Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial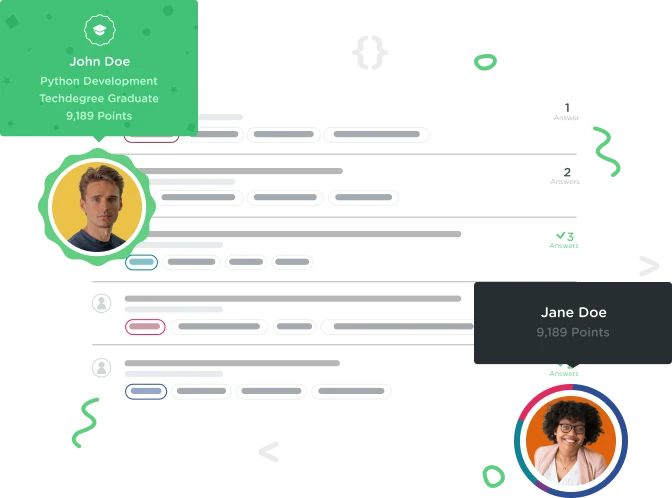

Codrin Postu
8,022 Points[Solved] My app crashes when I change the background color
When I try to change the Background Color my App Builds without errors but when I try to run the app it just crashes. Here is the code.
package com.withur.facttest;
import android.graphics.Color;
import android.support.v7.app.AppCompatActivity;
import android.os.Bundle;
import android.view.View;
import android.widget.Button;
import android.widget.RelativeLayout;
import android.widget.TextView;
public class MainActivity extends AppCompatActivity {
// Declare our View variables
private FactBook factBook = new FactBook();
private RelativeLayout relativeLayout;
private TextView factTextView;
private Button showFactButton;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
relativeLayout = findViewById(R.id.mainLayout);
factTextView = findViewById(R.id.factTextView);
showFactButton = findViewById(R.id.showFactButton);
View.OnClickListener listener = new View.OnClickListener() {
@Override
public void onClick(View v) {
//Update the screen with a new fact
relativeLayout.setBackgroundColor(Color.RED);
factTextView.setText(factBook.getFact());
}
};
showFactButton.setOnClickListener(listener);
}
}
Edit: The issue was that I changed the layout to Constraint and didn't realize.

Lauren Moineau
9,483 PointsAwesome Codrin Postu ! I'm glad you fixed it :)
1 Answer

Codrin Postu
8,022 PointsOh Lauren Moineau that helped! I was using by accident ConstraintLayout because Relative Layout was throwing everything in the corner for me.
Lauren Moineau
9,483 PointsLauren Moineau
9,483 PointsHi Codrin. Could you copy/paste the error message you get in your logcat please? (using the "show only selected application" filter). Thanks