Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial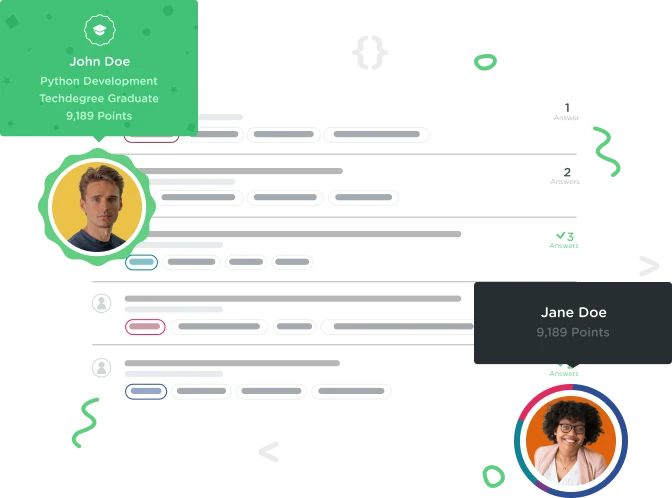
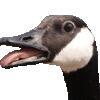
wildgoosestudent
11,274 PointsSolution without using zip codes, Openweather
Just thought I'd post my solution in case it might be helpful for someone else. This was a pretty tricky section for me and took me a while to understand what was going on.
//Require https modules
const https = require('https');
const http = require('http');
const api = require('./api.json')
// this allows the const parameters to be turned into a format that the URL can understand
const querystring = require('querystring');
function get(query) {
const parameters = {
// query is the name of the city entered in the console
// I'm not American so I've left out the zip code bit
q: query,
APPID: api.key,
units: 'metric'
};
// takes the parameters above and puts them in the URL to get the correct API fields
const url = `https://api.openweathermap.org/data/2.5/weather?${querystring.stringify(parameters)}`
// url looks like: https://api.openweathermap.org/data/2.5/weather?q=prague&APPID=********************************&units=metric
// **** = my api.key that I'll be keeping secret ;)
const request = https.get(url, response => {
let body = "";
response.on('data', data => {
body += data.toString();
})
response.on('end', () => {
const weather = JSON.parse(body);
printWeather(weather);
})
})
}
// print out the temperature in Celcius
function printWeather(weather) {
const message = `Current weather in ${weather.name}, ${weather.sys.country} is ${weather.main.temp}C, but feels like ${weather.main.feels_like} C`
console.log(message);
}
module.exports.get = get
In the console
node app.js prague
would print:
Current weather in Prague, CZ is 4.17C, but feels like 1.1 C