (test() == true) {
console.log("TRUE")
} else {
console.log("FALSE")
}
if (test() === true) {
console.log("TRUE")
} else {
console.log("FALSE")
}
The test will result in the first if statement running, but not the two other ones. In other words the "simplified if-statement" is actually looser than either of the other comparisons, in that it accepts "truthy" values like non-empty strings as true. Which the double equals comparison does not.
Also I made a post earlier that I have since deleted, if you can still read it then please disregard its contents, I realize after posting it that I had made a mistake while testing the code and come to the wrong conclusion.
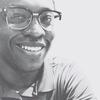
Ricardo Hill-Henry
38,442 PointsJudging by the function name, you wouldn't need to use either. If a function returns a boolean value, you can simply place it in an if statement without an explicit comparison.
if (isEmailEmpty()) {
//Do something
}
If statements only continue if the value within the parenthesis equals true. If your function (or variable) equals a boolean, then its a bit redundant to check if it's true or false using the comparison operators.
If you wanted to see if it was false, then you could simply put an exclamation mark in front of the value. The exclamation mark is the "not" operator, which converts a value to it's opposite.
if (!isEmailEmpty()) { //if isEmailEmpty() returns true then ! will make it false; if returns false then ! makes it true
//Do something
}
In this specific case, it wouldn't matter if you used == or === since both values are of the same type. However, === is often used to avoid type coercion. When using == your types can be implicitly converted as an attempt to check to see if they're equal in some way at all. The strict comparison (===) doesn't allow type coercion (unless explicitly written).
let a = true,
b = 1,
c = "23",
d = 23;
a == b; //true - the value 1 can be coerced to equal true because of convention
a === b; //false - this compares a boolean and a number 1; which do not equal
c == d; //true - the values are coerced to equal each other; either 23 == 23 or "23" == "23"
c === d; //false - the values 23 and "23" don't equal (clearly)
edit: I misunderstood your original question. It could be considered similar to loose comparison, but the real answer is neither. The if statement would simply look to see if a value exists. Any value. For example:
let a = true
let b = 2,
(a == b); //false
if(b){
"hello";
}
Although b clearly doesn't equal true, "hello" will still be written since there is some value at b. Any value besides: undefined, null, "", 0, -0, NaN, and false will be considered true if checked in that condition.