Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial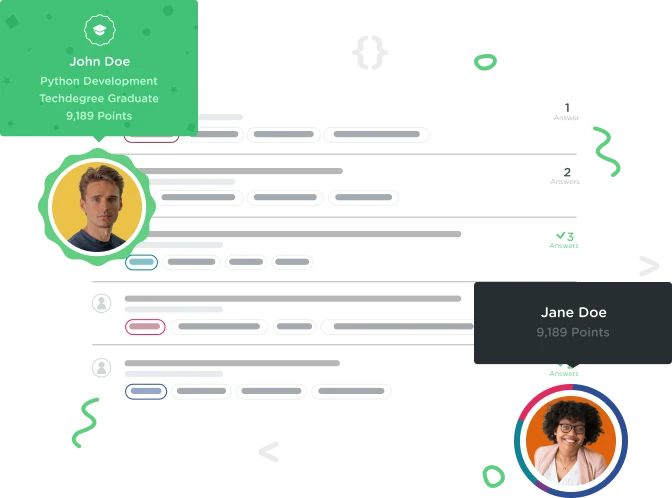
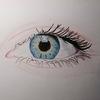
Ruby Bassi
3,873 PointsSimplifying my code
Hi all, my code works (phew!) but i was hoping someone can show me how to reduce / condense my code. P.s. excuse my random quiz questions :)
var a1 = "ELIZABETH" var a2 = "TEHRAN" var a3 = "BARCELONA" var a4 = "LONDON" var a5 = "HERCULES" score1 = 0
var q1 = prompt("What is the Queen's first name?"); if (q1.toUpperCase() === a1) { score1 += 1 ; } var q2 = prompt("What is the captial of Iran?"); if (q2.toUpperCase() === a2) { score1 += 1 ; } var q3 = prompt("What is the capital of Spain?"); if (q3.toUpperCase() === a3) { score1 += 1 ; } var q4 = prompt("Which city is the Big Ben?"); if (q4.toUpperCase() === a4) { score1 += 1 ; } var q5 = prompt("final quesiton, Who was Zeus' son?"); if (q5.toUpperCase() === a5) { score1 += 1 ; } if (score1 === 5) { document.write("That's great. You scored " + score1 + " points and earned a Gold crown!");
} else if (score1 === 4 || 3) {
document.write("Well done. You scored " + score1 + " points and earned a Silver crown!");
}
else if (score1 === 2 || 1) {
document.write("Nice! You scored " + score1 + " points and earned a Bronze crown!");
}
else if (score1 === 0) {
document.write("Bad luck! You scored " + score1 + " points.");
}
else
document.write("you scored " + score1 + " points");
2 Answers

Gryphon Chandler
12,668 PointsI managed to make it a bit shorter and much more clear by:
- Using a loop for questions and answers.
- Immediately using toUppercase().
- Using a switch to handle score.
const questions = [
prompt("What is the Queen's first name?").toUppercase(),
prompt("What is the captial of Iran?").toUppercase(),
prompt("What is the capital of Spain?").toUppercase(),
prompt("Which city is the Big Ben?").toUppercase(),
prompt("final question, Who was Zeus' son?").toUppercase();
]
const answers = [
"ELIZABETH",
"TEHRAN",
"BARCELONA",
"LONDON",
"HERCULES"
]
let score1 = 0;
for (i = 0; i < questions.length; i++) {
if (questions[i] === answers[i]) {
score1++;
}
}
switch (score1):
let feedback = "";
case 5:
feedback = "That's great. You scored 5 points and earned a Gold crown!";
break;
case 4:
case 3:
feedback = "Well done. You scored " + score1 + " points and earned a Silver crown!";
break;
case 2:
case 1:
feedback = "Nice! You scored " + score1 + " points and earned a Bronze crown!";
break;
default:
feedback = "Bad luck! You scored 0 points."
break;
document.write(feedback);

Jonathan Tuala
10,558 PointsWe haven't gotten to loops at this part of the course yet, but that looks to be great. :)
I would suggest two things: the instructor said you have to make sure you have complete conditions on either side of the operator, so
if (score1 === 4 || 3)
won't work. It should be
if (score1 === 4 || score1 === 3)
Also, you can get rid of a little bit of code at the end. Since you included all the possible outcomes for the value of score, you will never run the
else document.write("you scored " + score1 + " points");
portion and can just delete it. (Make sure you make the last score1===0
part an else statement.)
Matus Kotulak
1,921 PointsMatus Kotulak
1,921 PointsThis code is not valid at this level. We don't know what is 'const, let, for or switch' (so basically nothing). Doesn't belong here.