Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial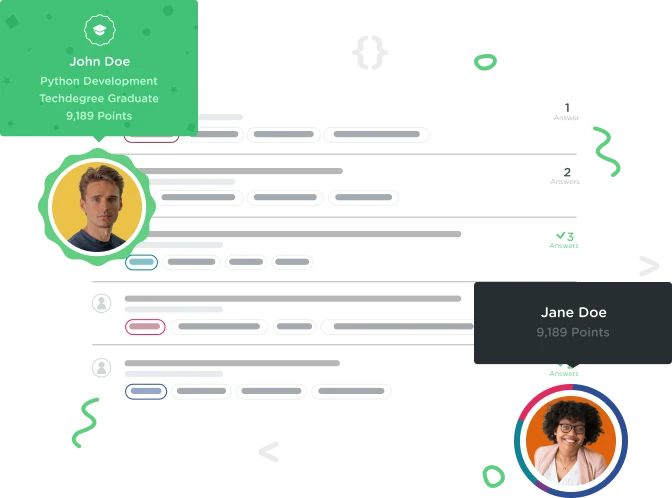

Thomas Cunningham
3,941 PointsSolution for Word Length?
Hi,
Could anyone explain to me why this code doesn't pass the Word Length challenge? It seems to work if I replace the count variable with a number but as it is, it just returns an empty list... Do escape counts not take variables?
Thanks very much!
import re
# EXAMPLE:
# >>> find_words(4, "dog, cat, baby, balloon, me")
# ['baby', 'balloon']
def find_words(count, data):
return re.findall(r"\b\w{count,}\b", data)
5 Answers
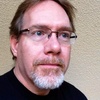
Chris Freeman
Treehouse Moderator 68,441 PointsAs you have it in your code, "count" is being interpreted as a string of 5 characters instead of a variable count
.
One way is to concatenate count
into the regex:
def find_words(count, data):
return re.findall(r"\b\w{"+str(count)+r",}\b", data)
Or use the format method:
def find_words(count, data):
return re.findall(r"\b\w{{{count},}}\b".format(count=count), data)
Note a double curly bracket is used to escape a {
and }
from being interpreted by the format
method as a field marker.
By comparison, here is the old style formatting: Or use the format method:
def find_words(count, data):
return re.findall(r"\b\w{%s,}\b" % count, data)
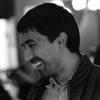
Martin Cornejo Saavedra
18,132 PointsI don't think this solution is beautiful but it worked for me:
def find_words(count, data):
word = '\w'*count
return re.findall(r"\b{}+\b".format(word), data)
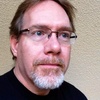
Chris Freeman
Treehouse Moderator 68,441 PointsThis works because it repeats the \w
term count
times, with the last one being followed by a +
to capture the "or more" counts. for count = 4
. this produces:
return re.findall(r'\b\w\w\w\w+\b'.format(word), data)

Than Win Hline
1,679 Pointscan you please explain why did you use {"+str(count)+r",} ? i dont understand why we are using + before and after str(count)
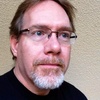
Chris Freeman
Treehouse Moderator 68,441 PointsFirst, look at the whole regex:
r"\b\w{"+str(count)+r",}\b"
# 3 parts
r"\b\w{" # 1
str(count) #2
r",}\b" #3
The first and last are raw strings. The second creates a string from the variable count
. Using the + concatenates the three strings into one string.
Post back if you need more help. Good luck!!!

Than Win Hline
1,679 PointsThank you for explaining nicely.

Than Win Hline
1,679 Pointscan you explain this ",}\b"? why are we using a comma. I thought we have to do something like return re.findall(r"\b\w{"+str(count)+r"}+\b", data) since '+' is used for one or more occurances.
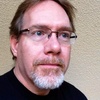
Chris Freeman
Treehouse Moderator 68,441 PointsUsing a + following a repeat { } is invalid syntax and raises a βmultiple repeatβ error
Thomas Cunningham
3,941 PointsThomas Cunningham
3,941 PointsGreat, thanks!
Than Win Hline
1,679 PointsThan Win Hline
1,679 PointsCan you please explain the format method a little bit more? I don't understand why we have to use double bracket around 'count' and why we need to assign count=count.