Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial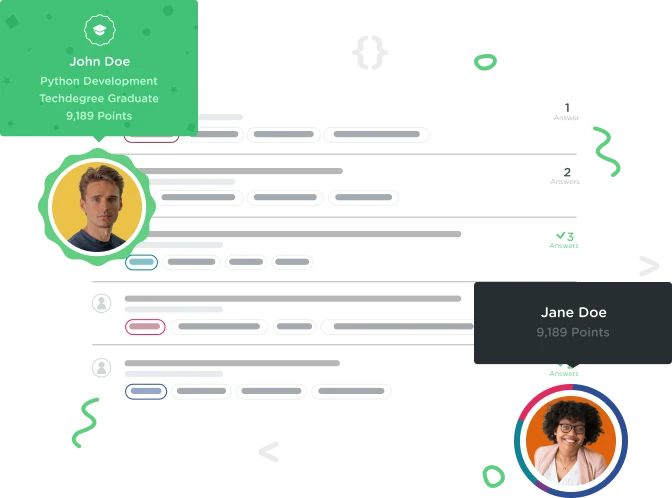

Mathew Yangang
4,433 PointsSo I keep getting "didn't get the right pattern"
I am returning the requested the value, but I keep getting "didn't get the right pattern", any idea what I am doing wrong here?
class Letter:
def __init__(self, pattern=None):
self.pattern = pattern
def __iter__(self):
yield from self.pattern
def __str__(self):
output = []
for blip in self:
if blip == '.':
output.append('dot')
else:
output.append('dash')
return '-'.join(output)
@classmethod
def from_string(cls, pattern):
fix_me = Letter(["_" "."])
return fix_me
class S(Letter):
def __init__(self):
pattern = ['.', '.', '.']
super().__init__(pattern)
1 Answer
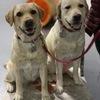
Alex Koumparos
Python Development Techdegree Student 36,887 PointsHi Mathew,
The question is telling you:
Create a class method in Letter named from_string that takes a string like "dash-dot" and creates an instance with the correct pattern (['_', '.']).
The use of the word "like", combined with the fact that you are being asked to make this method part of the Letter
class, indicates that your method should be able to take any string represented by some sequence of "dash" and or "dot" separated by hyphens and transform it into a list of corresponding underscore and period characters.
You should also note that ['_', '.']
(the example provided in the question) and ['_' '.']
(what you are using to construct your Letter instance) are not equivalent. The example's will create a Letter with a string value of "dash-dot" while yours will create a Letter with a string value of "dash".
Hope that points you in the right direction.
Cheers
Alex
Mathew Yangang
4,433 PointsMathew Yangang
4,433 PointsHi Alex, thanks for the help but I just added a , in between like ([".", "_"]), but I am still getting the same results "Didn't get the right pattern"
Alex Koumparos
Python Development Techdegree Student 36,887 PointsAlex Koumparos
Python Development Techdegree Student 36,887 PointsHi Mathew,
As I mentioned before, the fundamental issue with your code is that it returns the same output no matter what is provided as input.
Your code is supposed to transform the input into the appropriate output. If, as in the example, you are provided
"dash-dot"
, then your method should create a Letter with a pattern of['_', '.']
. But, if you are provided"dot-dot-dot"
, then your method should not create a Letter with a pattern of['_', '.']
. Instead, it should create a Letter with a pattern of['.', '.', '.']
.Hope that was more clear,
Cheers Alex