Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial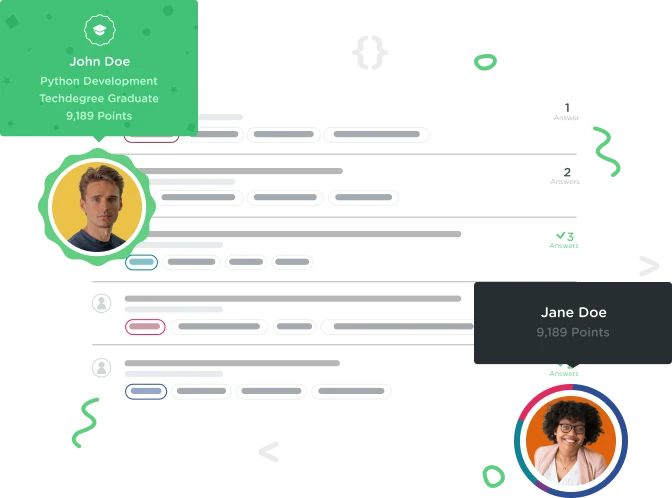
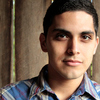
Eric Tirado
iOS Development Techdegree Student 7,036 PointsSo is it ok to use the '==' operator in cases such as form-validation where the answer is not case sensitive?
Is it ok to use the '==' operator in cases such as form-validation where the answer is not case sensitive, vs using '===' operator and having to make conditional statements based on all possible letter casing?
For example, will this work? prompt: "Who killed Goliath?" user answers all lowercase: "david"
var answer = prompt('Who killed Goliath?');
if ( answer == 'David' ) {
document.write("That's right!");
} else {
document.write("Sorry, try again!");
}
3 Answers
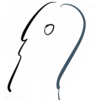
akak
29,446 Points== is case sensitive. What == does is that it doesn't compare types (and to be more specific it allows coercion while === does not). In either case david will not equal David.
The best way to approach this is to forcefully change the answer to lower or uppercase and then compare.
if ( answer.toLowerCase() === 'david' )
at this point answer DAViD, dAVID or david will be accepted since before comparison they are all made lowercase.
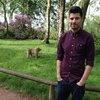
Danny Alder
9,119 PointsThe difference between == and === become more apparent when comparing certain variable types, such as boolean (True or False). The == operator will try to convert types to match which means it would convert TRUE to 1 and FALSE to 0. This can cause unintended behaviour in certain situations if you are not sure how the operators work.
0 === FALSE // Would result in a false return
0 == FALSE // Would result in a true return
The second condition returns true because the browser converts the FALSE statement to a 0. This does not always matter, there may be times that the programmer wants 0 to be the same as FALSE and other times when the programmer does not. As long as you understand what the two operators do in terms of type conversion then it does not matter which you use as long as you know it will return the result you intend. This link explains other unintentional behaviour the == operator can cause.
I feel it is suggested to always use the === operator as this means the values must be EXACTLY the same so there is less room for error, especially for beginner programmers who may overlook the difference in operators when trying to debug a program. From reading different sources many professional coders suggest always using the === operator and manually making type conversions so you are in control instead of the browser and it is easier to determine what you are looking for.
// Automatic Conversion
if ( "50" == 50) {}
// Manual Conversion
if ( parseInt("50") === 50) {}

Rails Duck
8,545 PointsBut then technically, you could use the double equal sign if you use the toLowerCase operator... right?
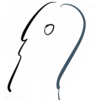
akak
29,446 PointsYes, in that case you could. But I think it's a best practice to use == only in cases when === is too restrictive. Here it's definately not. Only if for some reason you would like 123 == '123' to be true - then you should use ==. In other cases, I would suggest to stick to ===.
Eric Tirado
iOS Development Techdegree Student 7,036 PointsEric Tirado
iOS Development Techdegree Student 7,036 PointsI see. Thanks for that. I had my suspicion that there was a more reliable way to do it. Thanks for helping me level up.
akak
29,446 Pointsakak
29,446 PointsYou're welcome. Glad I could help :-)