Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial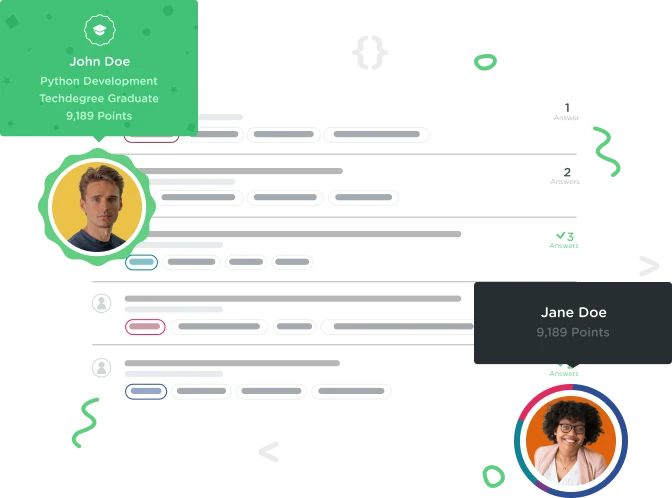
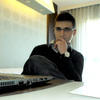
Daniel Nitu
13,272 PointsSolution to command line weather app using openweathermap.org API
Hi guys, I want to share the weather app I made using openweathermap.org. Please let me know if there is anything I could improve. I used the city name instead of a zip code so you can type in any city name you like.
//Use openweathermap.org API to get weather forecast by city name
var http = require('http');
//Print out message
function printMessage(city, description, temperature, wind, clouds){
var message = 'Currently, ' + city + ' has ' + description +
', a temperature of ' + temperature + ' degrees Celsius' +
', a wind speed of ' + wind +
' m/s and clouds covering ' + clouds + '% of the sky.';
console.log(message);
}
//Print out error
function printError(error){
console.error(error.message);
}
function getWeather(city){
var key = 'your API key';
var linkByCityName = 'http://api.openweathermap.org/data/2.5/weather?q=';
//Connect to the API
var request = http.get(linkByCityName + city + '&units=metric&APPID=' + key, function(response){
var body = '';
//Read data
response.on('data', function(chunk){
body += chunk;
});
response.on('end', function(){
//Parse data
var weather = JSON.parse(body);
printMessage(weather.name,
weather.weather[0].description,
weather.main.temp.toFixed(1),
weather.wind.speed,
weather.clouds.all);
});
});
request.on('error', printError);
}
var city = process.argv.slice(2);
getWeather(city);
Input looks like this:
treehouse:~/workspace$ node weather bucharest
Output looks like this, but it can me modified to show a lot of other weather characteristics:
Currently, Bucharest has clear sky, a temperature of 35.4 degrees Celsius, a wind speed of 2.1 m/s and clouds covering 0% of the sky.