Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial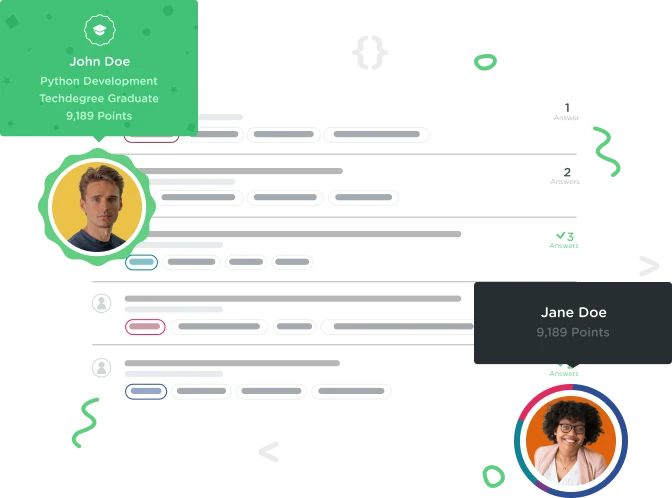

Noah Harness
1,383 Pointsslice; iterate backward to get evens in new list
def reverse_evens(iterable): iterable = list(range(10)) return iterable[::-2]
def first_4(iterable):
return iterable[0:4]
def first_and_last_4(iterable):
return iterable[:4] + iterable[-4::1]
def odds(iterable):
return iterable[1::2]
def reverse_evens(iterable):
iterable = list(range(10))
return iterable[::-2]
4 Answers
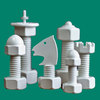
Steven Parker
230,274 PointsHere's a few hints:
- you must work with the iterable passed in, so do not assign it to something in the function!
- you might calculate which item to start with based on the length of the iterable
- another way would be to select even-indexed items with one slice, then use another slice to get the reverse
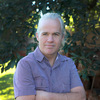
Christopher Shaw
Python Web Development Techdegree Graduate 58,248 PointsI had a different solution that did not require enumerate:
Basically takes the modulus of the length of the iterable divided by 2. This will be 0 for an even length or 1 for an odd length and added this value to -2 to make the start. So even length itrables started at -2, while odd length ones started at -1.
def reverse_evens(item):
return item[-2+len(item)%2::-2]
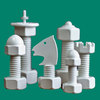
Steven Parker
230,274 PointsThat's exactly what I meant in my second hint. Good job!
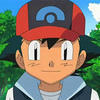
ASHOK Gudivada
11,701 Pointsgreat thank Christopher Shaw
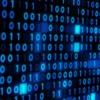
Alexander Davison
65,469 PointsThere's two important mistakes:
- You shouldn't set the value of the
iterable
parameter in the function. Like the other functions you created, you just take input and you don't change the input. - You function will work for a list with an even amount of elements, but it won't work for a list that has an odd amount of items. If the list has an odd item of items, it will return all odd indices.
To fix both issues, you can do:
- Remove the first line of code after the function definition for
reverse_evens
. This line code is disturbing the input. - Change the
return iterable[::-2]
line of code to a for loop that adds the element to a new list if the index is even.
Hint 1: You have to start with something like this...
def reverse_evens(iterable):
result_list = []
for idx, item in enumerate(iterable):
# Figure out what goes here...
return result_list
Hint 2: To see if a number is even, you can use this (where n
is the number you want to see if it's even): n % 2 == 0
The n % 2 == 0
code will return True if n
is even or if it's odd it will return False.
If you need additional hints or you don't understand what I'm saying, please post below :)
I hope this helps. ~Alex

fahad lashari
7,693 PointsHi I tried this method but it doesn't seem to be working. Can you have a look at my code and give additional clues?
def first_4(string):
return string[:4]
def first_and_last_4(string):
return string[:4] + string[-4::1]
def odds(string):
return string[1::2]
def reverse_evens(string): #problem is with function
result = []
for i, j in enumerate(string):
if i % 2 == 0:
result.append(j)
return result
EDIT:
found my stupid mistake. Thanks a bunch!
return result[::-1]

Damian Dabrowski
16,804 PointsI have used modulus to solve reverse_evens function:
def reverse_evens(item):
if len(item)%2 == 0:
return item[-2::-2]
else:
return item[::-2]
Alexander Davison
65,469 PointsAlexander Davison
65,469 PointsAnswered at the same time
Hahaha
Steven Parker
230,274 PointsSteven Parker
230,274 PointsYea, but you get the prize
for verbosity. 