Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial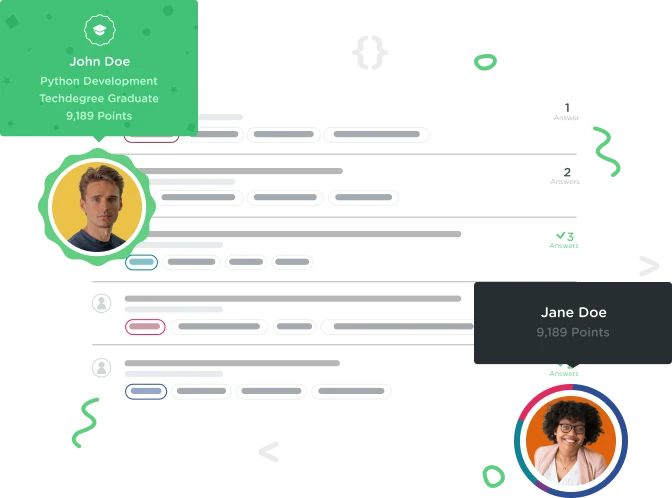

ahmed alghafli
3,446 PointsSo back to that ScrabblePlayer. I found that it's not enough to know if they just have a tile of a specific character. W
how I return count ?
So back to that ScrabblePlayer. I found that it's not enough to know if they just have a tile of a specific character. We need to know how many they actually have. Can you please add a method called getTileCount that uses the for each loop you just learned to loop through the characters and increment a counter if it matches? Return the count, please, thanks!
public class ScrabblePlayer {
private String mHand;
public ScrabblePlayer() {
mHand = "";
}
public String getHand() {
return mHand;
}
public void addTile(char tile) {
// Adds the tile to the hand of the player
mHand += tile;
}
public boolean hasTile(char tile) {
return mHand.indexOf(tile) > -1;
}
public String getTileCount() {
for (char tile: mHand.toCharArray()) {
if(mHand.indexOf(tile) >=0) ;
}
}

ahmed alghafli
3,446 Pointsthanks to all of you
4 Answers
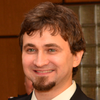
Miroslav Kovac
11,454 PointsDo you mean something like this?
public int getTileCount(char tile) {
int count = 0;
for (int i = 0; i < mHand.length(); i++) {
if (mHand.charAt(i) == tile)
count++;
}
return count;
}

Craig Dennis
Treehouse TeacherThat will work, you can use a for-each loop to loop through the hand too
for (char letter : mHand.toCharArray()) {

Kyler Callaway
6,257 Pointsthe only think that I don't understand in regards to this logic is that the char variable tile is not initialized so how can it be compared to a method or even another variable if the tile variable has no value

ahmed alghafli
3,446 Pointsammmm now I understand what is going on
thanks Craig ..
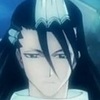
Ethan Smith
877 PointsI'm having the same problem and I got confused with all these different answers but what line would I put the, for (char letter : mHand.toCharArray()) {
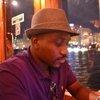
Steve Fielding
7,287 Pointsyou can try this <code> public int getTileCount(char tile) { int count = 0; for(char letter : mHand.toCharArray()) //Because we want to compare each tile with the actually tile in hand if(letter == tile) count++; </code>

Teferi Heye
3,448 Pointspublic int getTileCount(char tile){ int count =0; for(char mtile: mHand.toCharArray()){ if(mHand.indexOf(mtile)== mHand.indexOf(tile)){ count++; }
}
return count;
}
Andrew Nguyen
2,470 PointsAndrew Nguyen
2,470 PointsHi, if your getTileCount does your method return a String or an int? If it is an int then you can simply do.
How ever if you are returning a string then: