Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial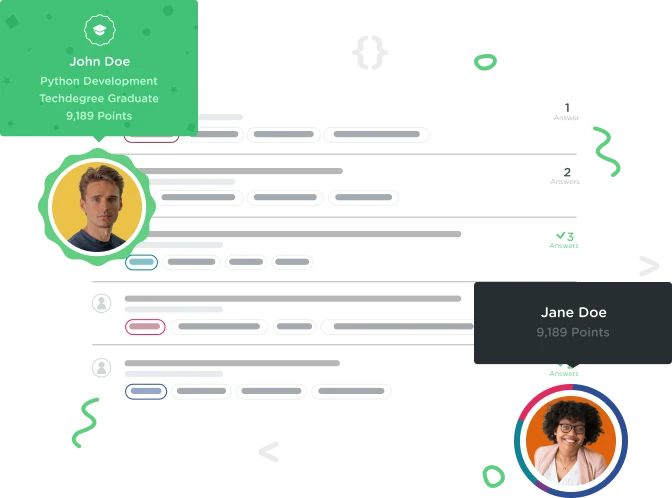

prasannakulkarni2
14,918 PointsSo back to that ScrabblePlayer. I found that it's not enough to know if they just have a tile of a specific character.
I am stuck on this challenge. This is the only challenge I have to complete for me to complete the Java Objects course. I am not sure exactly how to use the for loop. I think it has to be used on mHand somehow.
Could you please help me out? Thanks a lot!
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
}
3 Answers

Chase Marchione
155,055 PointsHello Prasanna,
- You need to create a method called getTileCount. Your method needs to return an integer (the count), and it needs to take in a char (the letter to check for.)
- You would want to initialize the count as 0, and before your conditional logic.
- You need to use a for loop to loop through the tiles.
- After initializing the counter variable, you need to write the for loop (so that you can 'run' through each tile.) Then, you need to check if the particular tile matches. If the test is met, you would increment the counter, because there would be a match.
- You need to return the count when it is ready to be returned (after all tiles are checked.)
Example code, if you're still stuck:
public int getTileCount(char t){
int count = 0;
for (char tile : mHand.toCharArray()){
if (tile == t) {
count++;
}
}
return count;
}
}

prasannakulkarni2
14,918 PointsThank you so much for the elaborate answer. It does makes sense.

daviddavis3
4,904 PointsThank you, thank you, thank you!
Yes, the object of this challenge is to pick a letter (variable t), then check all the tiles in mHand for how many, if any, match the letter. Wow!
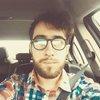
George Siaskov
1,292 PointsThank you!
prasannakulkarni2
14,918 Pointsprasannakulkarni2
14,918 PointsKen Alger Help please!