Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial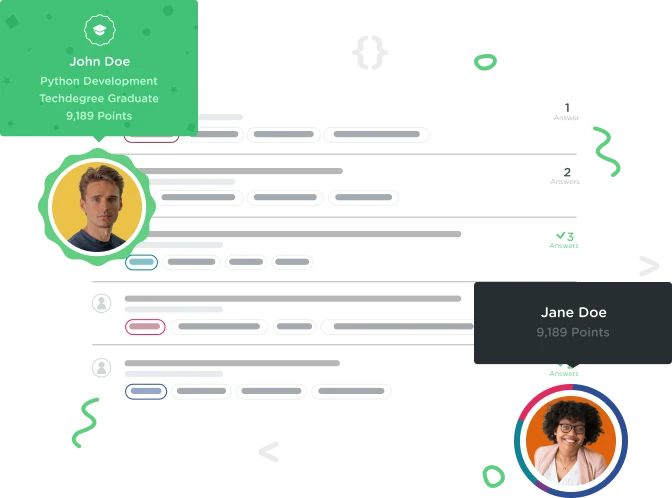
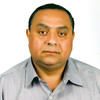
Mohsen Qaddoura
22,237 PointsSolution for either non-existent student or duplicate name
var message = '';
var student;
var search;
student_exist = false; //Setting up a flag
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
student.name.toLowerCase();
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('To search for a student : Enter name [Jody]; to exit enter quit');
if (search === null || search.toLowerCase() === 'quit') {
message = "";
print(message);
break;
}
message = "";
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
message += getStudentReport( student );
print(message);
student_exist = true; //Student found raising the flag
} //end of if statement
} // end of for loop
if (!(student_exist)) {
message = "No student in class by the name you entered.";
print(message);
}
student_exist = false; // resetting the flag for next search
} // end of while loop
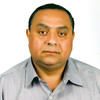
Mohsen Qaddoura
22,237 PointsThank you Samvel Tadevosyan, your remark about moving of the printing the message variable outside the loop is spot on. As for lowering the case of the search entry and the student name they are necessary for the search success, the user entry ( quit or name be the in lower case or uppercase! The print out comes in the proper capitalization as it is in Students. For the case of empty message it will only happen if there is no student matching the search entry which is already dealt with in the code. As for the message = "" inside the if statement before the break I added it to clean the page off the last search, a matter of personal taste :) , not a requirement though! Best of success for all my colleagues in TreeHouse.
Here is a modified and tested version of my code:
var message = '';
var student;
var search;
student_exist = false; //Setting up a flag
function print(message) {
var outputDiv = document.getElementById('output');
outputDiv.innerHTML = message;
}
function getStudentReport(student) {
student.name.toLowerCase();
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
while (true) {
search = prompt('To search for a student : Enter name [Jody]; to exit enter quit');
if (search === null || search.toLowerCase() === 'quit') {
message = "";
print(message);
break;
}
message = ""; // clearing last search result
for (var i = 0; i < students.length; i += 1) {
student = students[i];
if (student.name.toLowerCase() === search.toLowerCase() ) {
message += getStudentReport( student );
student_exist = true; //Student found raising the flag
} //end of if statement
} // end of for loop
print(message);// printing out the result of search
// checking if search did not find the search entry in students
if (!(student_exist)) {
message = "No student in class by the name you entered.";
print(message);
}
student_exist = false; // resetting the flag for next search
} // end of while loop

Samvel Tadevosyan
7,117 PointsMohsen,
I was not speaking about the var search for the lower case issue. You have such function:
function getStudentReport(student) {
student.name.toLowerCase();
var report = '<h2>Student: ' + student.name + '</h2>';
report += '<p>Track: ' + student.track + '</p>';
report += '<p>Points: ' + student.points + '</p>';
report += '<p>Achievements: ' + student.achievements + '</p>';
return report;
}
I am sorry, I did not get your point for this line: student.name.toLowerCase(); As I see it has no role. Just remove it and run your code, the results should be the same .
In this chunk of code:
print(message);
if (!(student_exist)) {
message = "No student in class by the name you entered.";
print(message);
}
you can do the same with 1 print(message), that will look like this:
if (!(student_exist)) {
message = "No student in class by the name you entered.";
}
print(message);
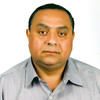
Mohsen Qaddoura
22,237 PointsSamvel Tadevosyan, you are right .
Thank you once more , Mohsen
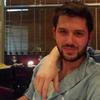
Nir Frank
3,578 PointsHi Mohsen Qaddoura , I'm trying to figure out a solution to the duplicate student name. Would you mind explaining what you did here exactly?
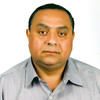
Mohsen Qaddoura
22,237 PointsNIr Frank, the solution for the duplicate name was straight forward. It only needed a tiny modification that is easy to miss; ( "+=" instead of "=").
In the if statement {} inside the for loop you'll find this assignment statement :
message += getStudentReport( student );
which is equivalent to:
message = message + getStudentReport( student );
meaning it keeps adding similar names , after checking them with if statement condition, instead of replacing the name , by reassigning, as it did before without the plus sign. I hope this explanation was clear enough .
Regards, Mohsen
1 Answer
Mark Glissmann
7,225 PointsI found it is a little more intuitive to place the
message = ""; // clearing last search result
line at the end of the while-loop when you reset student_exist to false. This way the variables that are being reset are grouped together.
Thanks for the help, Mark
Samvel Tadevosyan
7,117 PointsSamvel Tadevosyan
7,117 PointsThe code works but I see some little problems in it.
If you have student_n with name Jody and student_k (n < k) with the name Jody, your code prints student_n, then when loop gets to student_k, it prints again student_n and this 2nd time it prints student_k as well and you do not notice visually what happens. Imagine you have 5000 students and 80 of them are Jody, so you will print 79 times before printing the final list... That is why I think print(message) should be outside the loop.
The boolean student_exist is ok but I would prefer it with checking if the message is empty and alert about it or print as you wished.
No need to make lower case the name of the student before printing, in the function getStudentReport(student)
The line message = "" inside the if statement before the break isn't needed as well.
I'd also suggest use blank lines in your code more frequently to make the code more readable.
Thanks, Sam