Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial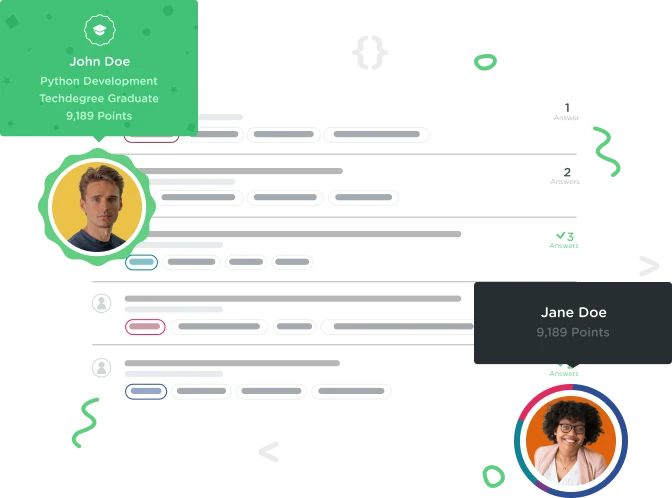
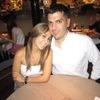
Antonio Jaramillo
15,604 PointsSlim 3.0 code for this lesson
This is not a question, but rather some code to help those who are struggling following this lesson while using Slim 3.0. Here is an excerpt from the Slim 3.0 release blog and is very important: "Slim’s HTTP request and response abstractions support PSR-7. This means their interfaces differ significantly from previous releases." To read more about it go here: http://www.slimframework.com/2015/12/07/slim-3.html
The request->post() method no longer works. Instead, we are to make use of PSR-7 HTTP message interfaces. What is that, you ask? Go here: http://www.php-fig.org/psr/psr-7/#3-2-1-psr-http-message-serverrequestinterface
After some research, I found that the method getParsedBody() , which is part of the PSR-7 specifications, does the trick. It does exactly what it's meant to do; it parses the request body. If you var_dump this then you essentially get what you would have wanted with the deprecated(?) post().
Here is my Slim 3.0 code up to this point of this course:
<?php
// From Slim 3
use \Psr\Http\Message\ServerRequestInterface as Request;
use \Psr\Http\Message\ResponseInterface as Response;
// Use Composer's autoload feature to autoload vendors
require __DIR__ . '/vendor/autoload.php';
// Create Slim 3.0 container. **This is required**
$container = new \Slim\Container;
// Register Slim 3.0's Twig-view component in container.
$container['view'] = function ($c) {
$view = new \Slim\Views\Twig('templates', [
'cache' => false
]);
$view->addExtension(new \Slim\Views\TwigExtension(
$c['router'],
$c['request']->getUri()
));
return $view;
};
// Create new Slim app. Make sure to pass in $container. Passing these three parameters into
// the closure/callback are standard Slim 3.0 syntax.
$app = new \Slim\App($container);
// Render Twig template in route
$app->get('/', function (Request $request, Response $response, array $args) {
return $this->view->render($response, 'about.twig');
// New standard for naming routes.
})->setName('home');
// Render Twig template in route
$app -> get('/contact', function (Request $request, Response $response, array $args) {
return $this->view->render($response, 'contact.twig');
})->setName('contact');
// var_dump form request
$app->post('/contact', function (Request $request, Response $response, array $args){
$body = $this->request->getParsedBody();
var_dump($body);
});
// Run app
$app->run();
?>
Note that you no longer have to call use($app). Your callback functions using the get() method will reference $app with $this.
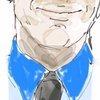
Abraham Juliot
47,353 PointsHere's a bundle of likes to your post. Much appreciated! :)
5 Answers

Tim Knight
28,888 PointsMaybe Hampton Paulk could include a link to this in the teachers notes for the course for students trying Slim 3?
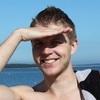
Kristjan Reinsberg
50,960 Pointsjust replace your code with...
$app->post('/contact', function ($request, $response, $args) {
$body = $this->request->getParsedBody();
var_dump($body);
});
Your problem was... (Request $request, Response $response, array $args....
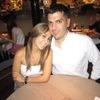
Antonio Jaramillo
15,604 PointsHmm? My code worked fine without your suggestions. Both solutions work.
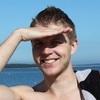
Kristjan Reinsberg
50,960 PointsAlso you want to receive those post elements..
//************ loop and echo all post elements*********** $post_items = $this->request->getParsedBody();
foreach($post_items as $key => $item){
echo $item;
}
//************ recive single post element***********
// recieve your POST variables
$post_items = $this->request->getParsedBody();
//Single POST/PUT parameter
echo $post_items['name'];
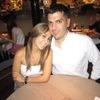
Antonio Jaramillo
15,604 PointsThat part is covered in the next lesson : )
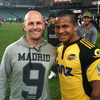
Bryn Humphreys
26,472 PointsThank you!
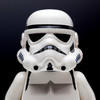
Petrov von Petrov
21,916 PointsThanks for posting $this, Antonio Jaramillo, it's a great time-saver. The out-of-dateness of this course is really annoying.
You probably won't care much about this now, but so that other people know, your post method can be trimmed a little bit, like this:
$app->post('/contact', function ($request, $response) {
var_dump ($this->request->getParsedBody());
});
You don't need the $args nor to create the $body variable.
PS: You should select an answer and close the question so it will show as solved in the forum. Many people only go for the green marks.
Cheers
Mattea Turnbull
4,134 PointsMattea Turnbull
4,134 Pointsthanks!