Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial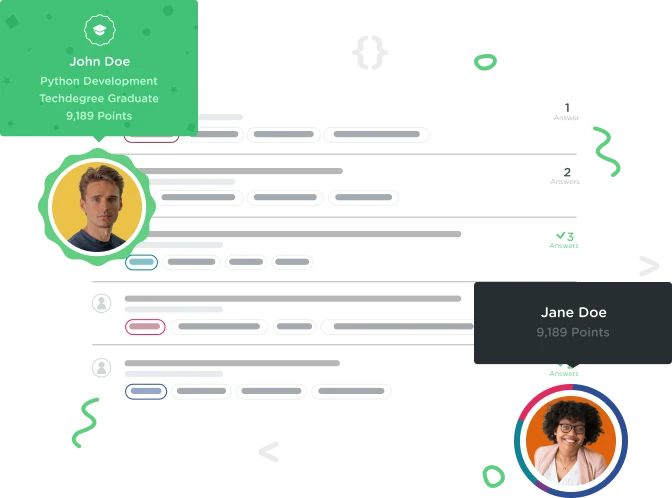

Russell Brookman
3,118 PointsSo, I just started this over for the 5th time. It doesn't seem like it should be that hard.
I just reset the code before asking this question because I got to a point were I was confusing myself.
I am mostly looking for a starting point. Please explain what they are asking for in each category without actually answering the question for me. I want to figure it out myself but I need a little help getting started.
public class ForumPost {
private User mAuthor;
private String mTitle;
private String mDescription;
public User getAuthor() {
return mAuthor;
}
public String getTitle() {
return mTitle;
}
// TODO: We need to expose the description
}
public class User {
public User(String firstName, String lastName) {
// TODO: Set the private fields here
}
}
public class Forum {
private String mTopic;
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
public class Example {
public static void main(String[] args) {
System.out.println("Starting forum example...");
if (args.length < 2) {
System.out.println("first and last name are required. eg: java Example Craig Dennis");
}
// Forum forum = new Forum("Java");
// Take the first two elements passed args
// User author = new User();
// Add the author, title and description
// ForumPost post = new ForumPost();
// forum.addPost(post);
}
}
1 Answer

Simon Coates
28,694 Pointsstep1:
public class Forum {
private String mTopic;
public Forum(String topic){
mTopic = topic;
}
public String getTopic() {
return mTopic;
}
public void addPost(ForumPost post) {
/* When all is ready uncomment this...
System.out.printf("New post from %s %s about %s.\n",
post.getAuthor().getFirstName(),
post.getAuthor().getLastName(),
post.getTitle());
*/
}
}
STEP1: oh, without answering the question. Step1 is pretty unambiguous. add constructor that accepts a string and sets mTopic.
STEP2: Switch to user object, add private fields to store values fed into the constructor. So you want private string fields for firstName and lastName. The naming convention being applied tends to have members start with m (eg. mTopic on forum). You can look at Forum if you get stuck on private member strings. Next add lines to User constructor to set the fields using the values passed to that constructor. The final part is adding getters for the member variables you created (forum has an example of a getter for mTopic if you get stuck)
Step 3: Add a constructor for ForumPost, It should receive values to set the member fields of the class definition (the order of values passed in is important). Then add a getter for mDescription (there are a couple example getters in this class).
Step 4: Remove entire lines starting with /* and */ in Forum.java's addPost (NOT THE LINES INBETWEEN). Switch to Example.java and remove the // from lines that are obviously code. Now debugging. stop reading here if you want to have a go at debugging on your own. okay, fine, good. the first error is on the line with new User(); The problem is that when you define a User constructor with arguments, the default no arg constructor is no longer available. As such, you need to pass in the values expected by the user constructor (these need to be the values passed in when the main method is called). The problem with new ForumPost() is the same as new User() - so just feed in values.
Simon Coates
28,694 PointsSimon Coates
28,694 Pointsif the desire to solve it yourself fades, there is some code that is supposedly complete at https://teamtreehouse.com/community/delivering-the-mvp-wrapping-up-last-challenge