Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial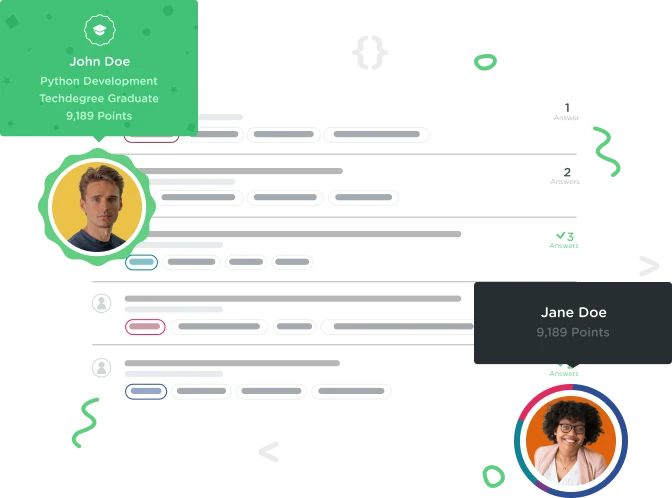
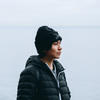
Zhenghao He
Courses Plus Student 2,389 PointsSo many questions about this video
Hi guys I'm new to React and I got really confused watching this video
in GuestList.js file, the teacher wrote
const GuestList = props =>
<ul>
{props.guests.map((guest, index) =>
<li key={index}>
<span>{guest.name}</span>
<label>
<input type="checkbox" checked={guest.isConfirmed}/> Confirmed
</label>
<button>edit</button>
<button>remove</button>
</li>)}
</ul>;
It seems to me that GuestList is a component, if it is, why there is not render()? and about the props being passed in this function, we have
<GuestList guests={this.state.guests}/>
at App.js file so I assume that the props being passed in is this.state.guests i.g. the array guests. My question is when accessing the props, why do we need to write
{props.guests.map((guest, index) =>....
instead of
{props.map((guest, index) =>....
since the props itself is the array?
Sorry guys I know maybe my question is trivial but I just found the React Basics course here is kind outdated so I just jump into this course. Do you guys have any other study resources for React beginners ?
4 Answers

Bruno Junqueira
31,740 PointsHi.
Your first question, we don't need the render method on a stateless component, just in a stateful component. This is a example of a stateful component.
class Hello extends React.Component {
constructor(props) {
super(props);
this.state = {value: 'Hello World'};
}
render() {
return(
<input
ref={(c) => this.input = c}
type="text"
value={this.state.value}
onChange={() => this.setState({value: this.input.value})}
/>
)
}
}
A stateful component is used, basically, when you have to save and manipulate a local state on the component.
This is a example of a stateless component:
const Hello = (props) =>
<div>Hello World</div>
A stateless component is used, basically, when you have to only show data. But it can contain dynamic data passed from props.
Your second question, we need to specify which prop you are going to map, because you can pass multiple props, example:
<GuestList guests={this.state.guests} otherProp="something" anotherProp="somethingElse" />
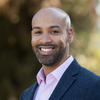
Alfredo Pabon
328 PointsI am still getting the following message, even when I have type EVERYTHING exactly as in the video. Please help :(
TypeError: Cannot read property 'map' of undefined GuestList 3 | 4 | const GuestList = props => 5 | <ul>
6 | {props.guests.map((guest, index)=> 7 | <li> 8 | <span>Joel</span> 9 | <label>

Brian Liotti
917 Pointsdoes not render (at timestamp 1:50). You need to wrap the jsx (<ul> to </ul>) in a div

Jacob Mishkin
23,118 PointsWithout looking at the entire code, My guess would be that "guests" is an object that you are mapping over, pulling out the index and the individual guest. There should be a React updated course, or if not I would go with the basics course then jump into the update for React course.