Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial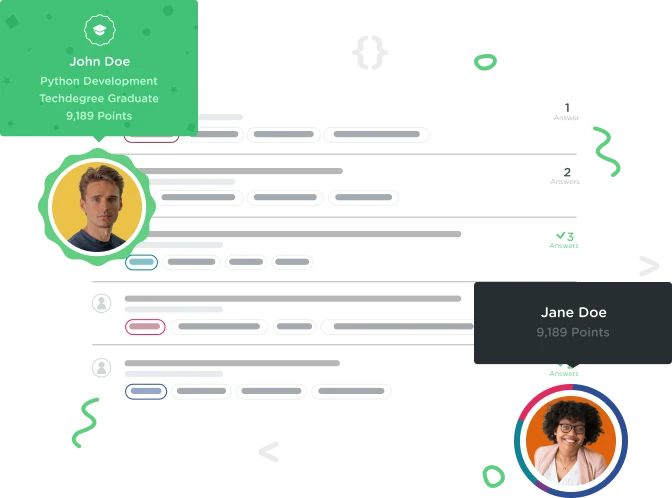
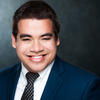
Jonathan Fernandes
Courses Plus Student 22,784 PointsSo my code is different than the offered solution... Is this good or bad? Mind giving me some feedback?
Here's my code:
import random
chances = 1
secret_number = random.randint(1,10)
print("What number am I thinking of that is between 1-10? You get 5 chances.")
while True:
users_guess = int(input(">>> "))
if chances == 5:
print("You ran out of chances. My number was {}. Sorry... :(".format(secret_number))
break
elif users_guess == secret_number:
print("YOU FIGURED IT OUT! My number was {}! It only took you {} tries too!".format(secret_number,chances))
break
else:
if users_guess > secret_number:
print("Oh no, you guessed to high")
elif users_guess < 1 or users_guess > 10:
print("Sorry, but that isn't a number between 1-10. Make your next guess between 1-10.")
else:
print("Oh no, you guessed to low")
chances = chances + 1
continue
4 Answers

Andrei Fecioru
15,059 PointsHi Jonathan,
Here's my opinion:
I am not sure that the
continue
statement at the end of thewhile
loop is necessary. The loop will execute the next iteration automatically.I see you are trying to do a little bit of input data validation there by checking whether the input is in the expected range. But that assumes that the user inputs an integer at the prompt. What if the user passes something else (like an arbitrary string or float)? I would wrap the
input()
function call intry/except
blocks:
try:
users_guess = int(input(">>> "))
except TypeError:
print("Sorry, but that isn't a number between 1-10. Make your next guess between 1-10.")
continue # here you actually need to start the loop over
Other than that it looks ok to me.
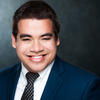
Jonathan Fernandes
Courses Plus Student 22,784 PointsSweet! So I incorporated the input check. Forgot about that actually. And I used the shorthand which I should know from coding in swift. But yeah, thanks for the response!

Carlos Vasconcelos
3,344 PointsI didn't use any loop, stead a function that calls itself, beside check out my if i thing is really cool, but tell me if i am wrong
import random
randNum = random.randint(1, 10)
userChoice = 0
tryNum = 0
def pregunta():
try:
userChoice = int(input('Adivina el numero entre 1 y 10\n>'))
except TypeError:
print('Eso no es un numero!')
if userChoice != randNum:
if userChoice < randNum:
print("El numero es mayor que {} \n".format(userChoice))
pregunta()
else:
print("El numero es menor que {} \n".format(userChoice))
pregunta()
else:
print('felicidades!')
pregunta()

eric93
625 PointsHi. This is what I did. I too am curious if it's ok that I've styled my code differently than what was demonstrated in the video. Kindly share some feedback? Thank you in advance.
import random
guesses_taken = 0
print("Pick a number between 1 and 10.")
print("You only get 5 tries.")
num = random.randint(1, 11)
while guesses_taken < 5:
guess = int(input("> "))
guesses_taken += 1
if guess < num:
print("Your number is too low.")
elif guess > num:
print("Your number is too high.")
else:
if guess == num:
break
# if correct
if guess == num:
guesses_taken = str(guesses_taken)
print("Correct! You've got the answer in {} tries!".format(guesses_taken))
# if incorrect
else:
if guess != num:
num = str(num)
print("I'm sorry. The correct number was {}.".format(num))
Andrei Fecioru
15,059 PointsAndrei Fecioru
15,059 PointsI see that the instructor also does the input type checking I have suggested for you (I just got the chance to watch the video) so I guess you were already aware of that.
P.S. There is s shortcut for incrementing the
chances
counter:chances += 1