Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial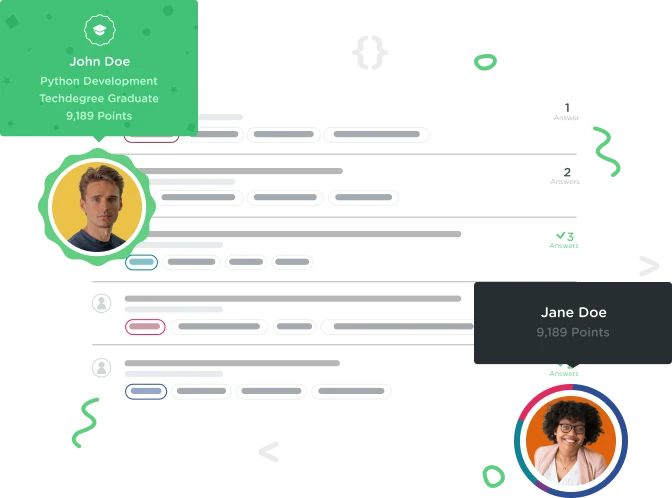

brandon saxon
520 PointsSince your square function returns a value, create a new variable named result to store the value from the function call
Since your square function returns a value, create a new variable named result to store the value from the function call.
def square(number):
value = number * number
return value
result = float(input(square))
1 Answer

Cooper Runstein
11,850 PointsThis function that you've created is meant to be interacted with by your program. I think your confusion likely comes from this fact because you put the input function in your code which is only used for interaction with a human user. You also haven't called your function, which you do by including "()" at the end of your function. This lets the computer know that you want to actually fun your function rather that just pass it around. You also never used the number 3 which the challenge wanted you to pass to the function. Notice that the challenge doesn't ask you to convert the number to a float, which makes it prone to error, but you had a really good instinct to do so even if it isn't required. Here's what the answer should look like:
def square(number):
value = number * number
return value
result = square(3);
Below is how to accomplish what I think you were trying to do in case you're interested.
def square(number):
value = number * number
return value
get_input = float(input("Pass a number"))
result = square(get_input);
If you haven't gotten there yet, soon you'll learn try/except blocks which will help protect your code from trying to multiply a string by a string as it appears you were attempting earlier.