Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial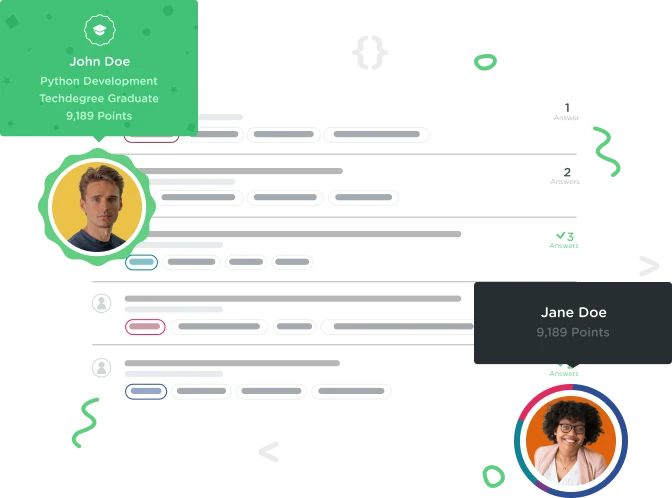

Erik Faye Karla
1,523 Pointsso how do i change the x and y coordinants??
i want to change the place robot is in but I dont know what code I need to do that!
class Point {
var x: Int
var y: Int
init(x: Int, y: Int){
self.x = x
self.y = y
}
}
class Machine {
var location: Point
init() {
self.location = Point(x: 0, y: 0)
}
func move(direction: String) {
print("Do nothing! Im a machine!")
}
}
// Enter your code below
class Robot: Machine {
override init(){
self.location = Point(x: 1, y: 1)
}
override func move(direction) -> String {
switch direction {
case "Up": Robot.y = y + 1
case "Down": Robot.y -= 1
case "Left": Robot.x -= 1
case "Right": Robot.x += 1
default: break
}
}
}
2 Answers

Florin Veja
6,912 PointsErik,
You do not need to override init in this one, because you do not define any properties, so you do not need to initialize them. Everything will get initialized with the init defined in the Machine class.
When you override the move method, you should not have the String return, as it is not returning anything. I believe you may have misplaced it and you should place it as the type of the direction parameter.
override func move(direction: String)
Lastly, instead of Robot.y or Robot.x, it should be self.location.y and self.location.x
See my complete answer in another post I recently answered, if you're still having trouble.
Florin

Erik Faye Karla
1,523 Pointsthank you! After I figured out I could press the swift recap 2 over my question i found the post you linked! thanks anyway