Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial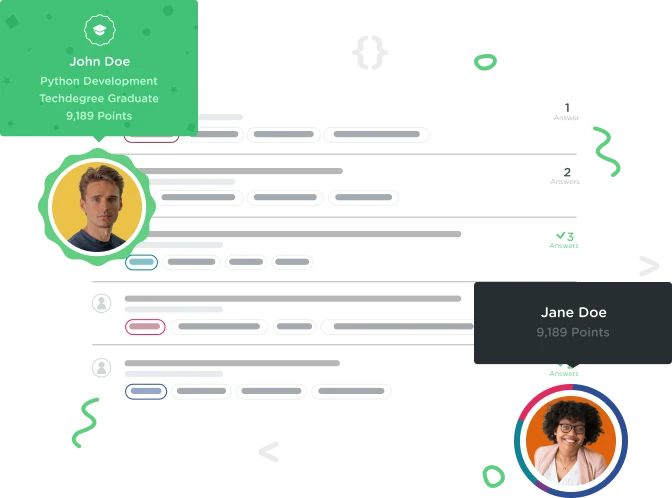

H Yang
2,066 PointsSingle vs double quotation marks
I had thought that the use of single vs double quotation marks was equivalent, but my code only runs correctly when I use double quotation marks:
function getRandomNumber( lower, upper ) {
if ( isNaN(lower) || isNaN(upper) ) {
throw new Error("Check that you didn't use two strings, you dingus");
}
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
console.log( getRandomNumber( 'nine', 24 ) );
When I try this with the single quotation marks it returns an error of "SyntaxError: missing ) after argument list"
function getRandomNumber( lower, upper ) {
if ( isNaN(lower) || isNaN(upper) ) {
throw new Error('Check that you didn't use two strings, you dingus');
}
return Math.floor(Math.random() * (upper - lower + 1)) + lower;
}
console.log( getRandomNumber( 'nine', 24 ) );
Any ideas as to what's going on? Thank you.
1 Answer

Gabriel Kartak
5,399 PointsHi Henry, normally, double quotes and single quotes are interchangeable, but in this case, the string inside of your quotes contains a single quote. If you use single quotes to surround the string, the single quote inside of it will break your code.
throw new Error('Check that you didn't use two strings, you dingus');
Here the javascript interpreter reads this as
throw new Error('Check that you didn'
- then it gets confused because the string has ended and the code after this point makes no sense if it's not in a string.
If you use
throw new Error("Check that you didn't use two strings, you dingus");
the javascript interpreter reads this as
throw new Error("Check that you didn't use two strings, you dingus");
and everything works because the single quote does not end your string early.

H Yang
2,066 PointsThank you! Great write up.

H Yang
2,066 PointsWhen you write code, do you use the single quotation more or the double? I see how using the single is nice because it precludes the necessity of hitting the shift key unnecessarily, but I foresee myself making more of these errors with unpaired quotation marks. What do you do?

Gabriel Kartak
5,399 PointsNo problem!

Gabriel Kartak
5,399 PointsI usually just use double quotes, but even then if you have to have double quotes " and single quotes ' inside of a string together you will run into a problem:
throw new Error("Check that you didn't use two "strings", you dingus");
Instead you can use a special character entity like so:
throw new Error("Check that you didn't use two "strings", you dingus");
So if you find that quotes are giving you trouble, just use the character entities inside of your strings.
H Yang
2,066 PointsH Yang
2,066 PointsI figured it out but I figured I should leave this up just in case someone else had the same question. In my error message, I have an apostrophe, which I didn't escape- it paired up with the first quotation mark, which left JavaScript with an odd number of quotation marks.