Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial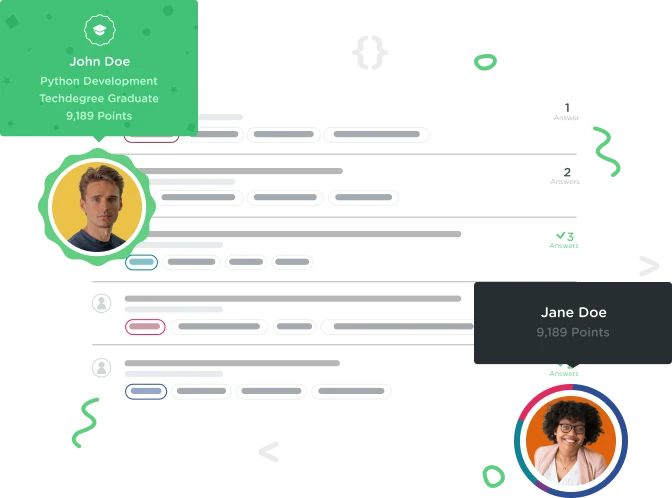
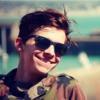
Romuald Le Bris
3,975 PointsSlim Application Error Message || PLEASE HELP || Slim v3.2
Hi everyone,
Composer installed the latest 3.2 v of Slim Framework and I'm encountering some difficulties with the routing.
Here is the message I have when trying to reach "/contact" in the URL :
Slim Application Error A website error has occurred. Sorry for the temporary inconvenience.
Here is my code :
<?php
require __DIR__ . '/vendor/autoload.php';
// use Monolog\Logger;
// use Monolog\Handler;
//object variable 'log' which is a new Monolog logger instance of the variable log.
// $log = new Logger('name');
//pushHandler has an object instantiated inside of it. Which is the StreamHandler from Monolog.
//Two arguments, 1/ is what file we're planning on logging to; 2/ is what type of default log level we're going to have.
// $log->pushHandler(new Monolog\Handler\StreamHandler('app.log', Logger::WARNING));
// call the method addWarning passing a string to the addWarning method which will add the 'foo' string to a warning log app.log file.
// $log->addWarning('Oh Noes.');
//*****************************************************************************\\
// \\
// SLIM APP CONFIG \\
// \\
//*****************************************************************************\\
// Create and configure Slim app
$app = new \Slim\App;
// Define app routes
$app->get('/', function() use($app){
$app->render('index.html');
});
$app->get('/contact', function() use($app) {
$app->render('contact.html');
});
// Run app
$app->run();
If someone has a clue for the solution of my problem, please HELP :)
5 Answers

yannm
2,164 PointsHi Romuald, I've had the same problem. Of course it's possible to install the same version of Slim as in the video, but i thought it would be nice to be able to use the latest version too. Here's the solution I've found so far. It works, but it may not be the slimmest solution out there. Don't forget to install the 'slim/twig-view' package in Composer before running it. Here's my code:
<?php
require __DIR__ . '/vendor/autoload.php';
date_default_timezone_set('Europe/Berlin');
$app = new \Slim\App(['settings' => ['displayErrorDetails' => true]]);
// Get container
$container = $app->getContainer();
// Register component on container
$container['view'] = function ($container) {
$view = new \Slim\Views\Twig('template', [
'cache' => false
]);
$view->addExtension(new \Slim\Views\TwigExtension(
$container['router'],
$container['request']->getUri()
));
return $view;
};
// Render Twig template in route
$app->get('/', function ($request, $response, $args) {
return $this->view->render($response, 'index.html'
);
});
$app->get('/contact', function ($request, $response, $args) {
return $this->view->render($response, 'contact.html'
);
});
$app->run();
Have a look at this page, that's where i took most of this code from, and it explains everything: http://www.slimframework.com/docs/features/templates.html
I hope this helps.
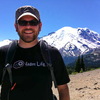
Geoffrey Mortstock
16,196 PointsFollowing the steps the instructor provided, you'll install v3+ which means the steps he's guiding us through won't work. While it would be ideal if the videos were updated to teach the current version of SLIM & ensure that the steps he's guiding us through are accurate, who knows if and when that will happen. Until it does, you can install SLIM v2.6 to follow along:
- Delete your SLIM directory in your workspace
- Go to your console
- Enter "composer require"
- Search for package: SLIM
- Choose option 0 (Slim/Slim)
- Version Constraint: 2.6
Specifying 2.6 will ensure you're following along with the version the instructor is using.
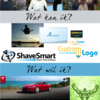
amadeo brands
15,375 PointsBasicly you try to say:
composer require slim/slim "2.6"
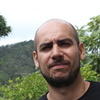
Mike Ribera
4,127 PointsThats the better way to handle it, stick to the 2.6.
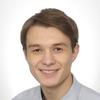
Sergey Podgornyy
20,660 PointsDid you already tried to get access through localhost/index.php/contact ?
Also, I found solution of your problem here:
$settings = [
'settings' => [
'displayErrorDetails' => true,
],
];
$app = new Slim\App($settings);
Hope this will help
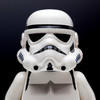
Petrov von Petrov
21,916 PointsThe code used in this video is useless with Slim 3, because the way it renders templates is completely different now.
You have 2 ways to go about it: either using Twig-View, like yannm did, or using PHP-View.
In case you're interested, here's the code for using PHP-View (don't forget to install the component first):
<?php
require __DIR__ . '/vendor/autoload.php';
date_default_timezone_set('Europe/Lisbon');
$app = new \Slim\App(['settings' => ['displayErrorDetails' => true]]);
// Get container
$container = $app->getContainer();
// USING PHP-VIEW
// Register component on container
$container['view'] = function ($container) {
return new \Slim\Views\PhpRenderer('templates/');
};
// Render template in route
$app->get('/', function ($request, $response) {
return $this->view->render($response, 'index.html');
});
$app->get('/contact', function ($request, $response) {
return $this->view->render($response, 'contact.html');
});
// Run app
$app->run();
//That's it!
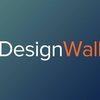
Design Wall
11,126 Points"don't forget to install the component first"
what's that packet?more instruction please?

Thomas Wang
11,335 Points@designwall
composer require slim/php-view
This command works for me

jameso
8,569 PointsI've been trying different solutions for hours, and yours finally works. This is the simplest method to use the current version of Slim. So far this course has been a headache with compatibility issues...
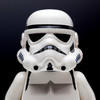
Petrov von Petrov
21,916 PointsPS: yannm, your code can be trimmed a bit. You don't need the cache declaration when registering the component on the container, nor the $args parameter in the the $app->get function.
amadeo brands
15,375 Pointsamadeo brands
15,375 PointsNice fix. I am also trying to do something similar.
But how would this work using this code snipit? Seems they made it more complex than necessary any explanation from where the extra code is needed?
Thank you.