Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial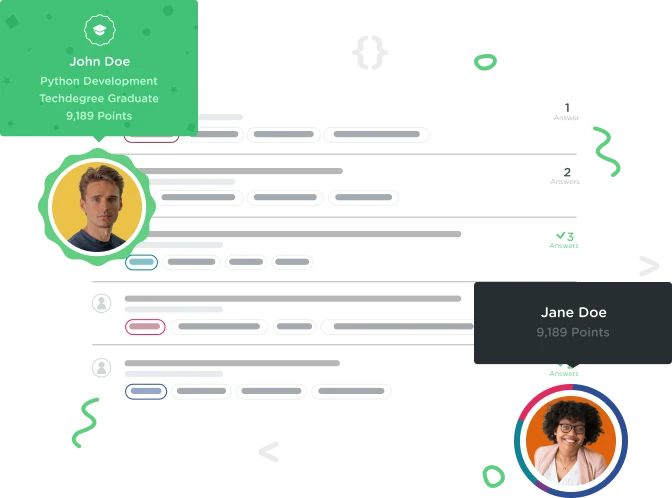

Dillon Reyna
9,531 PointsSo many [5] questions on the Templates video
1. Why do we have to nest our template files? (I.e. courses/templates/courses)
2. What is a context dictionary exactly? What happens if we don't include this?
3. Why is the Key of this context-dict 'courses'?
4. Why is the finished page located at '/courses' and not '/courses/course_list.html'?
5. When creating a templates folder for the project, why do we not have to include a folder inside of "learning_site/templates" called "learning_site"?
2 Answers

Ryan S
27,276 PointsHi Dillon,
Those are all good questions and I'll try to answer them for you.
The reason for the nested directories is for namespacing. Because apps in Django are designed to be able to be reused and plugged into other projects, this allows you to package all the necessary templates with your app and avoid naming conflicts. If two apps in the same project had a template with the same name, you wouldn't need to go through and change them. The namespacing would keep them distinct from one another.
A context dictionary is what is used to pass a variable to your template. If you don't include it, then you won't have access to any variables in your template.
The key of this context dictionary is the name of the variable you are going to use in your template. The value is the
courses
queryset that you defined in the view. They are named the same simply because it makes sense. You could give the key a different name if you wanted. It would still hold the value of thecourses
queryset when you reference it in your template.When a url pattern is matched in Django, it will call its associated view function. The view function is what renders the template to the browser and you don't need to specify the actual template name in the URL like you would with a static site. If you recall from earlier videos, you don't even need to use a template to render something to the browser. You could just give a text response.
You could namespace your main templates directory, but it is unnecessary. When Django looks for templates to render, it looks for a 'templates' subdirectory in each of your installed apps in addition to the main templates directory of your project. Since all of your app's templates are namespaced, there won't be any conflicts with your project templates.
Hope this clears things up.

Dillon Reyna
9,531 Pointsdef course_detail(request, pk):
course = Course.objects.get(pk=pk)
return render(request, 'courses/course_detail.html', {'course':course})
So let's use this bit of code as context.
Question 1 makes sense now - thanks for that!
Question 2 - You'll see in the Context Dict above, we have the key 'course' with a value of 'course'. Now, if I understand correctly, the value is simply equal to the course variable set one line above. If this is correct, when does the key 'course' come into play? Where is it used?
2.A On line 3, What is 'pk' and why do we set it equal to itself?
Question 3 and 5 make sense.
Could I get an ELI5 (Explain Like I'm 5) on Question 4?

Ryan S
27,276 Points2) In that snippet of code, the 'course' key will be used in the course_detail.html
template. Say you wanted to display certain attributes of the particular course that you just got from the database:
{{ course.title }}
{{ course.description }}
In this example, the 'course' variable in your template is the key from your context dictionary, and is equal to the specific course instance passed in as its value. If you changed the name of your key to 'my_course', you'd need to change your template:
{{ my_course.title }}
{{ my_course.description }}
I'll try to answer 2A and 4 together since they are related.
pk
stands for "primary key" and by default is equal to the id of the course instance. If you create a new course instance in the database, it will be assigned a new id. So every course in the database has a unique id, and therefore a unique primary key.
Here, you are not setting it equal to itself. The .get()
method has a "pk" keyword argument (the left side of the equal sign). The value which we are assigning to the argument originally comes from the URL. It has been decided to also name it "pk".
The urlpattern that is associated with this view will have a named group in the regex called "pk". When you enter a URL, and enter along with it a primary key where Django is expecting to find it, it will pass it along with the request to the course_detail view.
So, for example, in this project the url pattern is the following:
url(r'(?P<pk>\d+)/$', views.course_detail)
Note that this url has a prefix of 'courses/' that is defined in the main urls.py.
So if you went to "localhost/courses/1", then our pk would have a value of 1 and would be passed to the view, which would in turn be utilized by the .get()
method. This would get the course with an id of 1, and you'd use the context dictionary to make use of it in the course_detail.html template.
Dillon Reyna
9,531 PointsDillon Reyna
9,531 PointsThis was meant to be a reply to your reply.
Whoa - that makes sense! Thanks for breaking down the 'pk' map for me, that really cleared things up.
I'm gonna have to read this over a few times, but I'm glad to say It makes sense.
I marked your initial answer as "Best" :)