Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial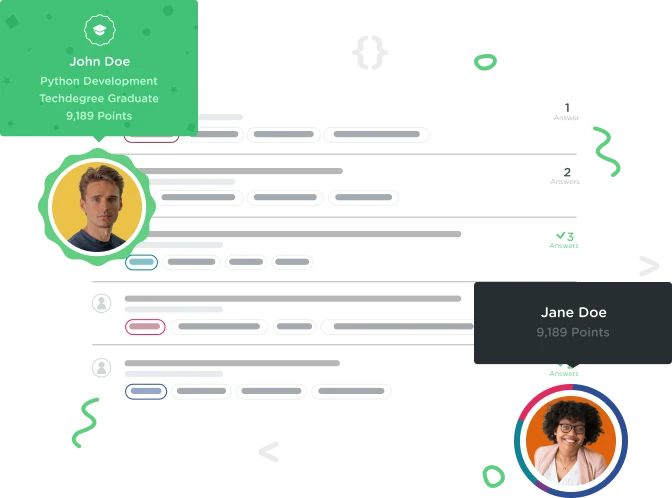
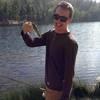
aronlilland
8,951 Pointsso what exactly is the advantage structs offer over classes if it seemingly is the same amount of code?
#the original code sample
class Customer
attr_accessor :name, :email
def initialize(name, email)
@name, @email = name, email
end
end
customer = Customer.new("Jason", "jason@teamtreehouse.com")
puts customer.inspect
#struct example given
Customer = Struct.new(:name, :email) do
def name_with_email
"#{name} <#{email}>"
end
end
customer = Customer.new("Jason", "jason@teamtreehouse.com")
customer.each_pair do |name, value|
puts "#{name} - #{value}"
end
the video example doesnt make it seem like structs are worth it, what are some examples in the wild that would make them a good solution?
2 Answers

Todd MacIntyre
12,248 PointsYou are not comparing the same thing.
class Customer
attr_accessor :name, :email
def initialize(name, email)
@name, @email = name, email
end
end
vs
Customer = Struct.new(:name, :email)
6 lines of code vs 1 line of code.

Derek Lin
1,563 PointsStruct is a fast way to create a class with attributes, without explicitly adding in attr-accessors for assigning the attributes in the constructor.
Erik Nuber
20,629 PointsErik Nuber
20,629 PointsThe video was just an example showing of the concept. He mentions in the waning moments that structs are good for running a few lines of code but, anything larger than that a class should be used instead. Even with the given example it is also shown that a single line of code does the same thing as the class just put into a struct.
Customer = Struct.new(:name, :email)
So that is quite a bit less code than a class. Plus the attributes of a structure are already set up as an attr_accessor so you can quickly and easily change them using Customer["name"] = newName or Customer[:email] = newEmail.