Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial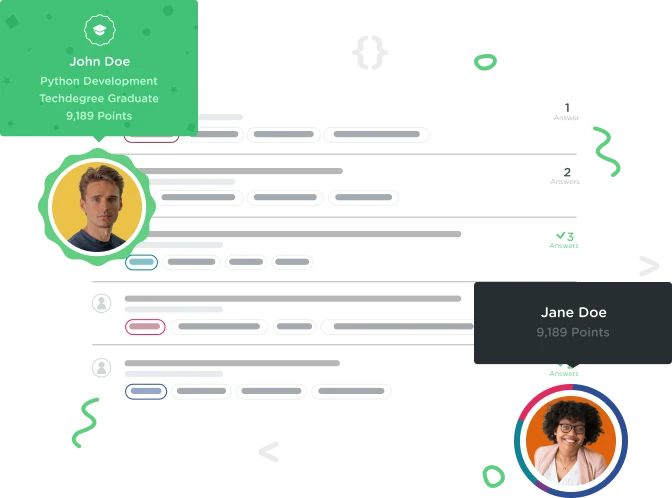

Abhishek Dahiya
3,380 Pointssolution please i am really having a hard time understanding functions...
After your newly created returnValue function, create a new variable named echo. Set the value of echo to be the results from calling the returnValue function. When you call the returnValue function, make sure to pass in any string you'd like for the parameter.
The function will accept the string you pass to it and return it back (using the return statement). Then your echo variable will be set with what is returned, the results.
function returnValue (upper) {
var randomNumber = Math.floor(Math.random() * upper) + 1;
return upper;
}
<!DOCTYPE HTML>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>JavaScript Basics</title>
</head>
<body>
<script src="script.js"></script>
</body>
</html>
2 Answers
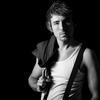
Jacek Grzegorczyk
8,974 PointsHey there. If you are having troubles with understanding the functions i suggest you make a little practice with even simpler examples so you can really "feel it".
Also when writing programs try to decompose it step by step In this particular example:
1.Set the value of echo to be the results from calling the returnValue function. var echo = returnValue(); 2.When you call the returnValue function, make sure to pass in any string you'd like for the parameter. var echo = returnValue(5); //here we are setting upper argument to 5;
- If you want to check the result you can use console.log console.log(echo);
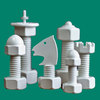
Steven Parker
230,688 PointsIt looks like you started to build something for a different challenge. Your function computes a random number, but then doesn't use or return it. It still passes the challenge because it returns the argument, but that calculation should not be there. That line should be removed and the function shortened to this:
function returnValue (upper) {
return upper;
}
Then, for step 2 you just need to assign a variable named "echo" to the result of calling that function on a string variable. Leave the function as is, and add something like this below it:
var echo = returnValue("this is my test string");
Note that what is in the string is your choice, but the argument should be a string (something enclosed in quotes).