Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial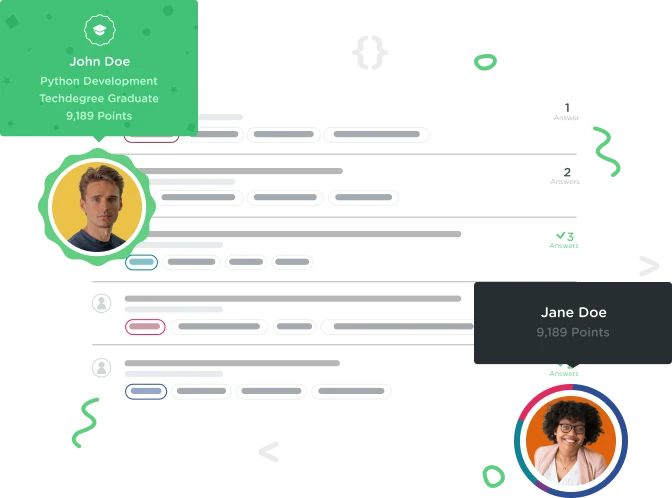
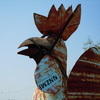
Will Anderson
28,683 Points[SOLVED] Possible version compatibility problem - NameError in my Python but not in workspaces
[RESOLUTION] I installed Python 3.6 on my Mac, then following the instructions for multiple Python installs used the command python3 in the terminal to use 3.6. The game dungeon_game.py works exactly as expected. Version compatibility issue confirmed. (Background) I have been using Python 2.7 as the development language for ROBOT Test, a web app test automation framework that only works with py 2.7. Good to know.
[ORIGINAL ENTRY] This is dungeon_game.py, finishing up the coding in the Movement section. I run Python 2.7 on my Mac using jEdit. My problem is that I get the following error on my system which does not occur in Workspaces: Welcome to the dungeon! You are currently in room (1, 4) You can move LEFT, RIGHT, UP Enter QUIT to quit
LEFT Traceback (most recent call last): File "dungeon_game.py", line 67, in <module> move = input("> ") File "<string>", line 1, in <module> NameError: name 'LEFT' is not defined Wills-Air:python willanderson$ <- That's me!
Is it a version compatibility error? Any help is greatly appreciated!
And here's the entire source code file contents: import os import random
dungeon_game.py
draw grid
pick random location for player
pick random location for the 'monster'
draw player in the grid
take input for movement of player
move player, unless invalid move (like off the grid)
check for win/loss
clear screen and redraw the grid
list of tuples as design pattern for smaller grids
CELLS = [(0,0), (1,0), (2,0), (3,0), (4,0), (0,1), (1,1), (2,1), (3,1), (4,1), (0,2), (1,2), (2,2), (3,2), (4,2), (0,3), (1,3), (2,3), (3,3), (4,3), (0,4), (1,4), (2,4), (3,4), (4,4)]
def clear_screen(): os.system('cls' if os.name == 'nt' else 'clear')
def get_locations(): return random.sample(CELLS, 3)
def move_player(player, move): x, y = player if move == "LEFT": x -= 1 if move == "RIGHT": x += 1 if move == "UP": y -= 1 if move == "DOWN": y += 1 return x, y
def get_moves(player): moves = ["LEFT", "RIGHT", "UP", "DOWN"] x, y = player if x == 0: moves.remove("LEFT") if x == 4: moves.remove("RIGHT") if y == 0: moves.remove("UP") if y == 4: moves.remove("DOWN") return moves
player, monster, door = get_locations()
while True: valid_moves = get_moves(player) clear_screen() print("Welcome to the dungeon!") print("You are currently in room {}".format(player)) print("You can move {}".format(", ".join(valid_moves))) print("Enter QUIT to quit")
move = input("> ")
move = move.upper()
if move == 'QUIT':
break
if move in valid_moves:
player = move_player(player, move)
else:
print("\n ** Walls are hard, yo! Don't run into them! **\n")
continue