Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial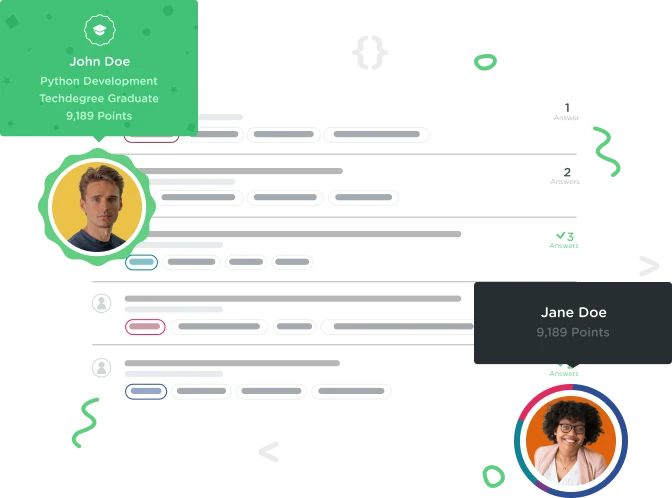

Sebastian Akerman
1,572 PointsSo what if the lowNumber entered is higher than the highNumber? How do we adjust this in our code?
This would result in the following console.log statement
Ie) 5 is a number between 1 and 1.
How do we correct this to add a message like "Your low number is higher than your high number, please adjust and try again"...? Thanks for helping :D
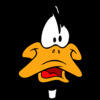
Jason Rich
Front End Web Development Techdegree Student 10,048 PointsHere's how I made that check.
const userNumber1 = prompt(`Can I get a number please?`);
const userNumber2 = prompt(`And a second, larger number?`);
const lowNumber = parseInt(userNumber1);
const highNumber = parseInt(userNumber2);
if (lowNumber < highNumber) {
const random = Math.floor(Math.random() * (highNumber - lowNumber + 1) + lowNumber);
console.log(`${random} is a random number between ${lowNumber} and ${highNumber}.`);
} else if (lowNumber > highNumber) {
console.log(`Please provide a larger second number. Try again.`);
} else {
console.log(`Please provide a number. Try again.`)
}
1 Answer
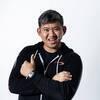
Sean Do
11,933 PointsYou could give an error to the user stating the first number needs to be lower than the second number, but I think that would be a bad user experience to force reload. What I did to check after you've validated both numbers are numbers, I do a check between the two numbers which is higher than the other. Then I put the variables according to their equation. Of course, a function is a more advanced topic, but I figured to use it since I was repeating code.
// Collect input from a user
const inputLow = prompt("Please enter a number: ");
const inputHigh = prompt("Please enter another number: ");
function getRandomInt(min, max) {
min = Math.ceil(min);
max = Math.floor(max);
return Math.floor(Math.random() * (max - min + 1));
}
// Convert the input to a number
const lowNumber = parseInt(inputLow);
const highNumber = parseInt(inputHigh);
// Checks
if (lowNumber || highNumber) {
if (lowNumber > highNumber) {
console.log(getRandomInt(highNumber, lowNumber) + ` is a random number between ${highNumber} and ${lowNumber}`);
} else {
console.log(getRandomInt(lowNumber, highNumber) + ` is a random number between ${lowNumber} and ${highNumber}`);
}
} else {
console.log("You need to provide a number. Try again.");
}
Raynauld Minkema
12,832 PointsRaynauld Minkema
12,832 PointsI used this:
if (inputNumberLow >= inputNumberHigh) { console.log('Your second number is not higher than the first! Error!');
Which is probably a bit crude, but it worked for me.