Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial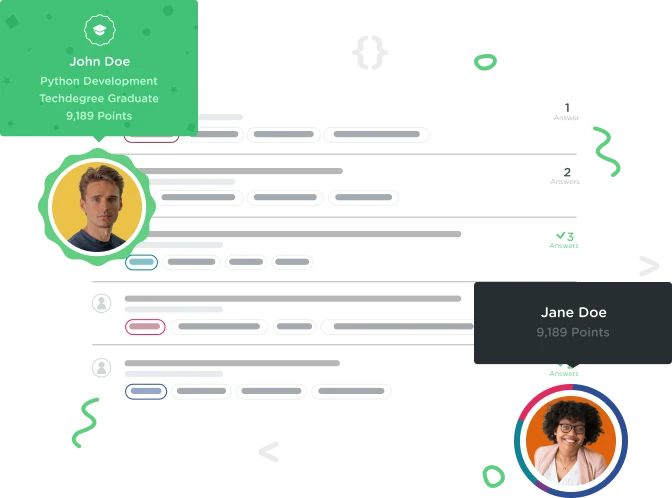

Ryan Bleecker
509 PointsSo i have rewatched the video and reread my code several times now but I cannot get the frog to move in play mode.
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class PlayerMovement : MonoBehaviour {
private Animator playerAnimator;
private float moveHorizontal;
private float moveVertical;
private Vector3 movement;
private float turningSpeed = 20f;
private Rigidbody playerRigidbody;
// Use this for initialization
void Start () {
// Gather components from the Player GameObject
playerAnimator = GetComponent<Animator> ();
playerRigidbody = GetComponent<Rigidbody> ();
}
// Update is called once per frame
void Update () {
// Gather input from the keyboard
moveHorizontal = Input.GetAxisRaw ("Horizontal");
moveVertical = Input.GetAxisRaw ("Vertical");
movement = new Vector3 (moveHorizontal, 0.0f, moveVertical);
}
void FixedUpate () {
//if the players movement vector does not = 0...
if (movement != Vector3.zero) {
//...then create a target rotation based on the movement vector...
Quaternion targetRotation = Quaternion.LookRotation (movement, Vector3.up);
//and create another rotation that moves from the current rotation to the target rotation
Quaternion newRotation = Quaternion.Lerp (playerRigidbody.rotation, targetRotation, turningSpeed * Time.deltaTime);
//..and change the players rotation to the new incremental rotation...
playerRigidbody.MoveRotation (newRotation);
//then play the jump animation.
playerAnimator.SetFloat ("Speed", 3f);
} else {
//Otherwise dont play the jump animation
playerAnimator.SetFloat ("Speed", 0f);
}
}
}
Moderator edited: Added markdown to make the code render properly on the forums. -JN
Thoughts? Only thing I did was when prompted I changed the line endings form Unix to windows.

Ryan Bleecker
509 PointsYeah its in there as a component.
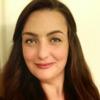
Jennifer Nordell
Treehouse TeacherI've looked at your screenshot and there are at least a couple of things that stand out (to me). You have some things which appear grayed out, where Nick's does not. Is it possible that you made some move within the file system to relocate your project and can you absolutely confirm that you've saved all files and the scene?
Specifically, your Player
, PlayerController
and PlayerMovement
script all appear to be grayed out. I feel like if we can figure out why that is, we'll have the answer to your question.

Ryan Bleecker
509 PointsPlayerMovement is the only one grayed out, from what I can tell my highlighting of player is color highlighting it and Playercontroller i cant really tell. But yes I did notice that my Playermovement is grayed out...not sure where to begin with that one...I did go back and ctrl s both my script and the scene

Ryan Bleecker
509 Pointshttps://gyazo.com/897aa973c65c811906e6e3aee7486675
Turned the debugger on and it looks like its not reading my script? Also according to the Unity forums, the field being grayed out is standard as of 2015 and you need to switch the inspector to debug mode to ungray it. However, it is saying that player animator is none...
Jennifer Nordell
Treehouse TeacherJennifer Nordell
Treehouse TeacherHi there! I've also looked at your code, and can't see the error which makes me suspect that there might not be one. So, let's check some basics. When you select the object, can you see the
PlayerMovement
class connected as a component? Make sure it looks like what is shown in 9:02 of this video. Also, make sure you've saved the scene. Let me know if these are correct but you're still not able to move the frog!