Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial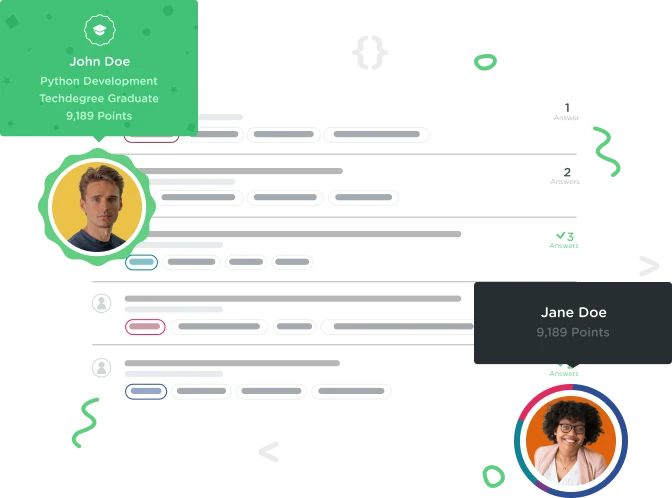
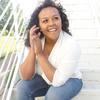
Enzie Riddle
Front End Web Development Techdegree Graduate 19,278 PointsSlice with Steps
Here's the question:
Great work! OK, this second one should be pretty similar to the first. Make a new function named first_and_last_4. It'll accept a single iterable but, this time, it'll return the first four and last four items as a single value.
What am I doing wrong? PLEASE explain in a way that is easy to understand: I am new to this!
def first_4(items):
for iterables in items:
return items[0:4]
def first_and_last_4(single_iterable):
for value in single_iterable:
return single_iterable[0:4] and single_iterable[-1:-5:-1]
2 Answers
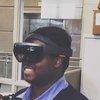
Guled Ahmed
12,806 PointsHey Enzie,
There is a problem with your first method first_4
. I'll walk you through this step by step. In your function first_4
you initially created a loop. The job of a loop is to go through all items found in a list one by one. The assignment said to return the first 4 items. There are many ways to do this. One way could be using a for
loop to store only 4 items in a separate list and return that list or simply use slicing to easily return 4 items. In your first function you tried to do a little of both, I'll help you fix that.
What's actually happening in your function first_4
is that you you're telling the computer "For each item in my list (items
) I want you to return the first 4 things in that list". Rather than looking through the list one item at a time and simultaneously trying to give us back the first 4 items we should simply return the first 4 items right away using slicing in Python like so:
def first_4(items):
# Give me all items in the list `items` between index 0 to index 4
return items[0:4]
Now for the second method first_and_last_4
. You attempted to use a for loop here again which is not necessary. We just want to use slicing to extract the first 4 and the last 4 items in the list. Here's how we can do that. We already know how to get the first 4 items in the list all we have to do now is figure out how to get the last 4 items and how to put the first 4 items and last 4 items into one list.
Let's start off by removing the loop:
def first_and_last_4(single_iterable):
return single_iterable[0:4] and single_iterable[-1:-5:-1]
Now we have the first 4 items represented by single_iterable[0:4]
which is great, but we have a problem. You got the last 5 items but their reversed and you didn't put everything into one list. Let me explain. I'm going to use a little syntax cheatsheet that will help me when slicing in Python here it is:
[start:stop:step]
# start - The index we will start from
# stop - The index we will end at
# step - The steps we'll take when traversing the list (ex: step of 1 would mean to go through the list 1 item at a time)
And let's use an example list called my_list
to visualize all of this:
my_list = [1,2,3,4,5,6,7,8,9,10]
Note: when you use negative numbers when slicing in Python you are actually traversing the list backwards.
What you tried to do is start at -1 which is the last item of the list (10), stop at -5 which represents 6 and step -1 which means to move one item at a time going backwards starting from the last element of the list which is 10 in this case.
The final result would look like:
[10,9,8,7,6]
What we actually want is:
[7,8,9,10]
Here's how we can achieve this and there are many ways to do this. I'll do the easiest one. I want to start at the 4th to the last item in the list. This would be -4, so we should have:
[-4
So far so good. Now, I want everything after the 4th to the last item of the list so my end will be the last item of the list. Now remember when slicing in Python when you specify the end what you really get is end-1 (what you actually get is the item before the end index). To account for this we can simply leave end blank which tells the computer to just grab everything from -4 to the end of the list. Now we should have:
[-4:]
To combine (or concatenate) two lists together you use the +
symbol. Our final solution is:
def first_and_last_4(single_iterable):
return single_iterable[0:4] + single_iterable[-4:]
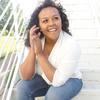
Enzie Riddle
Front End Web Development Techdegree Graduate 19,278 PointsThank you so much! I've never had anyone answer my question so clearly. I really appreciate it!

Temiye Oluseun
2,902 Pointsdef first_and_last_4(single_iterable): return single_iterable[0:4] + single_iterable[-4:]
not working still giving Oops! It looks like Task 1 is no longer passing.
Ali Dahud
3,459 PointsAli Dahud
3,459 PointsBut It excludes the last 4th item doesn't it?
charles foust
7,854 Pointscharles foust
7,854 PointsExcellent explanation! Really helped me understand. Thanks Guled!