Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial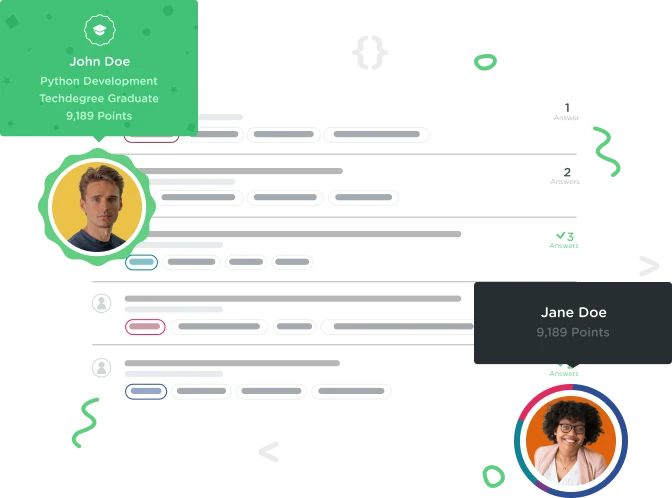
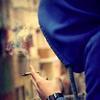
Tushar Singh
Courses Plus Student 8,692 Pointsslice() Javascript
Truncate a string(FreeCodeCamp challenge)
Truncate a string (first argument) if it is longer than the given maximum string length (second argument). Return the truncated string with a ... ending.
Note that inserting the three dots to the end will add to the string length.
However, if the given maximum string length num is less than or equal to 3, then the addition of the three dots does not add to the string length in determining the truncated string.
Remember to use Read-Search-Ask if you get stuck. Write your own code.
Here are some helpful links:
String.slice()
-------Tasks to complete------------
truncateString("A-tisket a-tasket A green and yellow basket", 11) should return "A-tisket...".
truncateString("Peter Piper picked a peck of pickled peppers", 14) should return "Peter Piper...".
truncateString("A-tisket a-tasket A green and yellow basket", "A-tisket a-tasket A green and yellow basket".length) should return "A-tisket a-tasket A green and yellow basket".
truncateString("A-tisket a-tasket A green and yellow basket", "A-tisket a-tasket A green and yellow basket".length + 2) should return "A-tisket a-tasket A green and yellow basket".
truncateString("A-", 1) should return "A...".
truncateString("Absolutely Longer", 2) should return "Ab...".
function truncateString(str, num) {
// Clear out that junk in your trunk
return str;
}
truncateString("A-tisket a-tasket A green and yellow basket", 11);
1 Answer
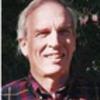
jcorum
71,830 PointsTry this:
function truncateString(str, num) {
// Clear out that junk in your trunk
if (num >= 3 && num < str.length) {
return str.slice(0, num - 3) + '...';
} else if (num >= str.length) {
return str.slice(0, num);
} else {
return str.slice(0, num) + '...';
}
}
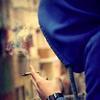
Tushar Singh
Courses Plus Student 8,692 PointsThanks! It worked.
But what did i do wrong?
Tushar Singh
Courses Plus Student 8,692 PointsTushar Singh
Courses Plus Student 8,692 PointsWhy is it not working????
Problem is with the last 2 challenges:
truncateString("A-", 1) should return "A...".
truncateString("Absolutely Longer", 2) should return "Ab..."
Please help me out here!