Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial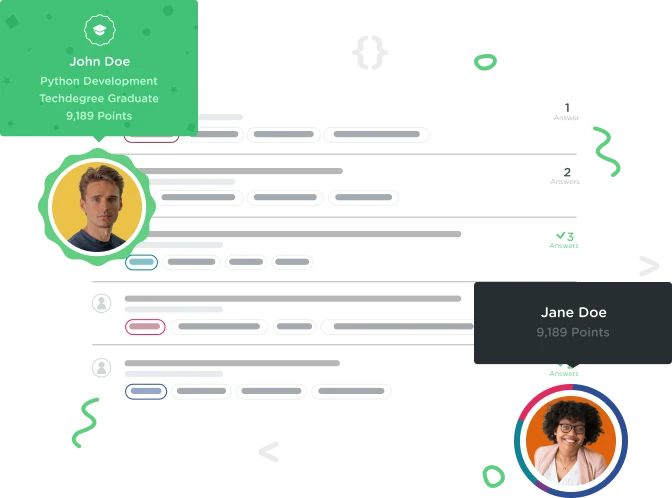

Codrin Postu
8,022 Points[Solved] For me the Expected field doesn't work. I get the "cannot find symbol" error
Did anyone else encounter this?

Codrin Postu
8,022 PointsLauren Moineau here is the code. It is exactly the code from the video.
package com.teamtreehouse.vending;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class AlphaNumericChooserTest {
private AlphaNumericChooser chooser;
@BeforeEach
void setUp() {
chooser = new AlphaNumericChooser(26, 10);
}
@Test
void validInputReturnsProperLocation() throws Exception {
AlphaNumericChooser.Location loc = chooser.locationFromInput("B4");
assertEquals(1, loc.getRow());
assertEquals(3, loc.getColumn());
}
@Test (expected = InvalidLocationException.class)
void choosingWrongInputIsNotAllowed() throws Exception {
chooser.locationFromInput("WRONG");
}
}

Lauren Moineau
9,483 PointsThanks
3 Answers

Miguel Nunez
1,081 PointsOh, wow, it's a lambda! Cool.

Raymond Cool
5,171 PointsThis discussion was very helpful. UPVOTE

Lauren Moineau
9,483 PointsThanks for posting your code Codrin. I haven't done that course yet but if I'm not mistaken I think JUnit 4 is used. In your code, your imports are for JUnit 5 Jupiter. It should be:
import org.junit.Before;
import org.junit.Test;
import static org.junit.Assert.*;
Hope that helps :)

Codrin Postu
8,022 PointsYes I did realize I was using JUnit 5 but didn't think it would be too different from 4. Apparently the new version doesn't use "expected" and "rule" anymore.
For anyone else having this issue this is the solution I have found other than using JUnit 4:
package com.teamtreehouse.vending;
import org.junit.jupiter.api.BeforeEach;
import org.junit.jupiter.api.Test;
import static org.junit.jupiter.api.Assertions.*;
class AlphaNumericChooserTest {
private AlphaNumericChooser chooser;
private Throwable thrown;
@BeforeEach
void setUp() {
chooser = new AlphaNumericChooser(26, 10);
}
@Test
void validInputReturnsProperLocation() throws Exception {
AlphaNumericChooser.Location loc = chooser.locationFromInput("B4");
assertEquals(1, loc.getRow());
assertEquals(3, loc.getColumn());
}
// The solution for the issue I had
@Test
public void choosingWrongInputIsNotAllowed() {
thrown = assertThrows(InvalidLocationException.class, () -> chooser.locationFromInput("WRONG"));
}
}

Lauren Moineau
9,483 PointsThanks Codrin. I haven't really looked into migration to JUnit 5 yet but I know some changes to the code are expected. I'm glad you sorted it out and thanks for posting your solution here. I'm sure it will help other students :)
Lauren Moineau
9,483 PointsLauren Moineau
9,483 PointsHi Codrin. Could you copy/paste your code here, please? Without it, it's impossible to help you. Thanks :)