Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial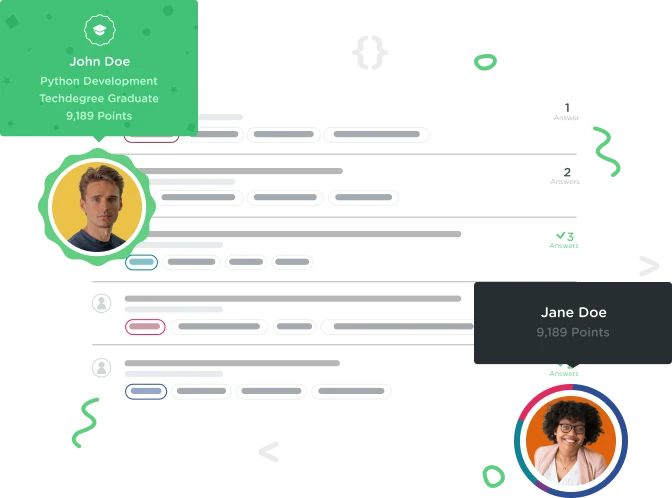
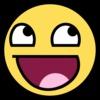
Marcus Schumacher
16,616 PointsSo close but need help :)
I don't see why the User.getLastName and get first name doesn't work here.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
//constructor
public ForumPost(User author, String title, String description) {
mAuthor = author;
mTitle = title;
mDescription = description;
}
public String getDescription() {
return mDescription;
}
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
private String mFirstName;
private String mLastName;
public User(String firstName, String lastName) {
mFirstName = firstName;
mLastName = lastName;
}
public String getFirstName() {
return mFirstName;
}
public String getLastName() {
return mLastName;
}
}
public class Forum {
private String mTopic;
private String topic;
public String getTopic() {
return mTopic;
}
public Forum(String topic) {
mTopic = topic;
}
public void addPost(ForumPost post) {
System.out.printf("New post from %s %s about %s.\n",
User.getFirstName(),
User.getLastName(),
ForumPost.getDescription());
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
Mark Miller
45,831 PointsMark Miller
45,831 PointsI think you must create an object of type User and then you can invoke its getter methods for the first and last names. This is because the Forum class is not an inner, or nested, class inside the User class. If you were calling the methods from inside of the User class, then it would be okay to user User.getFirstName() as you have here. But you are calling the methods from outside the class, so an object must first be created before the method of that class can be used.
Also, the static keyword can be used to change the normal behavior, I think. That's why you've done that before. It was either inside of that class, or the static keyword had been declared, enabling the class to invoke its properties outside of the class declaration file.
https://en.wikibooks.org/wiki/Java_Programming/Keywords/static
http://docs.oracle.com/javase/tutorial/java/javaOO/classvars.html