Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial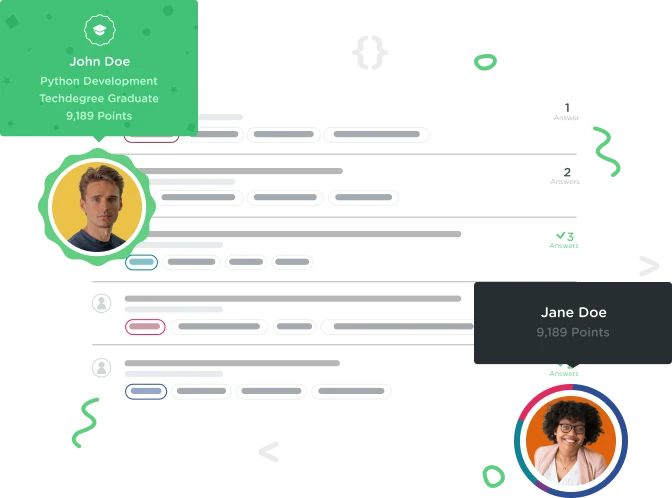

Hoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsSlight confusion about naming collisions, private member variables, getters and methods.
Hello people,
can someone clear up the following concerns I have.
- First of all in this Java Data Structures course we have been introduced to using 'm' to prevent naming collisions. In previous courses we used "this.". I think there is no difference but why does workspace highlight "this." as a keyword and not 'm'?
2.Allthough I know what member variables are, I struggle to visualize what is actually happening in the methods when we want to expose them to the users of our object. When we declare member variabels we don't want the users of our object to change them, we make it immutable by making it private. A public constructor is made were we initialize the variables with a 'm' in front to prevent naming collisions with the private member fields. But now nothing happened yet because the users of our object can't see anything. So we make methods named get followed by the member variables. Here is my confusion: what is actually happing when we make these methods. What happens when we name the method getSomething and return the mSomething? Didn't we just initialize in the constructor that mSomething = something?
3 Answers
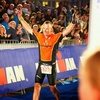
Steve Hunter
57,712 PointsHi there,
Two question - 2 answers; here's the first.
this
is a keyword; it is used, as you say, to distinguish between variables of the same name being used within the same scope. Another way of avoiding this is to use the prefix m
on the member variable. This is just the same as calling it a different name - it isn't a reserved word, it is just a (deprecated?) naming convention. I've lashed together some code to illustrate - this isn't supposed to be perfect so, if it isn't, don't let me know!
public class RandomClassName{
private String mName;
private String anotherName;
public RandomClassName(String anotherName, String name){
this.anotherName = anotherName; // need to use this as they're called the same
mName = name; // different names so no confusion
this.mName = name; // also correct
}
}
You can avoid the conflict by either using this
to specify the instance variable, or by calling the two variables, the instance variable and parameter, something different. Or both.
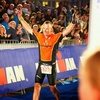
Steve Hunter
57,712 PointsTWO:
we make it immutable by making it private - that's not what we're doing, no. We're making it accessible only via the way the class creators intended. A variable that is private
is not immutable. It just has restricted access.
... we make methods named get ... what is actually happing when we make these methods. Getter and setter methods do what they say; they get the value held by a member variable or they set the value of that member variable (hence they're not immutable, just private
).
What happens when we name the method getSomething and return the mSomething? We're using the value held by that member variable. We decided to store it for a reason - that reason is because we want to use its value as part of the class responsibility.
Didn't we just initialize in the constructor that mSomething = something? Yes we did, but the parameter that is passed into the constructor isn't available anywhere else. It is out of scope of any other method; it only exists in the constructor. To preserve its value, we store it inside the instance which we then can access using the getters and setters.
Let me lash together another poor example ... a thermometer (no idea why, it just came to me and I'll probably regret this in a few lines' time!). Again, this code is for illustration purposes only - it likely won't work but I'm not going to put it through an IDE for the sake of an example.
public class Thermometer{
private float mDegreesKelvin;
public Thermometer(float degrees){
// parameter passes in the absolute temperature
mDegreesKelvin = degrees;
}
public float getDegreesKelvin(){ // could/should also be private
return mDegreesKelvin;
}
public float convertToCelsius(){
return getDegreesKelvin() - 273.15;
}
}
Right. I think that's what I'm trying to illustrate. I'll post this now then add the next paragraph in a minute.
[EDIT] So, the constructor receives a number from the outside world - that's anything; it could be passed in as an argument to the main
method, it could be received from a sensor on the Space Shuttle - the class doesn't care - it gets a number, creates an instance of the Thermometer
class and stores that received value in the member variable, which is private.
Some of our users don't understand what Kelvin means so we want to display the temperature in units they understand, Celsius. This conversion is dead easy, each increment of a degree is the same with Celsius and Kelvin, they just start counting at a different place. We need to deduct 273.15 degrees to convert from K to C.
The member variable is private
so I've created a getter method to demonstrate how they work. I call this getter inside the convertToCelsius
method. That returns the value minus 273.15.
This example is trite, though - what the private
accessor restriction is trying to do is to stop direct access through the instance of the class. Like this:
// I'm in another class or the main method - it doesn't matter.
Thermometer greenhouseTemp = new Thermometer(greenhouse); // greenhouse is a number - go with it!
// this is illegal - no access
console.printf("The temperature in the greenhouse is %f", greenhouseTemp.mDegreesKelvin - 273.15);
// this is OK - but risky in this scenario
console.printf("The temperature in the greenhouse is %f", greenhouseTemp.getDegreesKelvin() - 273.15);
// this is the intention:
console.printf("The temperature in the greenhouse is %f", greenhouseTemp.convertToCelsius());
We prevent direct access to the member variable but we can still call the getter from outside the class. We may (and probably should) want to restrict that to private
too, so that access the temperature is only via the public
method called convertToCelsius()
which guarantees that the user cannot be confused by looking at a Kelvin temperature. We can control what the user sees by limiting access to that temperature figure such that Celsius is only ever presented. Opening that airlock at 10 degrees Kelvin would be a mistake; at 10 degrees Celsius, it's fine! We can use restricted access to make sure the user only sees what they're expecting and understand. A combination of getters and helper methods can ensure this happens.
A tenuous example, and I apologise, I'm not a teacher for a reason! But I hope that gave you some insight into how my warped mind understands the concepts you asked about.
Questions? Fire away!
Steve.

Hoessein Abd
Python Web Development Techdegree Graduate 19,107 PointsThank you Steve. Your example with the thermometer made it click for me.
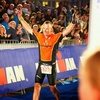
Steve Hunter
57,712 PointsGlad it helped.
Steve.