Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial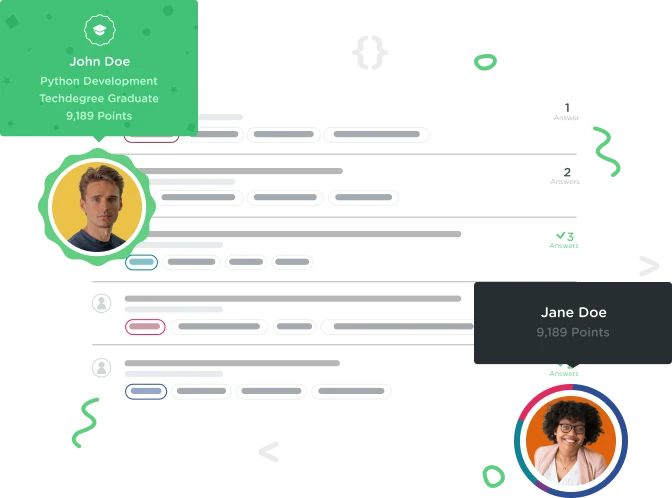

Raul Cisneros
7,319 PointsSolved. Not sure if this is the correct way, but it works.
let newTeachers = [
{
name: 'James',
topicArea: 'Javascript'
},
{
name: 'Treasure',
topicArea: 'Javascript'
}
];
const teachers = [
{
name: 'Ashley',
topicArea: 'Javascript'
}
];
const courses = ['Introducing JavaScript',
'JavaScript Basics',
'JavaScript Loops, Arrays and Objects',
'Getting Started with ES2015',
'JavaScript and the DOM',
'DOM Scripting By Example'];
var i = courses.length;
function addNewTeachers(newTeachers) {
// TODO: write a function that adds new teachers to the teachers array
teachers.push(newTeachers[0]);
teachers.push(newTeachers[1]);
}
function printTreehouseSummary() {
// TODO: fix this function so that it prints the correct number of courses and teachers
for (var i = 0; i < teachers.length; i++) {
console.log(`${teachers[i].name} teaches ${teachers[i].topicArea}`);
}
console.log(`Treehouse has ${courses.length} JavaScript courses, and ${teachers.length} Javascript teachers`);
}
addNewTeachers(newTeachers);
printTreehouseSummary();
3 Answers
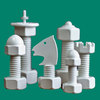
Steven Parker
230,274 PointsIn programming, "it works" is at least 90% of "correct". The rest is about being concise, efficient and readable/maintainable — and from my viewpoint you've covered all the bases. Good job!

Matthew Fung
8,643 PointsWhile this does work and congrats on getting this far, you haven't really used let or const to help you out here.
In the addNewTeachers function; you could make this a bit more DRY (don't repeat yourself). If there were 50 or 100 new Teachers; would you want to type out the same thing over and over with different indexes?
function addNewTeachers(newTeachers) {
// TODO: write a function that adds new teachers to the teachers array
teachers.push(newTeachers[0]);
teachers.push(newTeachers[1]);
}
// Could be iterated; regardless of how many teachers there are.
function addNewTeachers(newTeachers) {
// TODO: write a function that adds new teachers to the teachers array
// ES6 spread operator
teachers.push(...newTeachers);
// ES5 For Loop
for (let i = 0; i < newTeachers.length; i++ ) {
teachers.push(newTeachers[i]);
}
}
A spread operator 'spreads' the arguments into individual arguments. This means you can perform an action on an iterable for each item in that array / object.
The idea in the printTreeHouseSummary fucntion was to limit the scope of the variable 'i' to the for loop; as it's already declared in the global scope. Using 'let' allows us to localise the scope of the varaible to the scope it is used in. This means that we don't overwrite the value of i ever.
Your way does work, but you're not using let or const, which is the point of this task! Otherwise you may as well be writing in ES5!
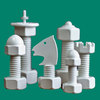
Steven Parker
230,274 PointsClever use of the spread operator! Just be aware that the maximum number of parameters that one function can take is limited in practice, and may vary with different JavaScript engines. See the MDN page for apply() for more details.

Steve Gallant
14,943 PointsI would suggest this is "a" correct way, because it does work, however what if there were 50 new teachers? Try adding a for loop to iterate over the newTeachers array and push each object into the teachers array.
Steven Parker
230,274 PointsSteven Parker
230,274 PointsFor only two items, a loop would not make the code more compact. But Steve and Matthew are right that if there were more teachers, a loop would be a very good idea!
And I totally overlooked that this was for the course "Practice Let and Const in JavaScript" — this would certainly be a good opportunity to refactor the code and replace "var" with "const" where possible and with "let" otherwise.