Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial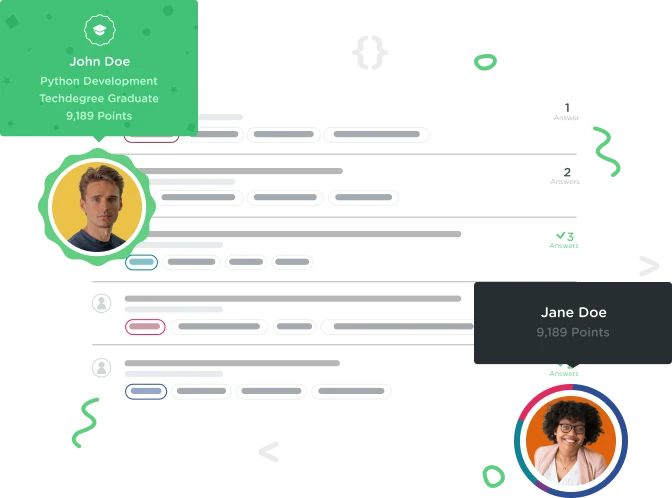

Blair Ammon
Courses Plus Student 1,130 PointsSimulator crashing when I press the fun fact button
When i press the fun fact button I get this error, Thread 1:breakpoint 1.1 It happens each time. It started after I added in the changing of the color of both the background and button text at the same time

Aaron Martin
1,855 PointsI'm getting the same error after placing in the suggested code.
''' class ViewController: UIViewController {
@IBOutlet weak var funFactLabel: UILabel!
let factBook = FactBook()
let colorWheel = ColorWheel()
override func viewDidLoad() {
super.viewDidLoad()
// Do any additional setup after loading the view, typically from a nib.
funFactLabel.text = factBook.randomFact()
}
override func didReceiveMemoryWarning() {
super.didReceiveMemoryWarning()
// Dispose of any resources that can be recreated.
}
@IBAction func showFunFact() {
view.backgroundColor = colorWheel.randomColor()
funFactLabel.text = factBook.randomFact()
}
} '''
and...
''' struct FactBook {
let factsArray = [
"Ants stretch when they wake up in the morning.",
"Ostriches can run faster than horses.",
"Olympic gold medals are actually made mostly of silver.",
"You are born with 300 bones; by the time you are an adult you will have 206.",
"It takes about 8 minutes for light from the Sun to reach Earth.",
"Some bamboo plants can grow almost a meter in just one day.",
"The state of Florida is bigger than England.",
"Some penguins can leap 2-3 meters out of the water.",
"On average, it takes 66 days to form a new habit.",
"Mammoths still walked the earth when the Great Pyramid was being built."
]
func randomFact() -> String {
var unsignedArrayCount = UInt32(factsArray.count)
var unsignedRandomNumber = arc4random_uniform(unsignedArrayCount)
var randomNumber = Int(unsignedRandomNumber)
return factsArray[randomNumber]
}
} '''
and finally...
''' struct ColorWheel {
let colorsArray = [
UIColor(red: 90/255.0, green: 187/255.0, blue: 181/255.0, alpha: 1.0), // teal color
UIColor(red: 222/255.0, green: 171/255.0, blue: 66/255.0, alpha: 1.0), // yellow color
UIColor(red: 223/255.0, green: 86/255.0, blue: 94/255.0, alpha: 1.0), // red color
UIColor(red: 239/255.0, green: 130/255.0, blue: 100/255.0, alpha: 1.0), // orange color
UIColor(red: 77/255.0, green: 75/255.0, blue: 82/255.0, alpha: 1.0), // dark color
UIColor(red: 105/255.0, green: 94/255.0, blue: 133/255.0, alpha: 1.0), // purple color
UIColor(red: 85/255.0, green: 176/255.0, blue: 112/255.0, alpha: 1.0), // green color
]
func randomColor() -> UIColor {
var unsignedArrayCount = UInt32(colorsArray.count)
var unsignedRandomNumber = arc4random_uniform(unsignedArrayCount)
var randomNumber = Int(unsignedRandomNumber)
return colorsArray[randomNumber]
}
} '''
My breakpoint is on "var unsignedArrayCount = UInt32(colorsArray.count)"
1 Answer

Aaron Martin
1,855 PointsSo, I've gone through the supplied code and what has been walked through up to this point. It appears that there is one line that is different.
In your AppDelegate.swift file, there is a line missing in the 'fun application' function. At line 19 add this:
'''application.setStatusBarStyle(UIStatusBarStyle.LightContent, animated: false)'''
Adding this in to my app allows the background to change when the button is pushed without crashing.
Can we get some clarity in to why this fixes things?
Chris Shaw
26,676 PointsChris Shaw
26,676 PointsHi Blair,
Could you please post the code surrounding your
IBOutlet
function and yourstruct
containing the facts.https://teamtreehouse.com/forum/posting-code-to-the-forum