Welcome to the Treehouse Community
Want to collaborate on code errors? Have bugs you need feedback on? Looking for an extra set of eyes on your latest project? Get support with fellow developers, designers, and programmers of all backgrounds and skill levels here with the Treehouse Community! While you're at it, check out some resources Treehouse students have shared here.
Looking to learn something new?
Treehouse offers a seven day free trial for new students. Get access to thousands of hours of content and join thousands of Treehouse students and alumni in the community today.
Start your free trial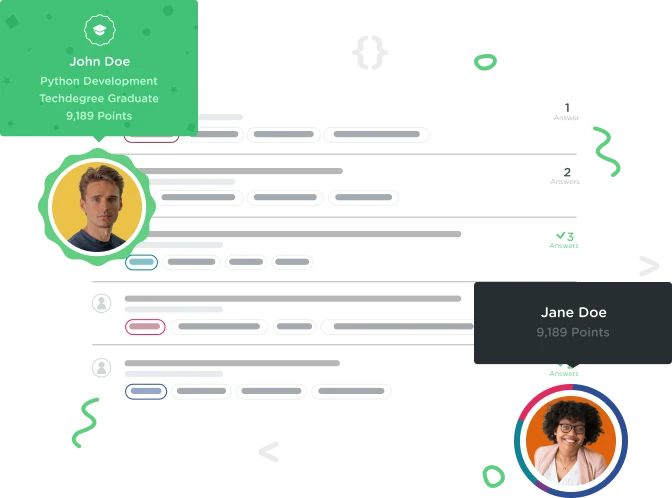
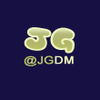
Jonathan Grieve
Treehouse Moderator 91,253 Points[SOLVED] Pressing Enter returns a TypeError
Sometimes when you come back to an error after another day you can get a fresh perspective on a problem and pick out the answer. Not today I'm afraid :)
import os
shopping_list = []
def clear_screen():
os.system("cls" if os.name == "nt" else "clear")
def show_help():
clear_screen()
print("What should we pick up at the store?")
print("""
Enter 'DONE' to stop adding items.
Enter 'HELP' for this help.
Enter 'SHOW' to see your current list.
""")
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should I add {}?\n"
"Press ENTER to add to the end of the list.\n"
"> ".format(item))
else:
position = 0
try:
position = abs(int(position))
except ValueError:
postion = None
if position is not None:
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
show_list()
def show_list():
clear_screen()
print("Here's your list:")
index = 1;
for item in shopping_list:
print("{}. {}".format(index, item))
index += 1
print("-"*10)
show_help()
while True:
new_item = input("> ")
if new_item.upper() == 'DONE' or new_item.upper() == "QUIT":
break
elif new_item.upper() == 'HELP':
show_help()
continue
elif new_item.upper() == 'SHOW':
show_list()
continue
else:
add_to_list(new_item)
show_list()
This is the script from the end of the video - the shopping list example. It almost works flawlessly, except when I press Enter the console returns the following TypeError.
Traceback (most recent call last):
File "shopping_list_3.py", line 66, in <module>
add_to_list(new_item)
File "shopping_list_3.py", line 34, in add_to_list
shopping_list.insert(position-1, item)
TypeError: unsupported operand type(s) for -: 'str' and 'int'
It's just possible I'm missing something obvious but I don't see it. I'd appreciate the pointer if possible :)
3 Answers
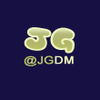
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi Dave Harker
I've just worked it out :)
In line 32 of my script I'd misspelled the position variable so it wasn't converting the type properly. Works like a dream now.
def add_to_list(item):
show_list()
if len(shopping_list):
position = input("Where should I add {}?\n"
"Press ENTER to add to the end of the list.\n"
"> ".format(item))
else:
position = 0
try:
position = abs(int(position))
except ValueError:
position = None
if position :
shopping_list.insert(position-1, item)
else:
shopping_list.append(new_item)
show_list()
Thanks for trying!!
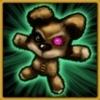
Dave Harker
Courses Plus Student 15,510 PointsHi Jonathan,
It's now 2:34am ... I could be wrong but ... your position variable is a string, and you're using that to define an index in a list.
perhaps change
if position is not None:
shopping_list.insert(position-1, item)
to
if position.isnumeric():
shopping_list.insert(position-1, item)
OK, eyes slowly closing on their own :P
Dave.
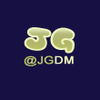
Jonathan Grieve
Treehouse Moderator 91,253 PointsHi Dave,
Changing the code as you suggest gives me an AttributeError the moment I submit my first item, so I don't think the answer lies there. :)
Traceback (most recent call last):
File "shopping_list_4.py", line 79, in <module>
add_to_list(new_item)
File "shopping_list_4.py", line 34, in add_to_list
if position.isnumeric():
AttributeError: 'int' object has no attribute 'isnumeric'
I just wonder if there's been a bug introduced to the code since the course was first recorded. Kenneth uses is not None
in his script which doesn't cause him an issue.
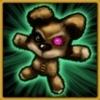
Dave Harker
Courses Plus Student 15,510 PointsInteresting ...
I popped in a little code snip into an online python repl and it ran ok, but then again it was 2:30(ish) AM :P Just woke up and off to work now but I'll take another peek when I get back if we've not had any traction on the thread by then. I should also watch the related video ... might help grin
Until then, have a good day/night (guess it depends on where you are)
Dave.

Nigel Ngai
3,056 PointsI had the exact problem, I misspelled position too. Thanks Jonathan for helping me figure out what was wrong with my code (X_X) !! <3
Dave Harker
Courses Plus Student 15,510 PointsDave Harker
Courses Plus Student 15,510 PointsHi Jonathan Grieve,
Glad it's all working now, and thank you for letting me know the error.
While I'd planned on looking into that a little later tonight ... wanted to get some study done first :) ... it had been on my mind a bit. I couldn't figure out why I could get my snippet working that I typed in but yours didn't work when I pasted that portion into the REPL. Now I know! So thank you again.
Best of luck and happy coding,
Dave
Jonathan Grieve
Treehouse Moderator 91,253 PointsJonathan Grieve
Treehouse Moderator 91,253 PointsI think the interpreters read Python a little differently to Workspaces or on my command line. When I was trying an online REPL I had to do a little digging to find a Python v3 one.
But eventually what I wasn't finding for a couple of days suddenly stuck out like a sore thumb.... which is sometimes how it goes lol. :D