Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial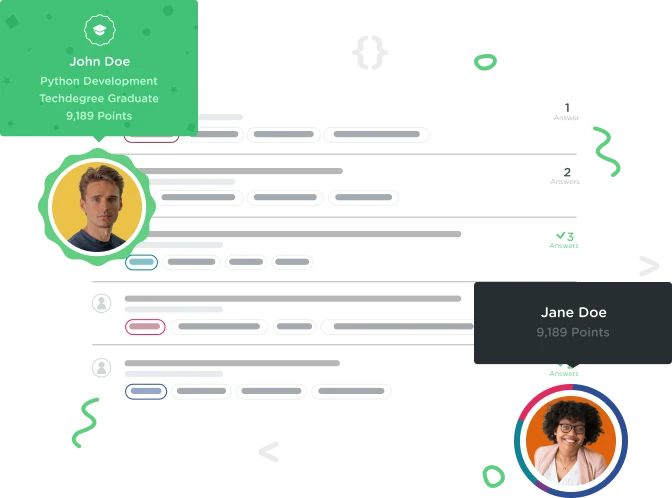
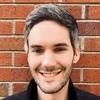
Jesse Vorvick
6,047 PointsSlightly different solution. Is this valid?
Here's my solution. The main difference is for step 1, I used concat
for the two arrays. In order for it to work, however, I had to change the const teachers
array to let teachers
. Is this equally valid, or not preferable? And why?
/*
1) Write the code for function addNewTeachers() found on line 35. This function should receive parameter newTeachers, defined on line 52, and add the new teachers to the treehouseTeachers variable.
2) Find function printTreehouseSummary(). There is something you need to fix in this function so the console.log() on the final line of the function outputs the correct number of JavaScript courses and teachers.
3) At this point, attempt to run your code by typing node let_const.js in your workspace console and hitting enter. You should receive an error message when you do this. Use the clues in this error message to fix the program so it runs.
*/
let teachers = [
{
name: 'Ashley',
topicArea: 'Javascript'
}
];
const newTeachers = [
{
name: 'James',
topicArea: 'Javascript'
},
{
name: 'Treasure',
topicArea: 'Javascript'
}
];
const courses = ['Introducing JavaScript',
'JavaScript Basics',
'JavaScript Loops, Arrays and Objects',
'Getting Started with ES2015',
'JavaScript and the DOM',
'DOM Scripting By Example'];
const i = courses.length;
function addNewTeachers(newTeachers) {
// TODO: write a function that adds new teachers to the teachers array
teachers = teachers.concat(newTeachers);
}
function printTreehouseSummary() {
// TODO: fix this function so that it prints the correct number of courses and teachers
for (let i = 0; i < teachers.length; i++) {
console.log(`${teachers[i].name} teaches ${teachers[i].topicArea}`);
}
console.log(`Treehouse has ${i} JavaScript courses, and ${teachers.length} Javascript teachers`);
}
addNewTeachers(newTeachers);
printTreehouseSummary();
1 Answer
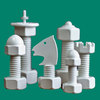
Steven Parker
231,269 PointsThis is certainly "valid" in the sense that it produces the required result, but it's not optimal for two reasons:
- it's less efficient because it copies the original array instead of adding to it
- it gives up the protection that "const" provided for the array