Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial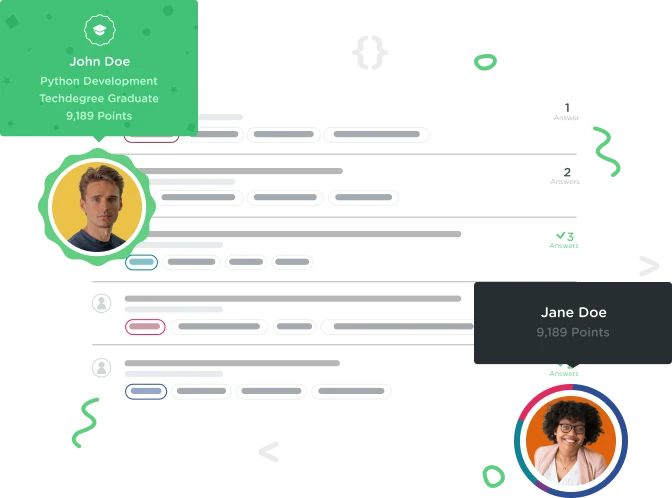

crystaldarin
7,046 PointsSolution achieved! Feedback please.
var answers = '';
var correctQuestions = [];
var wrongQuestions = [];
//Questions
var questions = [
['What is my first name?','crystal'],
['What is my favorite color?','orange'],
['Where do I live?','rhode island']
];
//Ask questions
for(var i = 0; i < questions.length; i += 1){
answers = prompt(questions[i][0]);
answers = answers.toLowerCase();
//Test answers
if(answers === questions[i][1]){
alert('Yeah you got the answer right!');
correctQuestions.push(questions[i][0]);
}
else{
alert('Sorry you got the answer wrong...');
wrongQuestions.push(questions[i][0]);
}
}
//Display correct questions
if(correctQuestions.length > 0 ){
var html = '<h2>Questions I got Right</h2> <ol>';
for(var i = 0; i < correctQuestions.length; i += 1){
html += '<li>' + correctQuestions[i] + '</li>';
}
html += '</ol>';
document.write(html);
}
//Display wrong questions
if(wrongQuestions.length > 0){
var html = '<h2>Questions I got Wrong</h2> <ol>';
for(var i = 0; i < wrongQuestions.length; i += 1){
html += '<li>' + wrongQuestions[i] + '</li>';
}
html += '</ol>';
document.write(html);
}

Ashi A
2,531 PointsCrystal - Love the use of arrays to track correctQuestions and incorrectQuestions. Also love that you pop an alert when they get answers right or wrong. That's a nice touch.
Edwin Carbajal
4,133 PointsEdwin Carbajal
4,133 PointsHi Crystal,
Great Job! You even added your own response to the user based on their response! My only feedback would be to wrap each individual task in its own function. This way it eliminates floating for loops and if statements and makes your code more neat. But other than that, keep up the good work! :)