Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial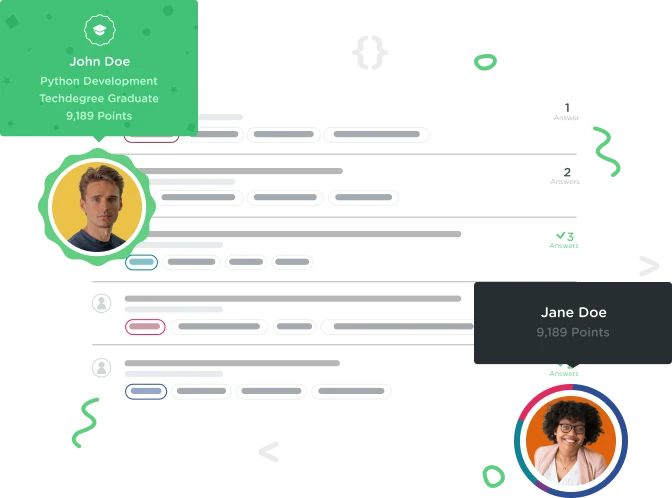
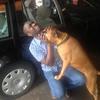
Kurt Daisley
4,228 PointsSo, I understand what a static method is .... but what does adding the keyword 'static' do to a variable we've declared?
I understand what the keywords 'public', 'private', and 'final' do to the variable, but, although I understand what a static method is, I'm not clear on the effect that it has on a variable. Could someone provide an example?
Addendum: Okay, so I've gone over the 'Constants' video a couple times, and I'm guessing that the 'static' keyword makes the value of our class variable constant or fixed - just like Integer.MAX_VALUE is a constant that can't be changed. Am I on the right track here?
3 Answers

Felix Sonnenholzer
14,654 PointsHi,
a static variable, or method, or whatsovever just means that is it in memory even if its class is not instantiated.
Simply speaking it means I can have cross-class access to it.
e.g. Integer.MAX_VALUE
is something you might want to have access to in other classes without having to create an Integer object for every class you might want to use it.
We have two classes:
ExampleClass1
public class ExampleClass1 {
public static String coolString = "my cool string!";
}
Normally you would have to create an instance of that Class to have access to the variable. Like this.
public class ExampleClass2 {
public static void main(String[] args) {
ExampleClass1 ex1 = new ExampleClass1();
System.out.println(ex1.coolString);
}
}
But if it is static you can access it like this:
public class ExampleClass2 {
public static void main(String[] args) {
System.out.println(ExampleClass1.coolString);
}
}
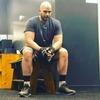
Timothy Hilley
2,292 PointsOk so:
public class SomeClass {
static String example = "Hey im an example!";
public String getExample() {
return example;
}
}
this is our class with the static variable, and here is why we would do so:
treat it like a method in the way of: it is now a variable assigned a value that you can use in every class the getter method is now redundant. There is no need for a getter method for static variables becuase it can be accessed by all classes in the form of SomeClass.example
public class differentClass {
SomeClass.example; //returns: Hey im an example!
A static variable is considered a class variable. A class variable can be accessed directly with the class, without the need to create a instance
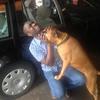
Kurt Daisley
4,228 PointsThank you Timothy and Felix! Much clearer now. :-)