Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial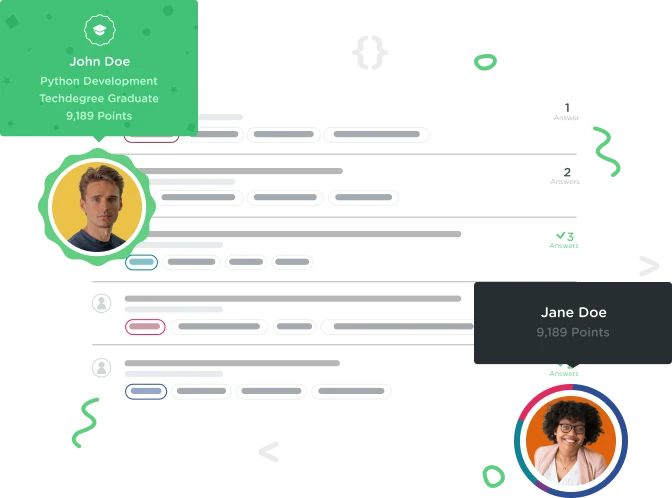

Devin Francis
13,377 PointsSo lost, need HELP!
I have watched, rewatched, and rewatched the MAPS video over 20 times and still cannot figure out what the heck they are wanting for this?!?!?! It is becoming ridiculous because they do not touch base on what they are asking us to do!! I have no code typed up right now because I have it over in a text editor instead so I can see it in action before submitting.
package main
import "fmt"
func main () { prices := map[string]float64{"OCKOPROG": 89.99, "ALBOOMME": 129.99, "TRAALLA": 49.99} prices2 := map[string]float64{"OCKOPROG": 89.99/2, "ALBOOMME": 129.99/2, "TRAALLA": 49.99/2} fmt.Println(prices) fmt.Println(prices2)
}
I can get it to work by running it this way, but it is not what they are asking for.
package sales
func HalfPriceSale(prices map[string]float64) map[string]float64 {
// YOUR CODE HERE
}
5 Answers

Daniel Boisselle
Front End Web Development Techdegree Student 17,438 PointsHello friend! I have just started learning Golang myself as well! Try to not get so frusturated
func HalfPriceSale(prices map[string]float64) map[string]float64 {
halvedPrices := map[string]float64{}
for item, value := range prices {
halvedPrices[item] = value/2
}
return halvedPrices
}
HalfPriceSale is a FUNCTION and we need to RETURN a new MAP that's the easiest way to explain what the prompt is asking!
Please reach out if you have any questions!! Daniel
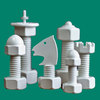
Steven Parker
230,274 PointsYou might be confusing things by working outside the challenge and not having boilerplate to simulate how the validator works. Some of the differences you need to account for:
- the challenge is to create only a function, no complete program or "main" is used
- the challenge doesn't require you to "print" anything
- the challenge does require you to "return" the result
- the challenge will supply test data that you won't see (and will not be like the example)
An approach to solving this task you might consider is to create a new "map" variable and then construct a loop to go through the one that is given as the argument. Inside the loop, you could add an element to the new map using the name of the one from the incoming argument and half of the price as the value. Then when the loop has finished, return the new map.

Devin Francis
13,377 PointsBut when I remove the "return" i get he error, "missing return at end of function FAIL sales". So therefore it is asking me for a return..... And honestly, all that just confused me more.
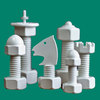
Steven Parker
230,274 PointsThe return is essential, that's one of my points. See my other comments on the code you re-posted.

Devin Francis
13,377 Pointspackage sales
func HalfPriceSale(prices map[string]float64) map[string]float64 {
product := map[string]float64{"OCKOPROG": 89.99, "ALBOÖMME": 129.99, "TRAALLÅ": 49.99}
for product, prices := range products {
products[product] = prices/2
}
return products
}
Then what am I doing wrong?
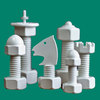
Steven Parker
230,274 PointsThat's close! But don't pre-fill "product" with static data. And do initialize "products" as an empty map.
Also, the argument name is "prices" so use a different name for your loop variable. But use "prices" as the iterator.

Devin Francis
13,377 PointsBut don't pre-fill "product"
I have to establish what is in "product" before linking the second part of the task to the new name "products", don't I?
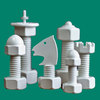
Steven Parker
230,274 PointsNo, "product" gets filled in with one item at a time by the loop.

Daniel Boisselle
Front End Web Development Techdegree Student 17,438 PointsThe main function is happening in the background while your 'HalfPriceSales' is the function being tested
package main
import (
"fmt"
"reflect"
)
func main () {
prices := map[string]float64{"OCKOPROG": 89.99, "ALBOOMME": 129.99, "TRAALLA": 49.99}
expected := map[string]float64{"OCKOPROG": 44.995, "ALBOOMME": 64.995, "TRAALLA": 24.995}
result := HalfPriceSale(prices)
//this checks that both maps are equal of type and value, and in this case we are expecting 'true' to print.
fmt.Println(reflect.DeepEqual(expected, result) )
}
func HalfPriceSale(prices map[string]float64) map[string]float64 {
halvedPrices := map[string]float64{}
for item, value := range prices {
halvedPrices[item] = value/2
}
return halvedPrices
}

Devin Francis
13,377 Points... func HalfPriceSale(prices map[string]float64) map[string]float64 {
prices = map[string]float64{
"OCKOPROG": 89.99,
"ALBOOMME": 129.99,
"TRAALLA": 49.99,
}
halvedPrices := make(map[string]float64)
for product, value := range prices {
halvedPrices[product] = value / 2
}
return halvedPrices
} ...
Here is what I have come up with.. And I am still getting the 0.00 error??
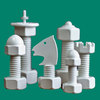
Steven Parker
230,274 PointsYou do not need any fixed data in your function, and you certainly don't want to overwrite the "prices" parameter. The challenge will be passing in the real data to you in it.

Ralph Bankston
8,631 PointsThink of the data they show you as just "example" data that you know what it looks like. You just need to take the name, prices bits and put it in a for loop assign to a new map that you return.