Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial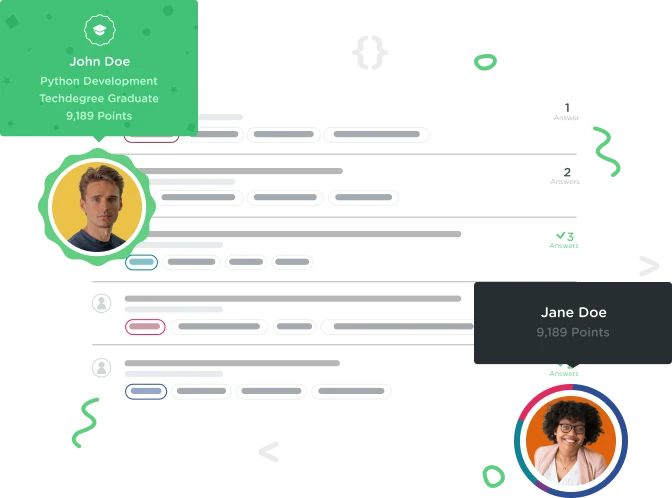

brendon hanson
6,191 PointsSmall Project! NEED HELP! JS
So I am making this little program where I have a "bank" and i can change their accounts and they receive notifications and such. I have a small issue where I have to type an entire separate chunk of code for the prompts for each user and I want to add more ppl to the array but then i'd have to add a whole other section of prompts that are identical to the other prompts except it has that persons number. How can i make this work where I only have to type one set of prompts that only pop up according to the persons account i am on. I tried to o this but the prompts for both accounts appeared when looking at one account. Hope you understand what I'm saying lol. PS: (main issue is in while loop near end of program)
var students = [
{
name: 'luke',
memberSince: 'October 9, 2017',
memberShip: 'Platinum',
OuncesofSilver: 4,
savings: 0,
checking: 1.60,
debts: 0,
booksDue: 0,
points: 50,
goldGrams: 0
},
{
name: 'julie',
memberSince: 'October 9, 2017',
memberShip: 'Gold',
OuncesofSilver: 0,
savings: 0,
checking: 0,
debts: 0,
booksDue: 0,
points: 10,
goldGrams: 0
}
];
let message = '';
let student;
let question;
let security;
//Custom Function that replaces document.write with a better alternative
const print = (message) => {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// These are the reports
const getReport = ( student ) => {
let report = `<h1 class="a"> Client: ${student.name} </h1>`;
report += `<p class="s"> MemberShip: ${student.memberShip} </p>`;
report += `<p class="d"> MemberSince: ${student.memberSince} </p>`;
report += `<p class="f"> Points: ${student.points} </p>`;
report += `<p class="g"> Checking: ${student.checking} </p>`;
report += `<p class="h"> Debts: ${student.debts} </p>`;
report += `<p class="j"> Savings: ${student.savings} </p>`;
report += `<p class="k"> Ounces of Silver: ${student.OuncesofSilver} </p>`;
report += `<p class="l"> Books Due: ${student.booksDue} </p>`;
report += `<p class="z"> GoldGrams: ${student.goldGrams} </p>`;
return report;
}
// transactions, deposits, etc.
// oct. 31, 2017 Luke: Deposit cash
students[0].checking += 2;
// nov. 1, 2017 Luke: Subtract Points
students[0].points -= 50;
// nov. 1, 2017 Luke: Checkout Book- DIary of Wimpy kid: Old School
students[0].booksDue += 1;
// nov. 1, 2017 Luke: Monthly Payment
students[0].checking -= 3.6;
// nov. 1, 2017 Julie: Monthly Payment
students[1].debts += 3;
// nov.1, 2017 Luke: Buy his silver
students[0].OuncesofSilver -= 2;
// nov. 1, 2017 Julie: Less than 20 in checking account
students[1].points -= 50;
// nov. 3, 2017 Luke: Returned His book! Diary of Wimpy Kid Old School. Rating *****
students[0].booksDue -= 1;
students[0].points += 10;
// nov. 3, 2017 Luke: CHeck out Diary of WImpy KidLong Haul
students[0].booksDue += 1;
// nov. 6, 2017 Luke: Renewed his book
// Returned his book LUKE
students[0].booksDue -= 1;
// Add 20 to checking
students[0].checking += 20;
students[0].points += 10;
// Nov. 12: Add $100 to account
students[0].checking += 100;
// nov. 12: Transfer $20 from checking to savings
students[0].savings += 20;
students[0].checking -= 20;
// nov. 13 Luke: Add $1 to savings
students[0].savings += 1;
// nov. 13 Luke: Confidential
students[0].savings -= 1;
// nov. 13 Luke: Add gold
students[0].goldGrams += 2;
// nov. 14 Luke: Checked out Double Down
students[0].booksDue += 1;
// The security code to only allow the person with card to see his/her profile
while (true) {
security = prompt("Type in your card number to continue");
if ( parseInt(security) === 4569) {
question = prompt("Would you like to see our records? Type in your name to see your record and type Quit to exit on the next prompt");
if ( students[0].name === question.toLowerCase() ) {
message = getReport( students[0] );
print(message);
}
if ( students[0].points > 250 ) {
alert(" 1 Month Free for any membership! OR wait for a bigger Reward!");
}
if ( students[0].points > 500 ) {
alert("50% of membership for 5 whole MONTHS! OR wait for better prize!");
}
if ( students[0].points > 1000 ) {
alert("Upgrade to next membership!");
}
if ( students[0].checking < 20 ) {
alert("Your checking account is low on cash! Get it to 20 dollars or you'll lose points soon!");
}
if ( students[0].debts > 0 ) {
alert("You owe money pay it off ASAP!");
}
if ( students[0].booksDue > 0 ) {
alert("You have book(s) due!");
}
if ( students[0].savings < 1 && students[0].OuncesofSilver < 1 && students[0].goldGrams < 1) {
alert("You need to start saving!");
}
break;
} else if (parseInt(security) === 7124) {
question = prompt("Would you like to see our records? Type in your name to see your record and type Quit to exit on the next prompt");
if ( students[1].name === question.toLowerCase() ) {
message = getReport( students[1] );
print(message);
}
if ( students[1].points > 250 ) {
alert(" 1 Month Free for any membership! OR wait for a bigger Reward!");
}
if ( students[1].points > 500 ) {
alert("50% of membership for 5 whole MONTHS! OR wait for better prize!");
}
if ( students[1].points > 1000 ) {
alert("Upgrade to next membership!");
}
if ( students[1].checking < 20 ) {
alert("Your checking account is low on cash! Get it to 20 dollars or you'll lose points soon!");
}
if ( students[1].debts > 0 ) {
alert("You owe money pay it off ASAP!");
}
if ( students[1].booksDue > 0 ) {
alert("You have book(s) due!");
}
if ( students[1].savings < 1 && students[1].OuncesofSilver < 1 && students[1].goldGrams < 1) {
alert("You need to start saving!");
}
break;
} else if ( parseInt(security) === 14 ) {
question = prompt("Would you like to see our records? Type in your name to see your record and type Quit to exit on the next prompt");
message = getReport( students[0] );
print(message);
}
else {
print("<h1>Nice Try HACKER! Wrong Code!" + "</h1>");
throw ( new Error('Wrong Code Fool!'));
break;
}
if (question === null || question.toLowerCase() === 'quit') {
break;
}
}
print(message);
print(message);
3 Answers
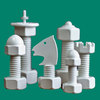
Steven Parker
231,846 PointsSome initial observations:
const notify = ( alert ) => {
while (true) {
if (students.points > 250) {
alert(" 1 Month Free for any membership! OR wait for a bigger Reward!");
}
//...
- if you name the argument "alert" you will not be able to access the system "alert" function
- this function shouldn't need a loop
- you can't access properties on the entire "students" array
- you might consider naming the argument "student" (singular) and access properties on that
- later, when you call the function, pass it the same argument you give to "getReport"
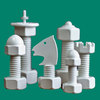
Steven Parker
231,846 PointsA good way to reduce redundant code is with functions.
You could write a function that would handle all the property checking and messages for a student record that was passed in as an argument. Then in your main program you would only need to identify the student and then call the function with their record.
You might make things even more compact by carrying the card number inside each student's record. Then you could loop through them to find a match with the entry and call the function if so, or print the error message if not.
Also, is there a reason to ask for the name, if you have already identified them from the card number?

brendon hanson
6,191 PointsOk, so now that I did the function when I type in code the right account come up but I get no Alerts! Whats wrong...
let message = '';
let student;
let question;
let security;
//Custom Function that replaces document.write with a better alternative
const print = (message) => {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
// These are the reports
const getReport = ( student ) => {
let report = `<h1 class="a"> Client: ${student.name} </h1>`;
report += `<p class="s"> MemberShip: ${student.memberShip} </p>`;
report += `<p class="d"> MemberSince: ${student.memberSince} </p>`;
report += `<p class="f"> Points: ${student.points} </p>`;
report += `<p class="g"> Checking: ${student.checking} </p>`;
report += `<p class="h"> Debts: ${student.debts} </p>`;
report += `<p class="j"> Savings: ${student.savings} </p>`;
report += `<p class="k"> Ounces of Silver: ${student.OuncesofSilver} </p>`;
report += `<p class="l"> Books Due: ${student.booksDue} </p>`;
report += `<p class="z"> GoldGrams: ${student.goldGrams} </p>`;
return report;
}
// transactions, deposits, etc.
// oct. 31, 2017 Luke: Deposit cash
students[0].checking += 2;
// nov. 1, 2017 Luke: Subtract Points
students[0].points -= 50;
// nov. 1, 2017 Luke: Checkout Book- DIary of Wimpy kid: Old School
students[0].booksDue += 1;
// nov. 1, 2017 Luke: Monthly Payment
students[0].checking -= 3.6;
// nov. 1, 2017 Julie: Monthly Payment
students[1].debts += 3;
// nov.1, 2017 Luke: Buy his silver
students[0].OuncesofSilver -= 2;
// nov. 1, 2017 Julie: Less than 20 in checking account
students[1].points -= 50;
// nov. 3, 2017 Luke: Returned His book! Diary of Wimpy Kid Old School. Rating *****
students[0].booksDue -= 1;
students[0].points += 10;
// nov. 3, 2017 Luke: CHeck out Diary of WImpy KidLong Haul
students[0].booksDue += 1;
// nov. 6, 2017 Luke: Renewed his book
// Returned his book LUKE
students[0].booksDue -= 1;
// Add 20 to checking
students[0].checking += 20;
students[0].points += 10;
// Nov. 12: Add $100 to account
students[0].checking += 100;
// nov. 12: Transfer $20 from checking to savings
students[0].savings += 20;
students[0].checking -= 20;
// nov. 13 Luke: Add $1 to savings
students[0].savings += 1;
// nov. 13 Luke: Confidential
students[0].savings -= 1;
// nov. 13 Luke: Add gold
students[0].goldGrams += 2;
// nov. 14 Luke: Checked out Double Down
students[0].booksDue += 1;
// The security code to only allow the person with card to see his/her profile
security = prompt("Type in your card number to continue");
const notify = ( alert ) => {
while (true) {
if ( students.points > 250 ) {
alert(" 1 Month Free for any membership! OR wait for a bigger Reward!");
}
if ( students.points > 500 ) {
alert("50% of membership for 5 whole MONTHS! OR wait for better prize!");
}
if ( students.points > 1000 ) {
alert("Upgrade to next membership!");
}
if ( students.checking < 20 ) {
alert("Your checking account is low on cash! Get it to 20 dollars or you'll lose points soon!");
}
if ( students.debts > 0 ) {
alert("You owe money pay it off ASAP!");
}
if ( students.booksDue > 0 ) {
alert("You have book(s) due!");
}
if ( students.savings < 1 && students.OuncesofSilver < 1 && students.goldGrams < 1) {
alert("You need to start saving!");
}
break;
}
}
if ( parseInt(security) === 4569) {
message = getReport( students[0] );
print(message);
notify(alert);
}
if ( parseInt(security) === 7124) {
message = getReport( students[1] );
print(message);
notify(alert);
}
print(message);
print(message);
brendon hanson
6,191 Pointsbrendon hanson
6,191 PointsThanks for your help! I found a fix! I created a variable i and put it after each student property in function so whenever a code was typed the var i would change to either 0 or 1 depending on the code and then it would show prompts for only that account. It didn't work at first because i used "let" instead of "var" when i made the variable 'i' so i was wondering what the issue was then i remembered a video talking about scope and needless to say I changed let to var and it worked...thanks again!
Steven Parker
231,846 PointsSteven Parker
231,846 PointsI'm glad you got it working but that's not quite what I was suggesting. What I had in mind would not require a global "i" and would use the passed-in student record to check the properties. It might look like this:
Then you would call it like this: