Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial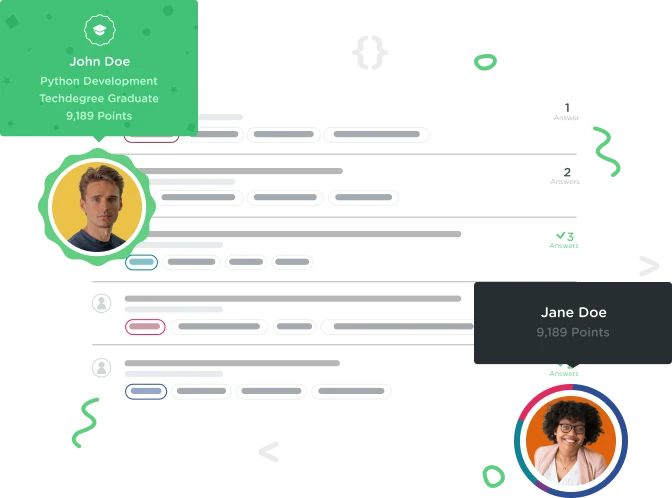
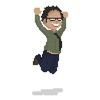
Quintin Marcelino
2,693 Points(SOLVED) NullPointerException: id == null
So I'm having what seems to be a similar problem as other students, but nothing from the other threads is helping. When I run the app, it runs, though the views are still populated with the hard coded data. Then it crashes within 10 seconds. Here's the error: ''' java.lang.NullPointerException: id == null at java.util.TimeZone.getTimeZone(TimeZone.java:328) at digitaltinderbox.com.stormy.CurrentWeather.getFormattedTime(CurrentWeather.java:76) at digitaltinderbox.com.stormy.MainActivity.updateDisplay(MainActivity.java:104) at digitaltinderbox.com.stormy.MainActivity.access$200(MainActivity.java:27) at digitaltinderbox.com.stormy.MainActivity$1$1.run(MainActivity.java:76) at android.os.Handler.handleCallback(Handler.java:733) at android.os.Handler.dispatchMessage(Handler.java:95) at android.os.Looper.loop(Looper.java:136) at android.app.ActivityThread.main(ActivityThread.java:5017) at java.lang.reflect.Method.invokeNative(Native Method) at java.lang.reflect.Method.invoke(Method.java:515) at com.android.internal.os.ZygoteInit$MethodAndArgsCaller.run(ZygoteInit.java:795) at com.android.internal.os.ZygoteInit.main(ZygoteInit.java:611) at dalvik.system.NativeStart.main(Native Method) '''
Here's my Main Activity
''' package digitaltinderbox.com.stormy;
import android.content.Context; import android.net.ConnectivityManager; import android.net.NetworkInfo; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.util.Log; import android.widget.TextView; import android.widget.Toast; import android.widget.ImageView;
import org.json.JSONException; import org.json.JSONObject;
import java.io.IOException;
import butterknife.BindView; import butterknife.ButterKnife; import okhttp3.Call; import okhttp3.Callback; import okhttp3.OkHttpClient; import okhttp3.Request; import okhttp3.Response;
public class MainActivity extends AppCompatActivity {
public static final String TAG = MainActivity.class.getSimpleName();
private CurrentWeather mCurrentWeather;
@BindView(R.id.timeLabel) TextView mTimeLabel;
@BindView(R.id.temperatureLabel) TextView mTemperatureLabel;
@BindView(R.id.humidityValue) TextView mHumidityValue;
@BindView(R.id.precipValue) TextView mPrecipValue;
@BindView(R.id.summaryLabel) TextView mSummaryLabel;
@BindView(R.id.iconImageView) ImageView mIconImageView;
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
ButterKnife.bind(this);
String apiKey = "d2fd58d75b2c25aa8f464fc723c028a2";
double latitude = 37.8267;
double longitude = -122.423;
String forecastUrl = "https://api.forecast.io/forecast/" + apiKey +
"/" + latitude + "," + longitude;
if(isNetworkAvailable()) {
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url(forecastUrl)
.build();
Call call = client.newCall(request);
call.enqueue(new Callback() {
@Override
public void onFailure(Call call, IOException e) {
}
@Override
public void onResponse(Call call, Response response) throws IOException {
try {
String jsonData = response.body().string();
Log.v(TAG, jsonData);
if (response.isSuccessful()) {
mCurrentWeather = getCurrentDetails(jsonData);
runOnUiThread(new Runnable() {
@Override
public void run() {
updateDisplay();
}
});
} else {
alertUserAboutError();
}
}
catch (IOException e) {
Log.e(TAG, "Exception caught: ", e);
}
catch (JSONException e) {
Log.e(TAG, "Exception caught: ", e);
}
}
});
}
else {
Toast.makeText(this, getString(R.string.network_unavailable_message),
Toast.LENGTH_LONG).show();
}
Log.d(TAG, "Main UI code is running");
}
private void updateDisplay() {
mTemperatureLabel.setText(mCurrentWeather.getTemperature() + "");
mTimeLabel.setText("At " + mCurrentWeather.getFormattedTime() + " it will be");
mHumidityValue.setText(mCurrentWeather.getHumidity() + "");
mPrecipValue.setText(mCurrentWeather.getPrecipChance() + "");
mSummaryLabel.setText(mCurrentWeather.getSummary());
}
private CurrentWeather getCurrentDetails(String jsonData) throws JSONException {
JSONObject forecast = new JSONObject(jsonData);
String timezone = forecast.getString("timezone");
Log.i(TAG, "From JSON: " + timezone);
JSONObject currently = forecast.getJSONObject("currently");
CurrentWeather currentWeather = new CurrentWeather();
currentWeather.setHumidity(currently.getDouble("humidity"));
currentWeather.setTime(currently.getLong("time"));
currentWeather.setIcon(currently.getString("icon"));
currentWeather.setPrecipChance(currently.getDouble("precipProbability"));
currentWeather.setSummary(currently.getString("summary"));
currentWeather.setTemperature(currently.getDouble("temperature"));
currentWeather.setTimeZone(timezone);
Log.d(TAG, currentWeather.getFormattedTime());
return new CurrentWeather();
}
private boolean isNetworkAvailable() {
ConnectivityManager manager = (ConnectivityManager)
getSystemService(Context.CONNECTIVITY_SERVICE);
NetworkInfo networkInfo = manager.getActiveNetworkInfo();
boolean isAvailable = false;
if (networkInfo != null && networkInfo.isConnected()){
isAvailable = true;
}
return isAvailable;
}
private void alertUserAboutError() {
AlertDialogFragment dialog = new AlertDialogFragment();
dialog.show(getFragmentManager(), "error_dialog");
}
}
'''
I THINK I have ButterKnife implemented properly, but maybe I'm just missing something. If you think so please let me know and I'll post my gradle file too. I know ButterKnife 8.0 is has the extra code and I've put that in and synced.
This one has been driving me nuts and and I just can't figure it out. Thanks for taking a look!
1 Answer

Seth Kroger
56,413 PointsYour ButterKnife config is fine, it would have caused a crash before that otherwise. In getCurrentDetails() you are returning a brand new and empty CurrentWeather object at the end, not the one you've filled in the data for. You should return the one you've been working on with the forecast data filled in.
Quintin Marcelino
2,693 PointsQuintin Marcelino
2,693 PointsThat was definitely it. Thanks! I was completely convinced it was ButterKnife implementation and I totally overlooked that.
Emily Conroyd
6,512 PointsEmily Conroyd
6,512 PointsThank you!!! Was returning a new object as well. Not sure when he updated that line of code in the video to return the currentWeather object.