Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial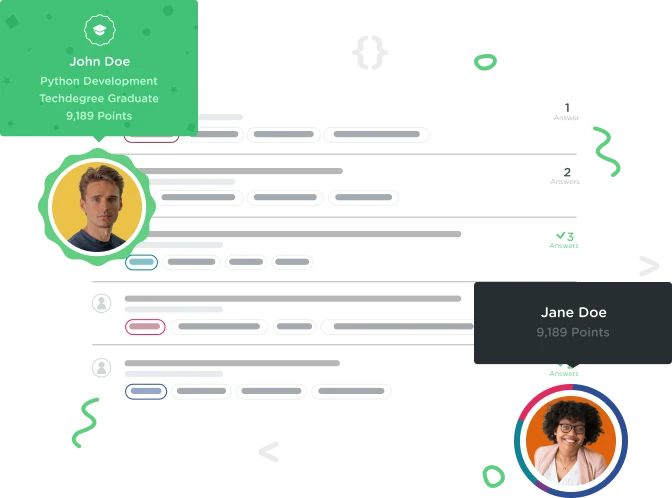

Chris Mitts
855 PointsSolution to Task 2 nullifies Task 1
I am attempting to solve this objective by turning my Discount Code string into a char array and checking each character in that char array. If the character in the char array is not a letter or $ then I throw the exception. When I check my work I get the error that now my Task 1 is no longer working and I am not sure why. Thank you for your help
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
private String normalizeDiscountCode(String discountCode) {
for (char letter : discountCode.toCharArray()) {
if ( letter != '$' || ! Character.isLetter(letter)) {
throw new IllegalArgumentException("Invalid discount code.");
}
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
public class Example {
public static void main(String[] args) {
// This is here just for example use cases.
Order order = new Order(
"Yoda PEZ Dispenser",
600);
// These are valid. They are letters and the $ character only
order.applyDiscountCode("abc");
order.getDiscountCode(); // ABC
order.applyDiscountCode("$ale");
order.getDiscountCode(); // $ALE
try {
// This will throw an exception because it contains numbers
order.applyDiscountCode("ABC123");
} catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
try {
// This will throw as well, because it contains a symbol.
order.applyDiscountCode("w@w");
}catch (IllegalArgumentException iae) {
System.out.println(iae.getMessage()); // Prints "Invalid discount code"
}
}
}
1 Answer

andren
28,558 PointsThe problem is that your code raises an exception for discount codes that are valid. In fact it actually raises an exception for all discount codes, and that's why task 1 is no longer passing.
You check if a character is not the $ symbol OR
not a letter, that means that an exception will be raised if either of those conditions are true. Let's say the character in question was 'A' that is a letter so the second condition would be false, but 'A' is not the $ symbol so the first condition would be true, which means that an exception would be thrown. Even though 'A' is a valid letter for the discount code.
The same type of thing would happen if the character was $, since that is not a letter.
The easiest way around this is to replace || (OR
) with && (AND
), that way an exception is only thrown if the character is both not a letter AND
not the $ symbol. Like this:
public class Order {
private String itemName;
private int priceInCents;
private String discountCode;
public Order(String itemName, int priceInCents) {
this.itemName = itemName;
this.priceInCents = priceInCents;
}
public String getItemName() {
return itemName;
}
public int getPriceInCents() {
return priceInCents;
}
public String getDiscountCode() {
return discountCode;
}
private String normalizeDiscountCode(String discountCode) {
for (char letter : discountCode.toCharArray()) {
if ( letter != '$' && ! Character.isLetter(letter)) {
throw new IllegalArgumentException("Invalid discount code.");
}
}
discountCode = discountCode.toUpperCase();
return discountCode;
}
public void applyDiscountCode(String discountCode) {
this.discountCode = normalizeDiscountCode(discountCode);
}
}
Edit: Changed my wording a bit.
Chris Mitts
855 PointsChris Mitts
855 PointsAppreciate the help, got my logic confused there, thank you!