Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial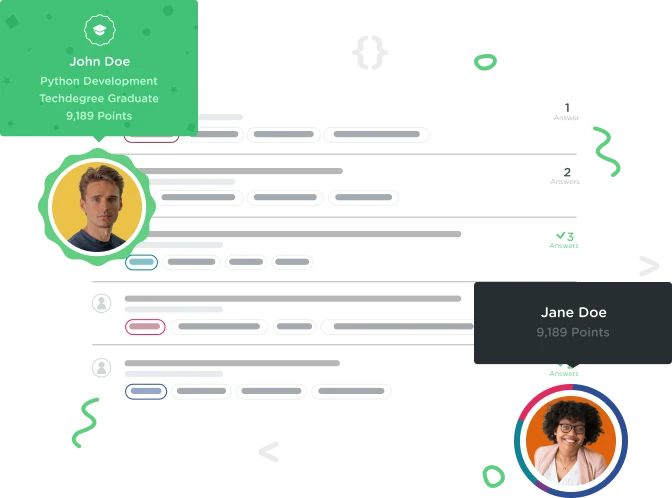

Martin Hansen
2,228 PointsSo close, now so confused - help wanted
I feel like I'm very close, been piecing this one together for so long I think I'm doing more harm than good. I get the error Bummer: time data '%y-%m-%d' does not match format '%m/%d/%y %H:%M'
I'm sadly a bit of a completionist, and just can't get this one..
Here is my code:
Examples
to_string(datetime_object) => "24 September 2012"
from_string("09/24/12 18:30", "%m/%d/%y %H:%M") => datetime
import datetime
def to_string(datetime_object):
date_format = datetime_object.strftime('%d %B %Y')
return date_format
def from_string(string_date, strf_time):
string_date = datetime.datetime.strptime("09/24/12 18:30", "%m/%d/%y %H:%M")
strf_time = string_date.strptime(strf_time, "%m/%d/%y %H:%M")
return strf_time, string_date
2 Answers
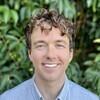
Asher Orr
Python Development Techdegree Graduate 9,409 PointsHi Martin! The second challenge says the from_string function must:
- take two arguments: a date as a string and an strftime-compatible format string
- return a datetime object created from them.
def from_string(string_date, strf_time):
string_date = datetime.datetime.strptime("09/24/12 18:30", "%m/%d/%y %H:%M")
strf_time = string_date.strptime(strf_time, "%m/%d/%y %H:%M")
return strf_time, string_date
In your code, you define the from_string function and pass in the correct arguments. The issue is with the body of the function.
Let's return to the instructions: The challenge asks to return one datetime object created, using your two arguments.
def from_string(string_date, strf_time):
string_date = datetime.datetime.strptime("09/24/12 18:30", "%m/%d/%y %H:%M")
#the line above creates a variable that contains a datetime object
#it takes the arguments "09/24/12 18:30" & "%m/%d/%y %H:%M"
strf_time = string_date.strptime(strf_time, "%m/%d/%y %H:%M")
#not sure what's going on with the line above.
return strf_time, string_date
#here, you are returning whatever the strf_time variable represents, then the datetime object (string_date)
When you return the string_date variable, you ARE returning a datetime_object. It's just not using the string_date and strf_time arguments. You passed your own arguments into it ("09/24/12 18:30", "%m/%d/%y %H:%M")
Upon clicking "Check Work," the Treehouse checker passes in its own arguments for string_date and strf_time. It will look something like this:
def from_string("whatever the date string is", '%y-%m-%d'):
string_date = datetime.datetime.strptime("whatever the date string is", '%y-%m-%d')
It's expecting to see whatever it passes in as the strf_time argument (in this case, the format '%y-%m-%d'), but your code is setup so that '%m/%d/%y %H:%M' is always your strf_time compatible format string.
Do you see the problem? If you:
- Adjust your string_date variable (you should change the name of that variable to datetime_object, by the way, so that Python knows whether you're referring to the function argument or the variable) to accept your function arguments
then
- Return just that variable
.. It should pass the challenge.
I hope this helps. Let me know if you have questions!
PS- whenever you post a question about a specific challenge, be sure to attach the challenge to your question. This is done by clicking the "Get Help" button on the challenge, then posting your question. It will attach automatically.

Martin Hansen
2,228 PointsThank you for the reply. It was my first post, so forgot the assignment question. I tried using your reply, but gotta say, I'm still so confused about what it wants me to do. I'm two seconds from just skipping the timedate section for a few weeks. Here is what I tried with your answer, and got the message: Bummer: time data '%y-%m-%d' does not match format '%y-%m-%d'. .... So the formats are the same, but don't match. I think I'm missing something with the arguments and the body of the function still..
def from_string(string_date, strf_time):
string_date = datetime.datetime.strptime("09/24/12 18:30", "%m/%d/%y %H:%M")
datetime_object = string_date.strptime("%y-%m-%d", "%y-%m-%d")
return string_date
One last try?
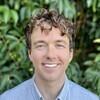
Asher Orr
Python Development Techdegree Graduate 9,409 PointsNo worries! Let me explain what the challenge is asking for, and why you're still getting that error message.
WHAT THE CHALLENGE IS ASKING FOR
It's asking you to create a function (from_string) that makes one datetime object from 2 strings.
Imagine that I'm using Python to manipulate a database. Many databases have a column called "Date" that only accept datetime objects. You can't enter a string that LOOKS like a datetime object in it ("09/24/12 18:30"), or you'll get an error.
If I have a list of strings that look like a datetime object, and I need to convert them to actual datetime objects in order to add them to the database, I might use a function like from_string
WHY YOU'RE STILL GETTING THAT ERROR MESSAGE
def from_string(string_date, strf_time):
Remember that when you click "Check Work," Treehouse is going to pass in its own argument in place of string_date and strf_time.
You're using your own arguments in the body of your function: datetime.datetime.strptime("09/24/12 18:30", "%m/%d/%y %H:%M"). Instead, you should be using the function parameters.
The following code should pass the challenge:
def from_string(date_as_string, strftime_compatible_format_string):
datetime_object = datetime.datetime.strptime(date_as_string, strftime_compatible_format_string)
return datetime_object
Does this make sense?

Martin Hansen
2,228 PointsYour example with the database makes sense, and I do understand what is going on theoretically. The technical side though, this "time" part of Beginning Python has been severely more difficult than the others. Often the solution is much simpler than what I've been digging myself into.
Thanks a bunch, and especially for also posting a solution ;)