Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial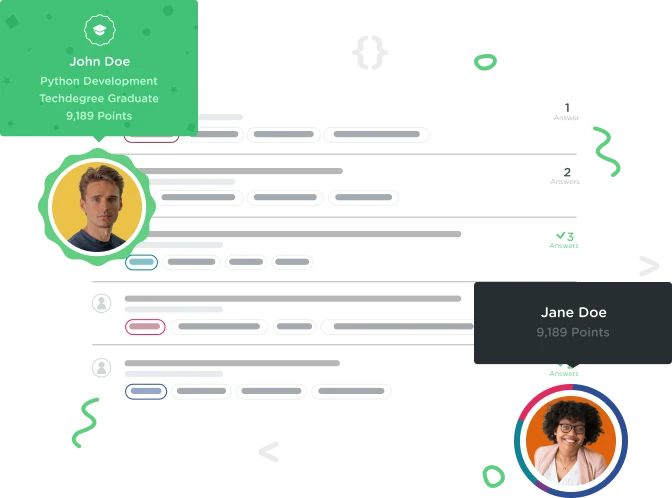

ousmane Harouna Kore
2,698 PointsSoccer League Organizer
Hi I am trying to complete the 2nd project but I am stuck with this issues : -I am not able to add more than 1 team -Whenever I create a new team the menu loop 1 extra time and "default case execute " -My Height report method is not working properly even when I choose player for the 3 ranges only two ranges are printing with sometimes player I didn't choose Thanks
package com.teamtreehouse;
import java.io.IOException;
import java.util.*;
import com.teamtreehouse.model.Player;
import com.teamtreehouse.model.Team;
public class TeamBuilder{
private Team team;
private Map<String,String>menu;
Scanner scan= new Scanner(System.in);
public TeamBuilder(){
menu= new LinkedHashMap<String,String>();
menu.put("Create "," create a new team");
menu.put("Add "," add players to a team");
menu.put("Remove "," remove players from a team");
menu.put("HR "," print height report");
menu.put("ER "," print balance report");
menu.put("Roster "," print roster");
menu.put("Quit "," exit the program");
}
//display menu
public String promptForChoice()throws IOException{
System.out.println("******************************** \n");
for(Map.Entry<String,String>choice: menu.entrySet()){
System.out.printf("%s-%s %n",
choice.getKey(),choice.getValue() );}
System.out.println("********************************");
String input = scan.nextLine();
return input.trim().toLowerCase();
}
//choose a an operation to be performed on the team
public void chooseOption()throws IOException {
String input="";
do{
try{
input=promptForChoice();}catch(IOException ioe){
throw new IOException();
}
switch(input){
case "create":
Team newTeam=promptForTeamInfo();
createTeam(newTeam);
break;
case "add":
Team addToTeam= DisplayTeamList();
addPlayerToTeam(addToTeam);
break;
case "remove":
Team removeFromTeam= DisplayTeamList();
removePlayerFromTeam(removeFromTeam);
break;
case "hr":
Team.heightReport();
break;
case "er":
Team.balanceReport();
break;
case "roster":
Team.printRoster();
break;
case "quit":
System.out.println("Thanks! See ya");
break;
default:
System.out.printf("non valid choice: '%s'.Try again.%n",input);
break;
}
}while(!input.equals("quit"));
}
//prompt for team and coach names
private Team promptForTeamInfo()throws IOException{
System.out.printf("Please enter the team name: ");
String teamName= scan.next();
System.out.printf("Please enter the coach name: ");
String coach= scan.next();
return new Team(teamName,coach);
}
//create a team
public static void createTeam(Team team){
Team.teams.add(team);
System.out.printf("team created%n");
}
//display teams'list
private Team DisplayTeamList()throws IOException{
System.out.println("available teams:");
int index =promptForTeamIndex(Team.teams);
return Team.teams.get(index);
}
//add player to a team
private void addPlayerToTeam(Team team)throws IOException{
Set<Player>playersPool= new TreeSet<>(Team.loadPlayers);
int index = promptForPlayerIndex(playersPool);
if(team.mPlayers.contains(Team.loadPlayers.get(index))){
System.out.printf("The player is already in the team: %s %n ",team);
}
team.mPlayers.add(Team.loadPlayers.get(index));
System.out.printf("The player is added to the team %s%n",team);
}
//remove a player from a team
private void removePlayerFromTeam(Team team)throws IOException{
Set<Player>playersPool= new TreeSet<>(Team.loadPlayers);
int index = promptForPlayerIndex(playersPool);
if(team.mPlayers.contains(Team.loadPlayers.get(index))){
team.mPlayers.remove(Team.loadPlayers.get(index));
System.out.printf("The player is removed from the team %s%n",team);
}else{
System.out.printf("The player is not in the team: %s %n ",team);
}
}
private int promptForTeamIndex(List<Team>choices)throws IOException{
int count=1;
for(Team choice:choices){
System.out.printf("%d.) %s%n",count,choice);
count++;
}
//get the user choice
String makeChoice=scan.nextLine();
int selected=Integer.parseInt(makeChoice.trim());
return selected-1;
}
private int promptForPlayerIndex(Set<Player>selection)throws IOException{
int count=1;
for(Player choice:selection){
System.out.printf("%d.) %s%n",count,choice);
count++;
}
String makeChoice=scan.nextLine();
int selected=Integer.parseInt(makeChoice.trim());
return selected-1;
}
}
package com.teamtreehouse.model;
import com.teamtreehouse.TeamBuilder;
import java.util.*;
import java.io.Serializable;
public class Team implements Comparable<Team>, Serializable{
private static final long serialVersionUID = 1L;
private String mTeamName;
private String mCoach;
public static Set<Player>mPlayers;
public static List<Team>teams;
public static List<Player>loadPlayers=new ArrayList<Player>(Arrays.asList(Players.load()));
//add constructor and initialize variables
public Team(String teamName,String coach){
mTeamName=teamName;
mCoach=coach;
teams= new ArrayList<Team>();
mPlayers = new TreeSet<Player>();
}
public String getTeamName(){
return mTeamName;
}
public String getCoach(){
return mCoach;
}
public static void heightReport(){
Map<String,List<Player>>heightRep=new TreeMap<>();
List<Player>playRep=new ArrayList<>();
playRep.addAll(mPlayers);
for(Player play:playRep){
if(play.getHeightInInches()>=35 && play.getHeightInInches()<=40){
List<Player>range1=heightRep.get("35-40");
if(range1==null){
range1=new ArrayList<>();
heightRep.put("35-40",range1);
}
range1.add(play);
}
if(play.getHeightInInches()>=41 && play.getHeightInInches()<=46){
List<Player>range2=heightRep.get("41-46");
if(range2==null){
range2=new ArrayList<>();
heightRep.put("41-46",range2);
}
range2.add(play);
}
if(play.getHeightInInches()>=47 && play.getHeightInInches()<=50){
List<Player>range3=heightRep.get("47-50");
if(range3==null){
range3=new ArrayList<>();
heightRep.put("47-50",range3);
}
range3.add(play);
}
}
for(Map.Entry<String,List<Player>>display:heightRep.entrySet()){
System.out.printf("%s - %s %n",
display.getKey(),
display.getValue());
}
}
public static void balanceReport(){
Map<String,List<Player>>ExperienceRep=new TreeMap<>();
List<Player>playExp=new ArrayList<>();
playExp.addAll(mPlayers);
for(Player play:playExp){
if(play.isPreviousExperience()){
List<Player> exp=ExperienceRep.get("Experienced");
if(exp==null){
exp = new ArrayList<>();
ExperienceRep.put("Experienced",exp);
}
exp.add(play);
}else{
List<Player> inexp=ExperienceRep.get("Inexperienced");
if(inexp==null){
inexp=new ArrayList<>();
ExperienceRep.put("Inexperienced",inexp);
}
inexp.add(play);
}
}
for(Map.Entry<String,List<Player>>display:ExperienceRep.entrySet()){
System.out.printf("%s - %s %n",
display.getKey(),
display.getValue());
}
}
public static void printRoster(){
for(Player printplayer: mPlayers){
System.out.printf("%s %s%n",printplayer.getLastName(),printplayer.getFirstName());
}
}
@Override
public int compareTo(Team other) {
if(equals(other)){
return 0;
}
return mTeamName.compareTo(other.mTeamName);
}
@Override
public String toString(){
return String.format("%s coached by: %s",mTeamName,mCoach);
}
}
1 Answer
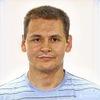
Alexander Nikiforov
Java Web Development Techdegree Graduate 22,175 PointsThe problem is the way you use
public static Set<Player>mPlayers;
public static List<Team>teams;
public static List<Player>loadPlayers=new ArrayList<Player>(Arrays.asList(Players.load()));
Don't try to use public static
. Static means it is loaded with the class, so you cannot change Team.teams
. Or may be you can, but you make things overcomplicated
It is a VERY BAD idea to use Teams
inside Team
.
Yes there is a Design Pattern for that, it is called Composite But it is not the place to Stick it.
Please follow the structure of the application as close as possible to KaraokeMachine
by Craig Dennis.
Song is like Player
Team is like SongBook
.
Does it make sense ?
And PUT Teams
as a private List<Team> teams
in a TeamBuilder
. As he did with SongBook
PS. Use Slack
Please use Slack for Techdegree Project related questions.
Why? out of 300 000 or so students in Treehouse, only 300 has access to Techdegree Project you are in, so you may not find help in a Community ...
But the more often you ask in Slack, the more you get to know people there and faster you get help
Especially with later Android Projects.
Good Luck!
ousmane Harouna Kore
2,698 Pointsousmane Harouna Kore
2,698 PointsHi Alexander Thanks for the help