Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial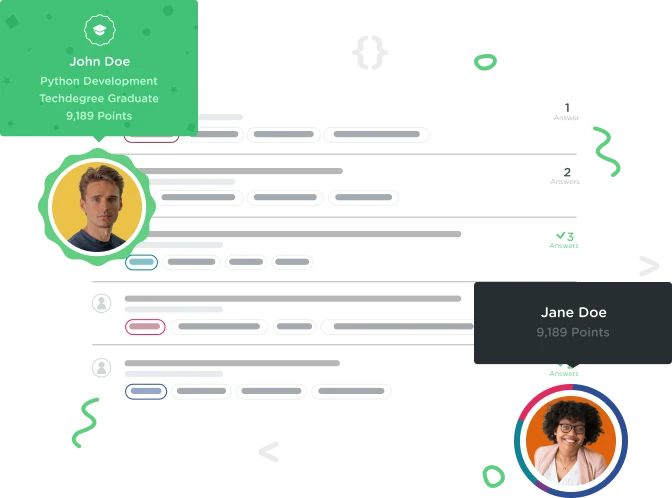

Michael Ott
3,149 Pointssolution to the challenge following Methods: Part 2.
The challenge asks: Sort the 'saying2' array, on about line 19, so that the words are listed in length order. The shortest first. Use the 'length' property on the strings in the sort function.
Below is the array: var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
<!DOCTYPE html>
<html lang="en">
<head>
<title> JavaScript Foundations: Arrays</title>
<style>
html {
background: #FAFAFA;
font-family: sans-serif;
}
</style>
</head>
<body>
<h1>JavaScript Foundations</h1>
<h2>Arrays: Methods Part 1</h2>
<script>
var saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse();
saying2;
</script>
</body>
</html>
3 Answers
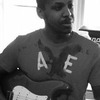
Samuel Webb
25,370 PointsLet's take this array:
var saying2 = [5, 4, 3, 2, 1, 0];
What happens when you pass in that "a - b" function is that a is equal to one of the numbers in the array, and b is equal to the next. Then you subtract a - b and either get a positive number or a negative number. 5 - 4 = +1 which means 4 is a lower number than 5. So it puts 4 ahead of 5. Then you can compare 5 - 3 = +2 which means 3 is lower and it goes before 5. The numbers do this until they get into an order where subtracting one number by the next number will equal a negative number. Which at that point would mean they have been sorted lowest to highest. That's basically what that function says.
saying2.sort(function(a,b){return a - b}; // for lowest to highest
To sum it up. First you have saying2.sort(). The sort() method can take an optional anonymous function to decide how the array is sorted. In that array, you have to pass in 2 instance variables to be compared. Those will go through the a and b variables. Once in the code block (between the curly braces) you decide how the 2 variables will be compared to each other. We're doing {return a - b}. So based on the way the sort() method works, this means it will keep comparing variables(numbers passed in from the saying2 array) passed into it until subtracting a number on the right from a number on the left always produces a negative number. Then it will return the array in the order it produced. Which will be lowest number to highest number.
I hope I explained that well enough for you as it is an interesting concept to try and explain. If you'd like to see more on this, check out ,W3Schools.
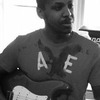
Samuel Webb
25,370 PointsWhen you use the 'length' property on a string, it returns a number which you then want to compare in an anonymous function, inside of your sort() function. Here's the code:
<script>
var saying1 = ["The", "quick", "brown", "fox", "jumps", "over", "the", "lazy", "dog"];
var saying2 = ["The", "quick", "brown", "fox", "jumped", "over", "the", "lazy", "dog's", "back"];
saying1.reverse();
saying2.sort(function(a, b){
return a.length - b.length
});
</script>

Michael Ott
3,149 PointsSamuel- Thanks for the message and the explanation!
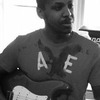
Samuel Webb
25,370 PointsNo problem.
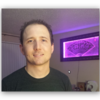
Brian van Vlymen
12,637 PointsHello, Samuel Webb, Can you please explained to me better example please. One mostly I dont really understand why should we put "return a - b" however I completely understand ASCII or Unicode how the sort or length method work. let don't worry about the "length" method.
var saying2= [5,4,3,2,1,0]
If saying2.sort(function(a, b){
return a - b;
});
console.log (saying2);
the answer would be 0,1,2,3,4,5 because of return is less than 0, sort a to a lower index than b, i.e. a comes first?
I am confused why required put "a - b" to make it work?
Brian van Vlymen
12,637 PointsBrian van Vlymen
12,637 Pointswow thank you so much for explained how the function works. thumb up I understood! again thank you !
Samuel Webb
25,370 PointsSamuel Webb
25,370 PointsGlad I could help.